# Description
Adds `|` patterns to `match`, allowing you to try multiple patterns for
the same case.
Example:
```
match {b: 1} { {a: $b} | {b: $b} => { print $b } }
```
Variables that don't bind are set to `$nothing` so that they can be
later checked.
This PR also:
fixes#8631
Creates a set of integration tests for pattern matching also
# User-Facing Changes
Adds `|` to `match`. Fixes variable binding scope.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
> **Note**
> from `nushell` you can also use the `toolkit` as follows
> ```bash
> use toolkit.nu # or use an `env_change` hook to activate it
automatically
> toolkit check pr
> ```
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
This removes autoprinting the final value of a loop, much in the same
spirit as not autoprinting values at the end of statements. As we fix
these corner cases, it becomes more consistent that to print to the
screen in a script, you use the `print` command.
This gives a noticeable performance improvement as a bonus.
Before:
```
C:\Source\nushell〉 for x in 1..10 { $x }
1
2
3
4
5
6
7
8
9
10
```
Now:
```
C:\Source\nushell〉 for x in 1..10 { $x }
C:\Source\nushell〉
```
# User-Facing Changes
**BREAKING CHANGE**
Loops like `for`, `loop`, and `while` will no longer automatically print
loop values to the screen.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
> **Note**
> from `nushell` you can also use the `toolkit` as follows
> ```bash
> use toolkit.nu # or use an `env_change` hook to activate it
automatically
> toolkit check pr
> ```
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
Prior to this PR, the less/greater than operators (`<`, `>`, `<=`, `>=`)
would throw an error if either side was null. After this PR, these
operators return null if either side (or both) is null.
### Examples
```bash
1 < 3 # true
1 < null # null
null < 3 # null
null < null # null
```
### Motivation
JT [asked the C#
folks](https://discord.com/channels/601130461678272522/615329862395101194/1086137515053957140)
and this is apparently the approach they would choose for comparison
operators if they could start from scratch.
This PR makes `where` more convenient to use on jagged/missing data. For
example, we can now filter on columns that may not be present in every
row:
```
> [{foo: 123} {}] | where foo? > 10
╭───┬─────╮
│ # │ foo │
├───┼─────┤
│ 0 │ 123 │
╰───┴─────╯
```
# Description
This does a few speedups for tight loops:
* Caches the DeclId for `table` so we don't look it up. This means users
can't easily replace the default one, we might want to talk about this
tradeoff. The lookup for finding `table` in a tight loop is currently
pretty heavy. Might be another way to speed this up.
* `table` no longer pre-calculates the width. Instead, it only
calculates the width when printing a table or record.
* Use more efficient way of collecting the block of each loop
* When printing output, only get the config when needed
Combined, this drops the runtime from a million loop tight iteration
from 1sec 8ms to 236ms.
# User-Facing Changes
_(List of all changes that impact the user experience here. This helps
us keep track of breaking changes.)_
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
> **Note**
> from `nushell` you can also use the `toolkit` as follows
> ```bash
> use toolkit.nu # or use an `env_change` hook to activate it
automatically
> toolkit check pr
> ```
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
Some minor code cleanup.
We've accumulated a few macros over the years that arguably don't need
to be macros. This PR removes 4 macros by either:
1. Inlining the macro
2. Replacing the macro with a local function
3. Replacing the macro with a closure
# Description
Add float, string, and date patterns to matcher.
This could probably use some tests 😅
# User-Facing Changes
_(List of all changes that impact the user experience here. This helps
us keep track of breaking changes.)_
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
> **Note**
> from `nushell` you can also use the `toolkit` as follows
> ```bash
> use toolkit.nu # or use an `env_change` hook to activate it
automatically
> toolkit check pr
> ```
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
This moves the representation of variables on the stack to a Vec, which
more closely resembles a stack. For small numbers of variables live at
any one point, this tends to be more efficient than a HashMap. Having a
stack-like vector also allows us to remember a stack position,
temporarily push variables on, then quickly drop the stack back to the
original size when we're done. We'll need this capability to allow
matching inside of conditions.
