mirror of
https://github.com/nushell/nushell.git
synced 2024-11-22 00:13:21 +01:00
standard library: add log commands (#8448)
# Description ```nushell log critical "this is a critical message" log error "this is an error message" log warning "this is a warning message" log info "this is an info message" log debug "this is a debug message" ``` 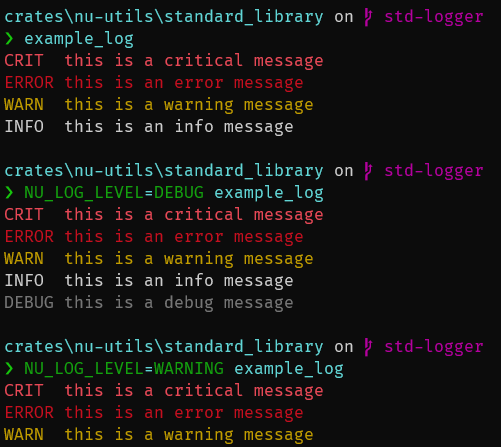 # Tests + Formatting Tests are written. To run automatically, #8443 needs to be merged before or after this PR. --------- Co-authored-by: Mate Farkas <Mate.Farkas@oneidentity.com>
This commit is contained in:
parent
c66bd5e809
commit
10a42de64f
@ -60,13 +60,13 @@ use /path/to/standard_library/std.nu
|
||||
### :test_tube: run the tests
|
||||
the following call should return no errors
|
||||
```bash
|
||||
nu /path/to/standard_library/tests.nu
|
||||
NU_LOG_LEVEL=DEBUG nu /path/to/standard_library/tests.nu
|
||||
```
|
||||
|
||||
> #### :mag: a concrete example
|
||||
> with `STD_LIB` defined as in the example above
|
||||
> ```bash
|
||||
> nu ($env.STD_LIB | path join "tests.nu")
|
||||
> NU_LOG_LEVEL=DEBUG nu ($env.STD_LIB | path join "tests.nu")
|
||||
> ```
|
||||
|
||||
[REPL]: https://en.wikipedia.org/wiki/Read%E2%80%93eval%E2%80%93print_loop
|
||||
|
@ -236,3 +236,63 @@ def-env _fetch [
|
||||
|
||||
cd ($env.DIRS_LIST | get $pos )
|
||||
}
|
||||
|
||||
def CRITICAL_LEVEL [] { 50 }
|
||||
def ERROR_LEVEL [] { 40 }
|
||||
def WARNING_LEVEL [] { 30 }
|
||||
def INFO_LEVEL [] { 20 }
|
||||
def DEBUG_LEVEL [] { 10 }
|
||||
|
||||
def parse-string-level [level: string] {
|
||||
(
|
||||
if $level == "CRITICAL" { (CRITICAL_LEVEL)}
|
||||
else if $level == "CRIT" { (CRITICAL_LEVEL)}
|
||||
else if $level == "ERROR" { (ERROR_LEVEL) }
|
||||
else if $level == "WARNING" { (WARNING_LEVEL) }
|
||||
else if $level == "WARN" { (WARNING_LEVEL) }
|
||||
else if $level == "INFO" { (INFO_LEVEL) }
|
||||
else if $level == "DEBUG" { (DEBUG_LEVEL) }
|
||||
else { (INFO_LEVEL) }
|
||||
)
|
||||
}
|
||||
|
||||
def current-log-level [] {
|
||||
let env_level = ($env | get -i NU_LOG_LEVEL | default (INFO_LEVEL))
|
||||
|
||||
try {
|
||||
($env_level | into int)
|
||||
} catch {
|
||||
parse-string-level $env_level
|
||||
}
|
||||
}
|
||||
|
||||
# Log critical message
|
||||
export def "log critical" [message: string] {
|
||||
if (current-log-level) > (CRITICAL_LEVEL) { return }
|
||||
|
||||
print --stderr $"(ansi red_bold)CRIT ($message)(ansi reset)"
|
||||
}
|
||||
# Log error message
|
||||
export def "log error" [message: string] {
|
||||
if (current-log-level) > (ERROR_LEVEL) { return }
|
||||
|
||||
print --stderr $"(ansi red)ERROR ($message)(ansi reset)"
|
||||
}
|
||||
# Log warning message
|
||||
export def "log warning" [message: string] {
|
||||
if (current-log-level) > (WARNING_LEVEL) { return }
|
||||
|
||||
print --stderr $"(ansi yellow)WARN ($message)(ansi reset)"
