Closes#7572 by adding a cache for compiled regexes of type
`Arc<Mutex<LruCache<String, Regex>>>` to `EngineState` .
The cache is limited to 100 entries (limit chosen arbitrarily) and
evicts least-recently-used items first.
This PR makes a noticeable difference when using regexes for
`color_config`, e.g.:
```bash
#first set string formatting in config.nu like:
string: { if $in =~ '^#\w{6}$' { $in } else { 'white' } }`
# then try displaying and exploring a table with many strings
# this is instant after the PR, but takes hundreds of milliseconds before
['#ff0033', '#0025ee', '#0087aa', 'string', '#4101ff', '#ff0033', '#0025ee', '#0087aa', 'string', '#6103ff', '#ff0033', '#0025ee', '#0087aa', 'string', '#6103ff', '#ff0033', '#0025ee', '#0087aa', 'string', '#6103ff', '#ff0033', '#0025ee', '#0087aa', 'string', '#6103ff','#ff0033', '#0025ee', '#0087aa', 'string', '#6103ff','#ff0033', '#0025ee', '#0087aa', 'string', '#6103ff','#ff0033', '#0025ee', '#0087aa', 'string', '#6103ff','#ff0033', '#0025ee', '#0087aa', 'string', '#6103ff','#ff0033', '#0025ee', '#0087aa', 'string', '#6103ff']
```
## New dependency (`lru`)
This uses [the popular `lru` crate](https://lib.rs/crates/lru). The new
dependency adds 19.8KB to a Linux release build of Nushell. I think this
is OK, especially since the crate can be useful elsewhere in Nu.
# Description
_(Thank you for improving Nushell. Please, check our [contributing
guide](../CONTRIBUTING.md) and talk to the core team before making major
changes.)_
_(Description of your pull request goes here. **Provide examples and/or
screenshots** if your changes affect the user experience.)_
# User-Facing Changes
_(List of all changes that impact the user experience here. This helps
us keep track of breaking changes.)_
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
General tree shaking through `cargo +nightly udeps` and moving mentions
of `nu-test-support` to the dev deps.
Also since #7488 no separate import of `nu-path` necessary
cc @webbedspace
ref #7339 - This PR updates explore to take some of the colors from
nushell, namely the line colors and the ls_colors.
note: Not sure why this regression appeared maybe it's a feature or it's
no longer supposed to be supported?
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
# Description
Closes#6909. You can now add closures to your `color_config` themes.
Whenever a value would be printed with `table`, the closure is run with
the value piped-in. The closure must return either a {fg,bg,attr} record
or a color name (`'light_red'` etc.). This returned style is used to
colour the value.
This is entirely backwards-compatible with existing config.nu files.
Example code excerpt:
```
let my_theme = {
header: green_bold
bool: { if $in { 'light_cyan' } else { 'light_red' } }
int: purple_bold
filesize: { |e| if $e == 0b { 'gray' } else if $e < 1mb { 'purple_bold' } else { 'cyan_bold' } }
duration: purple_bold
date: { (date now) - $in | if $in > 1wk { 'cyan_bold' } else if $in > 1day { 'green_bold' } else { 'yellow_bold' } }
range: yellow_bold
string: { if $in =~ '^#\w{6}$' { $in } else { 'white' } }
nothing: white
```
Example output with this in effect:
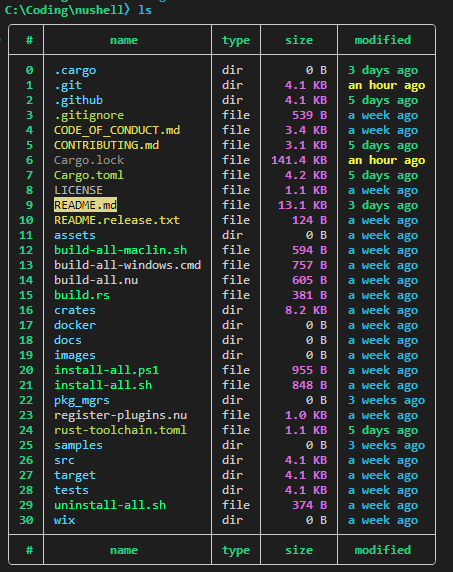
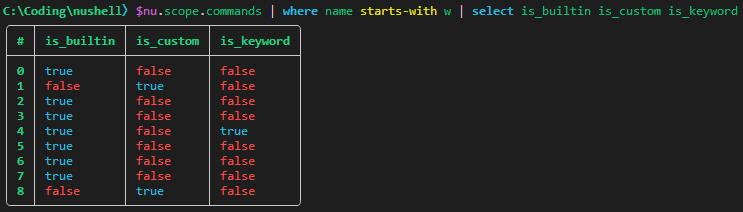
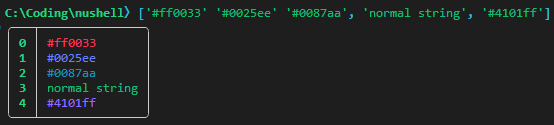
Slightly important notes:
* Some color_config names, namely "separator", "empty" and "hints", pipe
in `null` instead of a value.