On this mac, a simple run of:
`timeit { mut x = 1; while $x < 1000000 { $x += 1 } }`
Went from 1 sec 86 ms, down to 1 sec 2 ms. Clearly, we have a lot more
ground we can make up in looping speed 😅 but it's nice that for fixing
this to make matching easier, we also get a win in terms of lookup speed
for small numbers of variables.
# User-Facing Changes
Likely users won't (hopefully) see any negative impact and may even see
a small positive impact.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
> **Note**
> from `nushell` you can also use the `toolkit` as follows
> ```bash
> use toolkit.nu # or use an `env_change` hook to activate it
automatically
> toolkit check pr
> ```
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
Bumps [miette](https://github.com/zkat/miette) from 5.5.0 to 5.6.0.
<details>
<summary>Changelog</summary>
<p><em>Sourced from <a
href="https://github.com/zkat/miette/blob/main/CHANGELOG.md">miette's
changelog</a>.</em></p>
<blockquote>
<h2>5.6.0 (2023-03-14)</h2>
<h3>Bug Fixes</h3>
<ul>
<li><strong>ci:</strong> configure clippy-specific MSRV (<a
href="b658fc020b">b658fc02</a>)</li>
<li><strong>graphical:</strong> Fix wrong severity of related errors (<a
href="https://redirect.github.com/zkat/miette/issues/234">#234</a>) (<a
href="3497508aa9">3497508a</a>)</li>
<li><strong>atty:</strong> Switch out <code>atty</code> for
<code>is-terminal</code> (<a
href="https://redirect.github.com/zkat/miette/issues/229">#229</a>) (<a
href="443d240f49">443d240f</a>)</li>
</ul>
<h3>Features</h3>
<ul>
<li><strong>protocol:</strong> implement <code>Ord</code> for
<code>Severity</code> (<a
href="https://redirect.github.com/zkat/miette/issues/240">#240</a>) (<a
href="ed486c959d">ed486c95</a>)</li>
</ul>
<p><!-- raw HTML omitted --><!-- raw HTML omitted --></p>
</blockquote>
</details>
<details>
<summary>Commits</summary>
<ul>
<li><a
href="78fe18e699"><code>78fe18e</code></a>
chore: Release</li>
<li><a
href="2335b25ee7"><code>2335b25</code></a>
docs: update changelog</li>
<li><a
href="443d240f49"><code>443d240</code></a>
fix(atty): Switch out <code>atty</code> for <code>is-terminal</code> (<a
href="https://redirect.github.com/zkat/miette/issues/229">#229</a>)</li>
<li><a
href="ed486c959d"><code>ed486c9</code></a>
feat(protocol): implement <code>Ord</code> for <code>Severity</code> (<a
href="https://redirect.github.com/zkat/miette/issues/240">#240</a>)</li>
<li><a
href="3497508aa9"><code>3497508</code></a>
fix(graphical): Fix wrong severity of related errors (<a
href="https://redirect.github.com/zkat/miette/issues/234">#234</a>)</li>
<li><a
href="b658fc020b"><code>b658fc0</code></a>
fix(ci): configure clippy-specific MSRV</li>
<li><a
href="ebc61b5cf8"><code>ebc61b5</code></a>
docs: Mention miette::miette! macro under "... in application
code" (<a
href="https://redirect.github.com/zkat/miette/issues/233">#233</a>)</li>
<li><a
href="14f952dc91"><code>14f952d</code></a>
(cargo-release) start next development iteration 5.5.1-alpha.0</li>
<li><a
href="128c0a1fae"><code>128c0a1</code></a>
(cargo-release) start next development iteration 5.5.1-alpha.0</li>
<li>See full diff in <a
href="https://github.com/zkat/miette/compare/miette-derive-v5.5.0...miette-derive-v5.6.0">compare
view</a></li>
</ul>
</details>
<br />
[](https://docs.github.com/en/github/managing-security-vulnerabilities/about-dependabot-security-updates#about-compatibility-scores)
Dependabot will resolve any conflicts with this PR as long as you don't
alter it yourself. You can also trigger a rebase manually by commenting
`@dependabot rebase`.
