mirror of
https://github.com/nushell/nushell.git
synced 2024-10-17 07:42:53 +02:00
# Description Fixes #7510 . Remove support for tables from `to toml` command and update description. Previously, as indicated in #7510 , a table could be converted to toml and would result in this invalid toml: 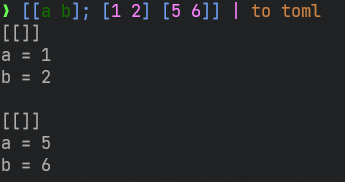 This commit removes functionality of serializing tables and now `to toml` produces an error: 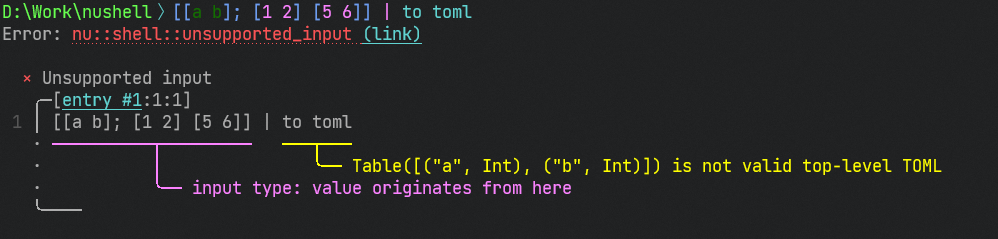 The `from toml` command already acknowledges the fact that toml can contain only records as indicated in its signature 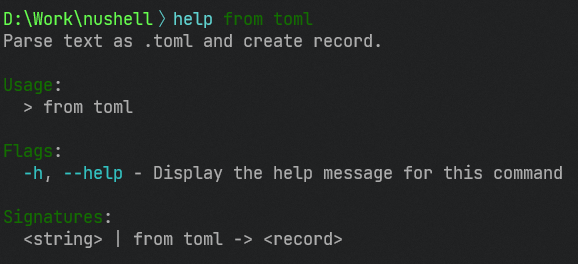 Now help of `to toml` reflects this feature of format as well: 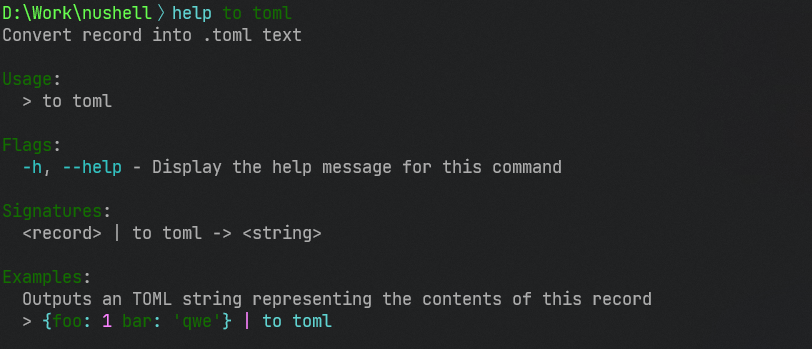 Additionally new tests were created for `to toml` command. See `crates\nu-command\tests\format_conversions\toml.rs`. Also removed undocumented behavior that would accept and validate a string as toml: 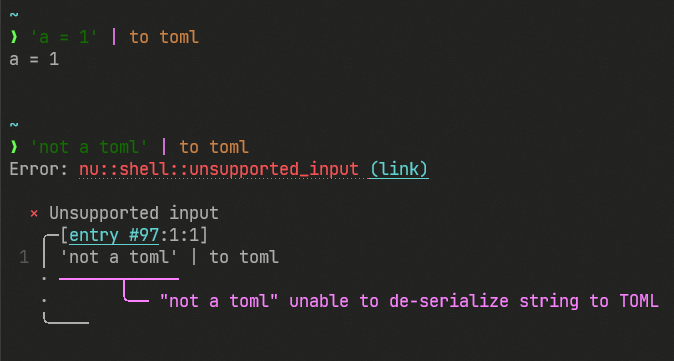 # User-Facing Changes - Serializing tables to toml now produces error instead of invalid toml - Updated `to toml` help - Remove undocumented "validation" (not really user-facing) # Tests + Formatting Don't forget to add tests that cover your changes. Make sure you've run and fixed any issues with these commands: - [x] `cargo fmt --all -- --check` to check standard code formatting (`cargo fmt --all` applies these changes) - [x] `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A clippy::needless_collect` to check that you're using the standard code style - [x] `cargo test --workspace` to check that all tests pass # After Submitting If your PR had any user-facing changes, update [the documentation](https://github.com/nushell/nushell.github.io) after the PR is merged, if necessary. This will help us keep the docs up to date.