* Currently, doing anything non-trivial inside a closure has an
understandably big perf hit. I currently do not actually recommend
something like `string: { if $in =~ '^#\w{6}$' { $in } else { 'white' }
}` for serious work, mainly because of the abundance of string-type data
in the world. Nevertheless, lesser-used types like "date" and "duration"
work well with this.
* I had to do some reorganisation in order to make it possible to call
`eval_block()` that late in table rendering. I invented a new struct
called "StyleComputer" which holds the engine_state and stack of the
initial `table` command (implicit or explicit).
* StyleComputer has a `compute()` method which takes a color_config name
and a nu value, and always returns the correct Style, so you don't have
to worry about A) the color_config value was set at all, B) whether it
was set to a closure or not, or C) which default style to use in those
cases.
* Currently, errors encountered during execution of the closures are
thrown in the garbage. Any other ideas are welcome. (Nonetheless, errors
result in a huge perf hit when they are encountered. I think what should
be done is to assume something terrible happened to the user's config
and invalidate the StyleComputer for that `table` run, thus causing
subsequent output to just be Style::default().)
* More thorough tests are forthcoming - ran into some difficulty using
`nu!` to take an alternative config, and for some reason `let-env config
=` statements don't seem to work inside `nu!` pipelines(???)
* The default config.nu has not been updated to make use of this yet. Do
tell if you think I should incorporate that into this.
# User-Facing Changes
See above.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace --features=extra -- -D warnings -D
clippy::unwrap_used -A clippy::needless_collect` to check that you're
using the standard code style
- `cargo test --workspace --features=extra` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
## Fix `nu-path` usage in `nu!` testing macro
The `nu-path` crate needs to be properly re-exported so the generated
code is valid if `nu-path` is not present among the dependencies of the
using crate.
Usage of crates in `macro_rules!` macros has to follow the
`$crate::symbol_in_crate` path pattern (With an absolute path-spec also
for macros defined in submodules)
## Move `nu-test-support` to devdeps in `nu-protocol`
Also remove the now unnecessary direct dependency on `nu-path`.
`nu!` macro had to be changed to make it a proper transitive dependency.
# Description
Closes#7059. Rather than generate a new Record each time $env.config is
accessed (as described in that issue), instead `$env.config = ` now A)
parses the input record, then B) un-parses it into a clean Record with
only the valid values, and stores that as an env-var. The reasoning for
this is that I believe `config_to_nu_record()` (the method that performs
step B) will be useful in later PRs. (See below)
As a result, this also "fixes" the following "bug":
```
〉$env.config = 'butts'
$env.config is not a record
〉$env.config
butts
```
~~Instead, `$env.config = 'butts'` now turns `$env.config` into the
default (not the default config.nu, but `Config::default()`, which
notably has empty keybindings, color_config, menus and hooks vecs).~~
This doesn't attempt to fix#7110. cc @Kangaxx-0
# Example of new behaviour
OLD:
```
〉$env.config = ($env.config | merge { foo: 1 })
$env.config.foo is an unknown config setting
〉$env.config.foo
1
```
NEW:
```
〉$env.config = ($env.config | merge { foo: 1 })
Error:
× Config record contains invalid values or unknown settings
Error:
× Error while applying config changes
╭─[entry #1:1:1]
1 │ $env.config = ($env.config | merge { foo: 1 })
· ┬
· ╰── $env.config.foo is an unknown config setting
╰────
help: This value has been removed from your $env.config record.
〉$env.config.foo
Error: nu:🐚:column_not_found (link)
× Cannot find column
╭─[entry #1:1:1]
1 │ $env.config = ($env.config | merge { foo: 1 })
· ──┬──
· ╰── value originates here
╰────
╭─[entry #2:1:1]
1 │ $env.config.foo
· ─┬─
· ╰── cannot find column 'foo'
╰────
```
# Example of new errors
OLD:
```
$env.config.cd.baz is an unknown config setting
$env.config.foo is an unknown config setting
$env.config.bar is an unknown config setting
$env.config.table.qux is an unknown config setting
$env.config.history.qux is an unknown config setting
```
NEW:
```
Error:
× Config record contains invalid values or unknown settings
Error:
× Error while applying config changes
╭─[C:\Users\Leon\AppData\Roaming\nushell\config.nu:267:1]
267 │ abbreviations: true # allows `cd s/o/f` to expand to `cd some/other/folder`
268 │ baz: 3,
· ┬
· ╰── $env.config.cd.baz is an unknown config setting
269 │ }
╰────
help: This value has been removed from your $env.config record.