|
||||
}
|
||||
# Log info message
|
||||
export def "log info" [message: string] {
|
||||
if (current-log-level) > (INFO_LEVEL) { return }
|
||||
|
||||
print --stderr $"(ansi white)INFO ($message)(ansi reset)"
|
||||
}
|
||||
# Log debug message
|
||||
export def "log debug" [message: string] {
|
||||
if (current-log-level) > (DEBUG_LEVEL) { return }
|
||||
|
||||
print --stderr $"(ansi default_dimmed)DEBUG ($message)(ansi reset)"
|
||||
}
|
||||
|
39
crates/nu-utils/standard_library/test_logger.nu
Normal file
39
crates/nu-utils/standard_library/test_logger.nu
Normal file
@ -0,0 +1,39 @@
|
||||
use std.nu *
|
||||
|
||||
def run [system_level, message_level] {
|
||||
cd $env.FILE_PWD
|
||||
do {
|
||||
nu -c $'use std.nu; NU_LOG_LEVEL=($system_level) std log ($message_level) "test message"'
|
||||
} | complete | get -i stderr
|
||||
}
|
||||
def "assert no message" [system_level, message_level] {
|
||||
let output = (run $system_level $message_level)
|
||||
assert eq $output ""
|
||||
}
|
||||
|
||||
def "assert message" [system_level, message_level, message_level_str] {
|
||||
let output = (run $system_level $message_level)
|
||||
assert ($output | str contains $message_level_str)
|
||||
assert ($output | str contains "test message")
|
||||
}
|
||||
|
||||
export def test_critical [] {
|
||||
assert no message 99 critical
|
||||
assert message CRITICAL critical CRIT
|
||||
}
|
||||
export def test_error [] {
|
||||
assert no message CRITICAL error
|
||||
assert message ERROR error ERROR
|
||||
}
|
||||
export def test_warning [] {
|
||||
assert no message ERROR warning
|
||||
assert message WARNING warning WARN
|
||||
}
|
||||
export def test_info [] {
|
||||
assert no message WARNING info
|
||||
assert message INFO info INFO
|
||||
}
|
||||
export def test_debug [] {
|
||||
assert no message INFO debug
|
||||
assert message DEBUG debug DEBUG
|
||||
}
|
@ -1,10 +1,12 @@
|
||||
use std.nu *
|
||||
|
||||
def main [] {
|
||||
for test_file in (ls ($env.FILE_PWD | path join "test_*.nu") -f | get name) {
|
||||
let $module_name = ($test_file | path parse).stem
|
||||
|
||||
echo $"(ansi default)INFO Run tests in ($module_name)(ansi reset)"
|
||||
log info $"Run tests in ($module_name) module"
|
||||
let tests = (
|
||||
nu -c $'use ($test_file) *; $nu.scope.commands | to nuon'
|
||||
nu -c $'use ($test_file) *; $nu.scope.commands | select name module_name | to nuon'
|
||||
| from nuon
|
||||
| where module_name == $module_name
|
||||
| where ($it.name | str starts-with "test_")
|
||||
@ -12,7 +14,7 @@ def main [] {
|
||||
)
|
||||
|
||||
for test_case in $tests {
|
||||
echo $"(ansi default_dimmed)DEBUG Run test ($module_name)/($test_case)(ansi reset)"
|
||||
log debug $"Run test ($module_name) ($test_case)"
|
||||
nu -c $'use ($test_file) ($test_case); ($test_case)'
|
||||
}
|
||||
}
|
||||
|
Loading…
Reference in New Issue
Block a user