103 lines
2.2 KiB
Rust
103 lines
2.2 KiB
Rust
use nu_test_support::{nu, pipeline};
|
|
|
|
#[test]
|
|
fn record_map_to_toml() {
|
|
let actual = nu!(
|
|
cwd: "tests/fixtures/formats", pipeline(
|
|
r#"
|
|
{a: 1 b: 2 c: 'qwe'}
|
|
| to toml
|
|
| from toml
|
|
| $in == {a: 1 b: 2 c: 'qwe'}
|
|
"#
|
|
));
|
|
|
|
assert_eq!(actual.out, "true");
|
|
}
|
|
|
|
#[test]
|
|
fn nested_records_to_toml() {
|
|
let actual = nu!(
|
|
cwd: "tests/fixtures/formats", pipeline(
|
|
r#"
|
|
{a: {a: a b: b} c: 1}
|
|
| to toml
|
|
| from toml
|
|
| $in == {a: {a: a b: b} c: 1}
|
|
"#
|
|
));
|
|
|
|
assert_eq!(actual.out, "true");
|
|
}
|
|
|
|
#[test]
|
|
fn records_with_tables_to_toml() {
|
|
let actual = nu!(
|
|
cwd: "tests/fixtures/formats", pipeline(
|
|
r#"
|
|
{a: [[a b]; [1 2] [3 4]] b: [[c d e]; [1 2 3]]}
|
|
| to toml
|
|
| from toml
|
|
| $in == {a: [[a b]; [1 2] [3 4]] b: [[c d e]; [1 2 3]]}
|
|
"#
|
|
));
|
|
|
|
assert_eq!(actual.out, "true");
|
|
}
|
|
|
|
#[test]
|
|
fn nested_tables_to_toml() {
|
|
let actual = nu!(
|
|
cwd: "tests/fixtures/formats", pipeline(
|
|
r#"
|
|
{c: [[f g]; [[[h k]; [1 2] [3 4]] 1]]}
|
|
| to toml
|
|
| from toml
|
|
| $in == {c: [[f g]; [[[h k]; [1 2] [3 4]] 1]]}
|
|
"#
|
|
));
|
|
|
|
assert_eq!(actual.out, "true");
|
|
}
|
|
|
|
#[test]
|
|
fn table_to_toml_fails() {
|
|
// Tables cant be represented in toml
|
|
let actual = nu!(
|
|
cwd: "tests/fixtures/formats", pipeline(
|
|
r#"
|
|
try { [[a b]; [1 2] [5 6]] | to toml | false } catch { true }
|
|
"#
|
|
));
|
|
|
|
assert_eq!(actual.out, "true");
|
|
}
|
|
|
|
#[test]
|
|
fn string_to_toml_fails() {
|
|
// Strings are not a top-level toml structure
|
|
let actual = nu!(
|
|
cwd: "tests/fixtures/formats", pipeline(
|
|
r#"
|
|
try { 'not a valid toml' | to toml | false } catch { true }
|
|
"#
|
|
));
|
|
|
|
assert_eq!(actual.out, "true");
|
|
}
|
|
|
|
#[test]
|
|
fn big_record_to_toml_text_and_from_toml_text_back_into_record() {
|
|
let actual = nu!(
|
|
cwd: "tests/fixtures/formats", pipeline(
|
|
r#"
|
|
open cargo_sample.toml
|
|
| to toml
|
|
| from toml
|
|
| get package.name
|
|
"#
|
|
));
|
|
|
|
assert_eq!(actual.out, "nu");
|
|
}
|