Error:
× Error while applying config changes
╭─[C:\Users\Leon\AppData\Roaming\nushell\config.nu:269:1]
269 │ }
270 │ foo: 1,
· ┬
· ╰── $env.config.foo is an unknown config setting
271 │ bar: 2,
╰────
help: This value has been removed from your $env.config record.
Error:
× Error while applying config changes
╭─[C:\Users\Leon\AppData\Roaming\nushell\config.nu:270:1]
270 │ foo: 1,
271 │ bar: 2,
· ┬
· ╰── $env.config.bar is an unknown config setting
╰────
help: This value has been removed from your $env.config record.
Error:
× Error while applying config changes
╭─[C:\Users\Leon\AppData\Roaming\nushell\config.nu:279:1]
279 │ }
280 │ qux: 4,
· ┬
· ╰── $env.config.table.qux is an unknown config setting
281 │ }
╰────
help: This value has been removed from your $env.config record.
Error:
× Error while applying config changes
╭─[C:\Users\Leon\AppData\Roaming\nushell\config.nu:285:1]
285 │ file_format: "plaintext" # "sqlite" or "plaintext"
286 │ qux: 2
· ┬
· ╰── $env.config.history.qux is an unknown config setting
287 │ }
╰────
help: This value has been removed from your $env.config record.
```
# User-Facing Changes
See above.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
After the 0.72.1 hotfix, let's bump our dev builds to 0.72.2.
# User-Facing Changes
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
This change makes SQLite queries (`open foo.db`, `open foo.db | query db
"select ..."`) cancellable using `ctrl+c`. Previously they were not
cancellable, which made it unpleasant to accidentally open a very large
database or run an unexpectedly slow query!
UX-wise there's not too much to show:
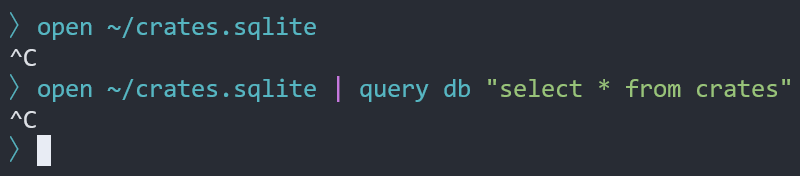
## Notes
I was hoping to make SQLite queries streamable as part of this work, but
I ran into 2 problems:
1. `rusqlite` lifetimes are nightmarishly complex and they make it hard
to create a `ListStream` iterator
2. The functions on Nu's `CustomValue` trait return `Value` not
`PipelineData` and so `CustomValue` implementations can't stream data
AFAICT.
# Description
Just some minor updates to xml deps
# User-Facing Changes
_(List of all changes that impact the user experience here. This helps
us keep track of breaking changes.)_
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
Warning: the `nu-json` crate seems to be undertested as the main test
code is commented out. Thus the unused dependencies there were just
commented out
# Description
Just bumping to the next dev version. Submitting as a draft until we're
ready.
# User-Facing Changes
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
This upgrades the `windows` and `trash` crates so we are only compiling
1 version of the `windows` crate ([I recently upgraded the version used
in `trash`](https://github.com/Byron/trash-rs/pull/58)).
I was hoping that this would lead to some decent compile time
improvements, but unfortunately it did not. Compiling 1 version of
`windows` with all the features unified is about as slow as compiling 2
versions with distinct feature sets. Still, might as well upgrade.
# Description
_(Thank you for improving Nushell. Please, check our [contributing
guide](../CONTRIBUTING.md) and talk to the core team before making major
changes.)_
_(Description of your pull request goes here. **Provide examples and/or
screenshots** if your changes affect the user experience.)_
# User-Facing Changes
_(List of all changes that impact the user experience here. This helps
us keep track of breaking changes.)_
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
This fixes the compilation problems with `aarch64-apple-darwin` on rust
1.64 as well as the `zstd` problems. We recently found out that `zstd`
pinned to 1.65.
