mirror of
https://github.com/nushell/nushell.git
synced 2024-10-16 23:33:49 +02:00
# Description As in other testing frameworks, the `setup` runs before every test case, and the `teardown` after that. A context can be created in `setup`, which will be in the `$in` variable in the test cases, and in the `teardown`. The `teardown` is called regardless of the test is passed, skipped, or failed. For example: ```nushell use std.nu * export def setup [] { log debug "Setup is running" {msg: "This is the context"} } export def teardown [] { log debug $"Teardown is running. Context: ($in)" } export def test_assert_pass [] { log debug $"Assert is running. Context: ($in)" } export def test_assert_skip [] { log debug $"Assert is running. Context: ($in)" assert skip } export def test_assert_fail_skipped_by_default [] { log debug $"Assert is running. Context: ($in)" assert false } ``` 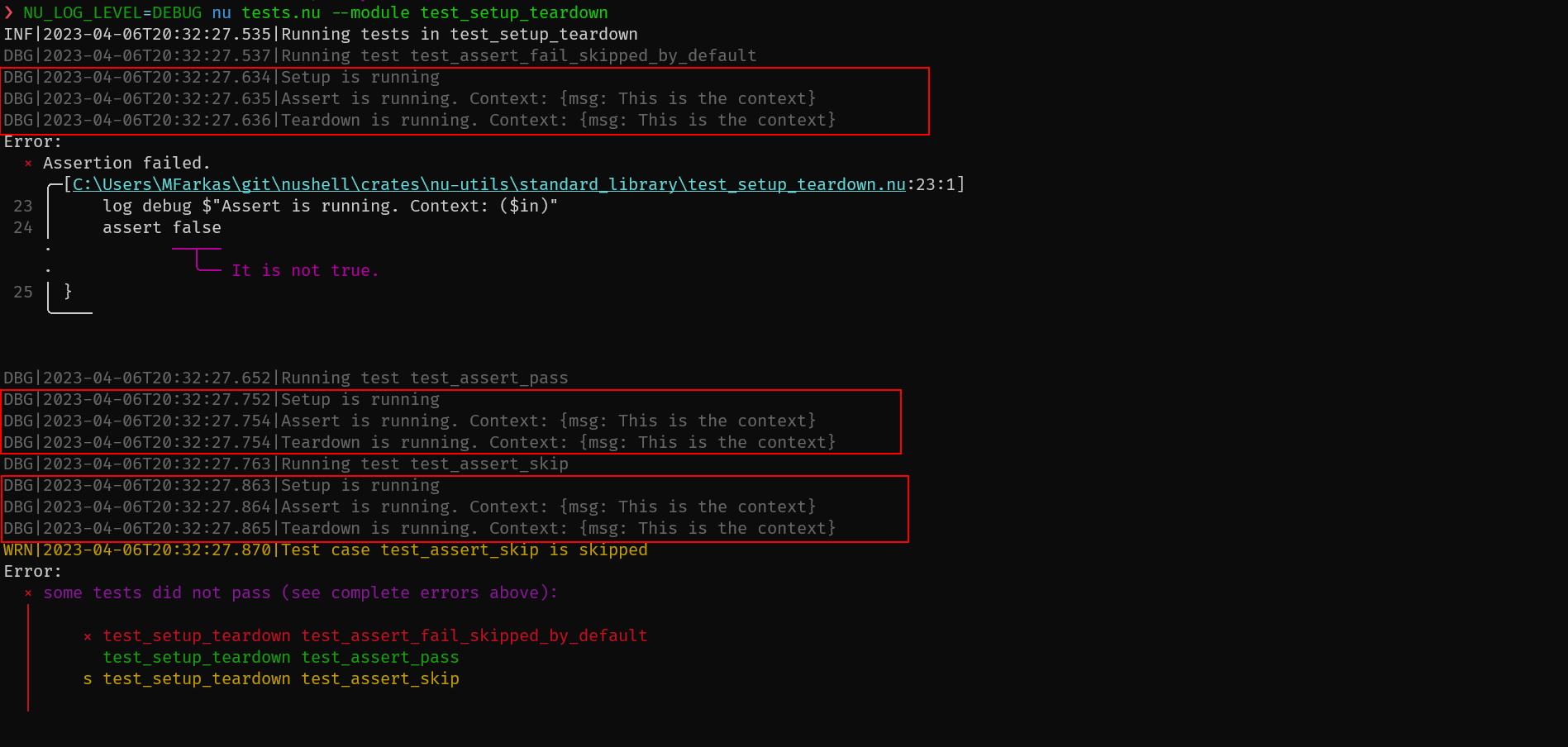 # After Submitting I'll update the documentation. --------- Co-authored-by: Mate Farkas <Mate.Farkas@oneidentity.com>
46 lines
2.3 KiB
Plaintext
46 lines
2.3 KiB
Plaintext
use std "xaccess"
|
|
use std "xupdate"
|
|
use std "xinsert"
|
|
use std "assert equal"
|
|
|
|
export def setup [] {
|
|
{sample_xml: ('
|
|
<a>
|
|
<b>
|
|
<c a="b"></c>
|
|
</b>
|
|
<c></c>
|
|
<d>
|
|
<e>z</e>
|
|
<e>x</e>
|
|
</d>
|
|
</a>' | from xml)
|
|
}
|
|
}
|
|
|
|
export def test_xml_xaccess [] {
|
|
let sample_xml = $in.sample_xml
|
|
|
|
assert equal ($sample_xml | xaccess [a]) [$sample_xml]
|
|
assert equal ($sample_xml | xaccess [*]) [$sample_xml]
|
|
assert equal ($sample_xml | xaccess [* d e]) [[tag, attributes, content]; [e, {}, [[tag, attributes, content]; [null, null, z]]], [e, {}, [[tag, attributes, content]; [null, null, x]]]]