# User-Facing Changes
N/A
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
chrono version update
# Description
upgrade chrono to 0.4.23
# Major Changes
If you're considering making any major change to nushell, before
starting work on it, seek feedback from regular contributors and get
approval for the idea from the core team either on
[Discord](https://discordapp.com/invite/NtAbbGn) or [GitHub
issue](https://github.com/nushell/nushell/issues/new/choose).
Making sure we're all on board with the change saves everybody's time.
Thanks!
# Tests + Formatting
Make sure you've done the following, if applicable:
- Add tests that cover your changes (either in the command examples, the
crate/tests folder, or in the /tests folder)
- Try to think about corner cases and various ways how your changes
could break. Cover those in the tests
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace --features=extra -- -D warnings -D
clippy::unwrap_used -A clippy::needless_collect` to check that you're
using the standard code style
- `cargo test --workspace --features=extra` to check that all tests pass
# After Submitting
* Help us keep the docs up to date: If your PR affects the user
experience of Nushell (adding/removing a command, changing an
input/output type, etc.), make sure the changes are reflected in the
documentation (https://github.com/nushell/nushell.github.io) after the
PR is merged.
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
# Description
The `zstd` team released a version that breaks dataframe compilation.
This change pins to `zstd-sys = "=2.0.1+zstd.1.5.2"` in order to prevent
the required `+nightly` build flag.
_(Thank you for improving Nushell. Please, check our [contributing
guide](../CONTRIBUTING.md) and talk to the core team before making major
changes.)_
_(Description of your pull request goes here. **Provide examples and/or
screenshots** if your changes affect the user experience.)_
# User-Facing Changes
_(List of all changes that impact the user experience here. This helps
us keep track of breaking changes.)_
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
* removes unused features.
* Adds back multithreading feature to sysinfo.
* Adds back alloc for percent-encoding
* Adds updated lock file.
* Missed one sysinfo.
* `indexmap` just defaults
* Revert `miette``default-features=false`
Co-authored-by: Stefan Holderbach <sholderbach@users.noreply.github.com>
Co-authored-by: JT <547158+jntrnr@users.noreply.github.com>
* Add failing test that list of ints and floats is List<Number>
* Start defining subtype relation
* Make it possible to declare input and output types for commands
- Enforce them in tests
* Declare input and output types of commands
* Add formatted signatures to `help commands` table
* Revert SyntaxShape::Table -> Type::Table change
* Revert unnecessary derive(Hash) on SyntaxShape
Co-authored-by: JT <547158+jntrnr@users.noreply.github.com>
Allows use of slightly optimized variants that check if they have to use
the heavier vte parser. Tries to avoid unnnecessary allocations. Initial
performance characteristics proven out in #4378.
Also reduces boilerplate with right-ward drift.
* fix: fixcd
try to fix
Log: try to fix the bug with can enter a permisson error fold
* change wording
* fat
* fmt
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
* Bump nushell-sytem dep to ntapi 0.4
0.3.7 trigger a warning about code being incompatible
with future rust versions. This is resolved in 0.4
https://github.com/MSxDOS/ntapi/issues/11
* Upgrade Cargo.lock for ntapi 0.4
* Remove unused dependencies
Inspired by #6938 ran `cargo +nightly udeps --features extra`.
Removes 2 crates and should remove an unnecessary intra-workspace
dependency which might open up further opportunities for compilation.
* Make windows-only dependency conditional in toml
`omnipath` is only used on Windows and already behind a `#[cfg]` block
in the code. Made the dependency in `Cargo.toml` conditional as well.
* Make `nu-pretty-hex` example a proper example
This allows making `rand` a dev-dependency in this crate.
Avoids compiling the crate twice due to incompatible versions from
dependencies. This avoids binary bloat before linking as well.
Narrow our feature selection to the used modules/functions to save
compile time. On my machine reduces `nix` crate compile time from
around 9 secs to 0.9 secs.
Disable backtrace on miette
- gimli crate requires several seconds
Disable diagnostics on wax
- depends on an outdated miette version
Builds fine, no observable loss in diagnostics quality of life
Removes 10 crates that have to be compiled.
* Add support to render right prompt on last line of the prompt
* reset reedline to main branch
* update reedline to fix right prompt to be rendered correctly
* reset reedline to main branch again
* Make Tab insert (partial) completion instead of select next menu item
* Use reedline feature branch
Co-authored-by: JT <547158+jntrnr@users.noreply.github.com>
* Reorder conditional deps for readability
* Pull reedline from the most recent main branch
* Map 'Submit' and 'SubmitOrNewline' events
Introduced by nushell/reedline#490
* add a new command to query the registry on windows
* cross platform tweaks
* return nushell datatype
* change visibility of exec and registry commands
* Revert "Revert "Try again: in unix like system, set foreground process while running external command (#6273)" (#6542)"
This reverts commit 2bb367f570.
* Make foreground job control hopefully work correctly
These changes are mostly inspired by the glibc manual.
* Fix typo in external command description
* Only restore tty control to shell when no fg procs are left; reuse pgrp
* Rework terminal acquirement code to be like fish
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
* Add "space" key to bind in vi normal mode
Implements #6586
No special logic to prevent you from binding it in other modes!
Needs a separate change to reedline to make it available in the default
listing of `keybindings list`.
* Update reedline to report the available `space`
Pulls in nushell/reedline#486
This is a de-facto standard supported by many terminals, originally
added to macOS Terminal.app, now also supported by VTE (GNOME),
Konsole (KDE), WezTerm, and more.
* Add support for Arrow IPC file format
Add support for Arrow IPC file format to dataframes commands. Support
opening of Arrow IPC-format files with extension '.arrow' or '.ipc' in
the open-df command. Add a 'to arrow' command to write a dataframe to
Arrow IPC format.
* Add unit test for open-df on Arrow
* Add -t flag to open-df command
Add a `--type`/`-t` flag to the `open-df` command, to explicitly specify
the type of file being used. Allowed values are the same at the set of
allowed file extensions.
* Add a 'commandline' command for manipulating the current buffer
from `executehostcommand` keybindings. Inspired by fish:
https://fishshell.com/docs/current/cmds/commandline.html
* Update to development reedline
Includes nushell/reedline#472
Co-authored-by: sholderbach <sholderbach@users.noreply.github.com>
* Terminate REPL if not connected to tty input
If the standard input stream is not a TTY abort the REPL execution.
Solves a problem as the current REPL tries to be IO fault tolerant and
would indefinetely fail when crossterm tries to handle the STDIN.
Fixesnushell/nushell#6452
* Improve the error message
* Avoid update_last_command_context "No command run" error
When using `executehostcommand` bindings without having run actual user input commands yet,
update_last_command_context is guaranteed to fail. A function has been added to reedline
that allows checking for this case.
* Update to most recent reedline
Includes bugfixes around the (SQlite) history
Co-authored-by: sholderbach <sholderbach@users.noreply.github.com>
* remove unnecessary FlatShape
* add proptest
* remove files that belonged in another PR
* more tests, more chars
* add exception for parser error unrelated ot PR
* Fix ps command CPU usage on Apple Silicon M1 macs. #4142
The cpu user and system times returned my libproc are not in
nanoseconds; they are in mach ticks units. This is not documented very
well. The convert from mach ticks to ns, the kernel provides a timebase
info function and datatype:
https://developer.apple.com/documentation/driverkit/3433733-mach_timebase_info
The commit makes the PS command work for me.
* Cargo fmt of previous commit.
* Clippy format suggestion
Co-authored-by: Ondrej Baudys <ondrej.baudys@nextgen.net>
* Test commands for proper names and search terms
Assert that the `Command.name()` is equal to `Signature.name`
Check that search terms are not just substrings of the command name as
they would not help finding the command.
* Clean up search terms
Remove redundant terms that just replicate the command name.
Try to eliminate substring between search terms, clean up where
necessary.
* Revert "Fix intermittent test crash (#6268)"
This reverts commit 555d9ee763.
* make a working version again
* try second impl
* add
* fmt
* check stdin is atty before acquire stdin
* add libc
* clean comment
* fix typo
* Update after Reedline API update
* Remove references to deleted `ReedlineEvent::ActionHandler`
* Update `DescriptionMenu` implementation for the new `Menu` trait
API changes that work on `Editor` rather than `LineBuffer` objects
* Update reedline
Includes nushell/reedline#460
Co-authored-by: Ben Parks <bnprks+git@gmail.com>
* Add decimals to int when using `into string --decimals`
* Add tests for `into string` when converting int with `--decimals`
* Apply formatting
* Merge `into_str` test files
* Comment out unused code and add TODOs
* Use decimal separator depending on system locale
* Add test helper to run closure in different locale
* Add tests for int-to-string conversion using different locales
* Add utils function to get system locale
* Add panic message when locking mutex fails
* Catch and resume panic later to prevent Mutex poisoning when test fails
* Move test to `nu-test-support` to keep `nu-utils` free of `nu-*` dependencies
See https://github.com/nushell/nushell/pull/6085#issuecomment-1193131694
* Rename test support fn `with_fake_locale` to `with_locale_override`
* Move `get_system_locale()` to `locale` module
* Allow overriding locale with special env variable (when not in release)
* Use special env var to override locale during testing
* Allow callback to return a value in `with_locale_override()`
* Allow multiple options in `nu!` macro
* Allow to set locale as `nu!` macro option
* Use new `locale` option of `nu!` macro instead of `with_locale_override`
Using the `locale` options does not lock the `LOCALE_OVERRIDE_MUTEX`
mutex in `nu-test-support::locale_override` but instead calls the `nu`
command directly with the `NU_LOCALE_OVERRIDE` environment variable.