|
|
assert equal ($sample_xml | xaccess [* d e 1]) [[tag, attributes, content]; [e, {}, [[tag, attributes, content]; [null, null, x]]]]
|
|
assert equal ($sample_xml | xaccess [* * * {|e| $e.attributes != {}}]) [[tag, attributes, content]; [c, {a: b}, []]]
|
|
}
|
|
|
|
export def test_xml_xupdate [] {
|
|
let sample_xml = $in.sample_xml
|
|
|
|
assert equal ($sample_xml | xupdate [*] {|x| $x | update attributes {i: j}}) ('<a i="j"><b><c a="b"></c></b><c></c><d><e>z</e><e>x</e></d></a>' | from xml)
|
|
assert equal ($sample_xml | xupdate [* d e *] {|x| $x | update content 'nushell'}) ('<a><b><c a="b"></c></b><c></c><d><e>nushell</e><e>nushell</e></d></a>' | from xml)
|
|
assert equal ($sample_xml | xupdate [* * * {|e| $e.attributes != {}}] {|x| $x | update content ['xml']}) {tag: a, attributes: {}, content: [[tag, attributes, content]; [b, {}, [[tag, attributes, content]; [c, {a: b}, [xml]]]], [c, {}, []], [d, {}, [[tag, attributes, content]; [e, {}, [[tag, attributes, content]; [null, null, z]]], [e, {}, [[tag, attributes, content]; [null, null, x]]]]]]}
|
|
}
|
|
|
|
export def test_xml_xinsert [] {
|
|
let sample_xml = $in.sample_xml
|
|
|
|
assert equal ($sample_xml | xinsert [a] {tag: b attributes:{} content: []}) ('<a><b><c a="b"></c></b><c></c><d><e>z</e><e>x</e></d><b></b></a>' | from xml)
|
|
assert equal ($sample_xml | xinsert [a d *] {tag: null attributes: null content: 'n'} | to xml) '<a><b><c a="b"></c></b><c></c><d><e>zn</e><e>xn</e></d></a>'
|
|
assert equal ($sample_xml | xinsert [a *] {tag: null attributes: null content: 'n'}) ('<a><b><c a="b"></c>n</b><c>n</c><d><e>z</e><e>x</e>n</d></a>' | from xml)
|
|
}
|