This allows for parallel test excecution.
* Fix: Add option identifier for `cwd` in usage of `nu!` macro
* Rely on `Display` trait for formatting `nu!` macro command
- Removed the `DisplayPath` trait
- Implement `Display` for `AbsolutePath`, `RelativePath` and
`AbsoluteFile`
* Default to locale `en_US.UTF-8` for tests when using `nu!` macro
* Add doc comment to `nu!` macro
* Format code using `cargo fmt --all`
* Pass function directly instead of wrapping the call in a closure
https://rust-lang.github.io/rust-clippy/master/index.html#redundant_closure
* Pass function to `or_else()` instead of calling it inside `or()`
https://rust-lang.github.io/rust-clippy/master/index.html#or_fun_call
* Fix: Add option identifier for `cwd` in usage of `nu!` macro
* when spawned process during register plugin, pass env to child process
* tweak comment
* tweak comment
* remove trailing whitespace
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
`rstest = 0.12` added support for asynchronous timeouts during testing
thus requiring a larger set of dependencies. Since `rstest = 0.14` this
can be disabled if not used.
Should keep build times for local or CI tests in check.
* add a new welcome banner to nushell
* remove top line
* tweaked colors and wording
* changed to dimmed white
* removed a comment
* make config nu stand out a little
* fix type-o
* Skeleton implementation
Lots and lots of TODOs
* Bootstrap simple CustomValue plugin support test
* Create nu_plugin_custom_value
* Skeleton for nu_plugin_custom_values
* Return a custom value from plugin
* Encode CustomValues from plugin calls as PluginResponse::PluginData
* Add new PluginCall variant CollapseCustomValue
* Handle CollapseCustomValue plugin calls
* Add CallInput::Data variant to CallInfo inputs
* Handle CallInfo with CallInput::Data plugin calls
* Send CallInput::Data if Value is PluginCustomValue from plugin calls
* Remove unnecessary boxing of plugins CallInfo
* Add fields needed to collapse PluginCustomValue to it
* Document PluginCustomValue and its purpose
* Impl collapsing using plugin calls in PluginCustomValue::to_base_value
* Implement proper typetag based deserialization for CoolCustomValue
* Test demonstrating that passing back a custom value to plugin works
* Added a failing test for describing plugin CustomValues
* Support describe for PluginCustomValues
- Add name to PluginResponse::PluginData
- Also turn it into a struct for clarity
- Add name to PluginCustomValue
- Return name field from PluginCustomValue
* Demonstrate that plugins can create and handle multiple CustomValues
* Add bincode to nu-plugin dependencies
This is for demonstration purposes, any schemaless binary seralization
format will work. I picked bincode since it's the most popular for Rust
but there are defintely better options out there for this usecase
* serde_json::Value -> Vec<u8>
* Update capnp schema for new CallInfo.input field
* Move call_input capnp serialization and deserialization into new file
* Deserialize Value's span from Value itself instead of passing call.head
I am not sure if this was correct and I am breaking it or if it was a
bug, I don't fully understand how nu creates and uses Spans. What should
reuse spans and what should recreate new ones?
But yeah it felt weird that the Value's Span was being ignored since in
the json serializer just uses the Value's Span
* Add call_info value round trip test
* Add capnp CallInput::Data serialization and deserialization support
* Add CallInfo::CollapseCustomValue to capnp schema
* Add capnp PluginCall::CollapseCustomValue serialization and deserialization support
* Add PluginResponse::PluginData to capnp schema
* Add capnp PluginResponse::PluginData serialization and deserialization support
* Switch plugins::custom_values tests to capnp
Both json and capnp would work now! Sadly I can't choose both at the
same time :(
* Add missing JsonSerializer round trip tests
* Handle plugin returning PluginData as a response to CollapseCustomValue
* Refactor plugin calling into a reusable function
Many less levels of indentation now!
* Export PluginData from nu_plugin
So plugins can create their very own serve_plugin with whatever
CustomValue behavior they may desire
* Error if CustomValue cannot be handled by Plugin
* nu-table: Refactoring
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
* nu-table: consider space for single `...` column not enough space
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
* nu-table: Fix header style
It did appeared again after my small change...
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
* nu-table: Add a empty header style test
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
* Return error when external command core dumped
Fixes#5903
Signed-off-by: nibon7 <nibon7@163.com>
* Use signal-hook to get signal name
Signed-off-by: nibon7 <nibon7@163.com>
* Fix comment
Signed-off-by: nibon7 <nibon7@163.com>
* feat: deprecate `hash base64` command
* feat: extend `decode` and `encode` command families
This commit
- Adds `encode` command family
- Backports `hash base64` features to `encode base64` and `decode base64` subcommands.
- Refactors code a bit and extends tests for encodings
- `decode base64` returns a binary `Value` (that may be decoded into a string using `decode` command)
* feat: add `--binary(-b)` flag to `decode base64`
Default output type is now string, but binary can be requested using this new flag.
This PR adds support for an SQLite history via nushell/reedline#401
The SQLite history is enabled by setting history_file_format: "sqlite" in config.nu.
* somewhat working sqlite history
* Hook up history command
* Fix error in SQlitebacked with empty lines
When entering an empty line there previously was the "No command run"
error with `SqliteBackedHistory` during addition of the metadata
May be considered a temporary fix
Co-authored-by: sholderbach <sholderbach@users.noreply.github.com>
* Fix `ls` for Windows system files
* Fix non-Windows builds
* Make Clippy happy on non-Windows platforms
* Fix new test on GitHub runners
* Move ls Windows code into its own module
Now more bindings are shared between vi-mode and emacs mode.
E.g. Ctrl-D, Ctrl-C, Ctrl-L, Ctrl-O will work in all modes.
Also arrow navigation extra functions will behave consistent.
Release notes: https://github.com/nushell/reedline/releases/tag/v0.6.0
This release contains several bug fixes and improvements to the vi-emulation and documentation.
- Improvements to the vi-style keybindings (@sadmac7000):
- `w` now correctly moves to the beginning of the word.
- `e` to move to the end of the word.
- Bugfixes:
- Support terminal emulators that erroneously report a size of 0x0 by assuming a default size to avoid panics and draw nevertheless (@DhruvDh)
- Fix `ListMenu` layout calculations. Avoids scrolling bug when wrapping occurs due to the line numbering (@ahkrr)
- Avoid allocating to the total history capacity which can cause the application to go out of memory (@sholderbach)
- Documentation improvements including addition of documentation intended for reedline developers (@petrisch, @sholderbach)
Fixes#5593 (OOM introduced with #5587 when no config was present and an attempt was
made to allocate all memory in advance)
Includes also other changes to reedline:
- Vi word definition fixed and `w` and `e` work as expected
* lazyframe definition
* expressions and lazy frames
* new alias expression
* more expression commands
* updated to polars main
* more expressions and groupby
* more expressions, fetch and sort-by
* csv reader
* removed open csv
* unique function
* joining functions
* join lazy frames commands with eager commands
* corrected tests
* Update .gitignore
* Update .gitignore
Co-authored-by: JT <547158+jntrnr@users.noreply.github.com>
* Replace ansi-cut with ansi-str
There's no issues with it we just need to use it later.
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
* Fix color losing in string spliting into Sublines
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
To avoid resuggesting the same completion, add a space after commands or flags that have been accepted via `Enter`. Don't do that for filepaths or external completions
* Add append_whitespace choice for suggestion
Signed-off-by: gipsyh <gipsyh.icu@gmail.com>
* Fixed `test <path>` appending space.
* Update reedline
Co-authored-by: sholderbach <sholderbach@users.noreply.github.com>
* nu-cli/completions: fix paths with special chars
* add backticks
* fix replace
* added single quotes to check list
* check escape using fold
* fix clippy errors
* fix comment line
* fix conflicts
* change to vec
* skip sort checking
* removed invalid windows path
* remove comment
* added tests for escape function
* fix fn import
* fix fn import error
* test windows issue fix
* fix windows backslash path in the tests
* show expected path on error
* skip test for windows
* Added search terms to math commands
* Attempts to add ~user.
From: // Extend this to work with "~user" style of home paths
* Clippy recommendation
* clippy suggestions, again.
* fixing non-compilation on windows and macos
* fmt apparently does not like my imports
* even more clippy issues.
* less expect(), single conversion, match. Should work for MacOS too.
* Attempted to add functionality for windows: all it does is take the home path of current user, and replace the username.
* silly mistake in Windows version of user_home_dir()
* Update tilde.rs
* user_home_dir now returns a path instead of a string - should be smoother with no conversions to string
* clippy warnings
* clippy warnings 2
* Changed user_home_dir to return PathBuf now.
* Changed user_home_dir to return PathBuf now.
* forgot to fmt
* fixed windows build errors from modifying pathbuf but not returning it
* fixed windows clippy errors from returning () instead of pathbuf
* forgot to fmt
* borrowed path did not live long enough.
* previously, path.push did not work because rest_of_path started with "/" - it was not relative. Removing the / makes it a relative path again.
* Issue fixed.
* Update tilde.rs
* fmt.
* There is now a zero chance of panic. All expect()s have been removed.
* database commands
* db commands
* filesystem opens sqlite file
* clippy error
* corrected error in ci file
* removes matrix flag from ci
* flax matrix for clippy
* add conditional compile for tests
* add conditional compile for tests
* correct order of command
* correct error msg
* correct typo
Changes to reedline since `v0.4.0`:
- vi normal mode `I` for inserting at line beginning
- `InsertNewline` edit command that can be bound to `Alt-Enter` if
desired to have line breaks without relying on the `Validator`
- `ClearScreen` will directly clear the visible screen. `Signal::CtrlL` has been
removed.
- `ClearScrollback` will clear the screen and scrollback. Can be used to
mimic macOS `Cmd-K` screen clearing. Helps with #5089
* nu-cli: added tests for file completions
* test adding extra sort
* Feature/refactor completion options (#5228)
* Copy completion filter to custom completions
* Remove filter function from completer
This function was a no-op for FileCompletion and CommandCompletion.
Flag- and VariableCompletion just filters with `starts_with` which
happens in both completers anyway and should therefore also be a no-op.
The remaining use case in CustomCompletion was moved into the
CustomCompletion source file.
Filtering should probably happen immediately while fetching completions
to avoid unnecessary memory allocations.
* Add get_sort_by() to Completer trait
* Remove CompletionOptions from Completer::fetch()
* Fix clippy lints
* Apply Completer changes to DotNuCompletion
* add os to $nu based on rust's understanding (#5243)
* add os to $nu based on rust's understanding
* add a few more constants
Co-authored-by: Richard <Tropid@users.noreply.github.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
- With a change to reedline hints can now be hidden. This is useful when
no ANSI coloring is available as hints become indistinguishable from the
actual buffer
- remove commented out code
- order the logging calls according to the implementation
To remove a default keybinding for a particular edit mode, set the `event: null`:
e.g. to disable screen clearing with Ctrl-L
```
let $config = {keybindings: [{
modifier: control
keycode: char_l
mode: [emacs, vi_normal, vi_insert]
event: null
} ]}
```
* Remove unused packages from base Cargo.toml
* Remove unused crossterm_winapi from nu-cli
* Remove unused dependencies from nu-system
* Remove unused dependencies from nu-test-support
* Add timestamp flag to `touch` command
* Add modify flag to `touch` command
* Add date flag to `touch` command
* Remove unnecessary `touch` test and fix tests setups
* Change `touch` flags descriptions
* Update `touch` example
* Add reference flag to `touch` command
* Add access flag to `touch` command
* Add no-create flag to `touch` command
* Replace `unwrap` with `expect`
* updated to reedline generic menus
* help menu with examples
* generic menus in the engine
* description menu template
* list of menus in config
* default value for menu
* menu from block
* generic menus examples
* change to reedline git path
* cargo fmt
* menu name typo
* remove commas from default file
* added error message
Enables the use of some features on reedline
- Keeping the line when clearing the screen with `Ctrl-L`
- Using the internal cut buffer between lines
- Submitting external commands via keybinding and keeping the line
Additional effect:
Keep the history around and do basic syncs (performance improvement
minimal as session changes have to be read and written)
Additional change:
Give the option to defer writing/rereading the history file to the
closing of the session ($config.sync_history_on_enter)
* nu-completer with suggestions
* help menu with scrolling
* updates description rows based on space
* configuration for help menu
* update nu-ansi-term
* corrected test for update cells
* changed keybinding
* Refactor usage of is_perf_true to be a parameter passed around
* Move repl loop and command/script execution to nu_cli
* Move config setup out of nu_cli
* Update config_files.rs
* Update main.rs
Co-authored-by: JT <547158+jntrnr@users.noreply.github.com>
At the moment `crossterm` apparently has a regression decoding certain important key combinations on Windows.
Thus reedline reverted to the previous version.
Some changes are necessary to remove the need for `crossterm` in the use of `lscolors`.
Introduces two local conversion traits.
Additionally update the `Highlighter` API to support the cursor
position.
This will enable brace/statement match highlighting.