[//]: # (dependabot-automerge-start)
[//]: # (dependabot-automerge-end)
---
<details>
<summary>Dependabot commands and options</summary>
<br />
You can trigger Dependabot actions by commenting on this PR:
- `@dependabot rebase` will rebase this PR
- `@dependabot recreate` will recreate this PR, overwriting any edits
that have been made to it
- `@dependabot merge` will merge this PR after your CI passes on it
- `@dependabot squash and merge` will squash and merge this PR after
your CI passes on it
- `@dependabot cancel merge` will cancel a previously requested merge
and block automerging
- `@dependabot reopen` will reopen this PR if it is closed
- `@dependabot close` will close this PR and stop Dependabot recreating
it. You can achieve the same result by closing it manually
- `@dependabot ignore this major version` will close this PR and stop
Dependabot creating any more for this major version (unless you reopen
the PR or upgrade to it yourself)
- `@dependabot ignore this minor version` will close this PR and stop
Dependabot creating any more for this minor version (unless you reopen
the PR or upgrade to it yourself)
- `@dependabot ignore this dependency` will close this PR and stop
Dependabot creating any more for this dependency (unless you reopen the
PR or upgrade to it yourself)
</details>
Signed-off-by: dependabot[bot] <support@github.com>
Co-authored-by: dependabot[bot] <49699333+dependabot[bot]@users.noreply.github.com>
# Description
This adds `match` and basic pattern matching.
An example:
```
match $x {
1..10 => { print "Value is between 1 and 10" }
{ foo: $bar } => { print $"Value has a 'foo' field with value ($bar)" }
[$a, $b] => { print $"Value is a list with two items: ($a) and ($b)" }
_ => { print "Value is none of the above" }
}
```
Like the recent changes to `if` to allow it to be used as an expression,
`match` can also be used as an expression. This allows you to assign the
result to a variable, eg) `let xyz = match ...`
I've also included a short-hand pattern for matching records, as I think
it might help when doing a lot of record patterns: `{$foo}` which is
equivalent to `{foo: $foo}`.
There are still missing components, so consider this the first step in
full pattern matching support. Currently missing:
* Patterns for strings
* Or-patterns (like the `|` in Rust)
* Patterns for tables (unclear how we want to match a table, so it'll
need some design)
* Patterns for binary values
* And much more
# User-Facing Changes
[see above]
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
> **Note**
> from `nushell` you can also use the `toolkit` as follows
> ```bash
> use toolkit.nu # or use an `env_change` hook to activate it
automatically
> toolkit check pr
> ```
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
This is a follow-up to https://github.com/nushell/nushell/pull/8379 and
https://github.com/nushell/nushell/discussions/8502.
This PR makes it so that the new `?` syntax for marking a path member as
optional short-circuits, as voted on in the
[8502](https://github.com/nushell/nushell/discussions/8502) poll.
Previously, `{ foo: 123 }.bar?.baz` would raise an error:
```
> { foo: 123 }.bar?.baz
× Data cannot be accessed with a cell path
╭─[entry #15:1:1]
1 │ { foo: 123 }.bar?.baz
· ─┬─
· ╰── nothing doesn't support cell paths
╰────
```
Here's what was happening:
1. The `bar?` path member access returns `nothing` because there is no field named `bar` on the record
2. The `baz` path member access fails when trying to access a `baz` field on that `nothing` value
After this change, `{ foo: 123 }.bar?.baz` returns `nothing`; the failed `bar?` access immediately returns `nothing` and the `baz` access never runs.
# Description
Add a `command_not_found` function to `$env.config.hooks`. If this
function outputs a string, then it's included in the `help`.
An example hook on *Arch Linux*, to find packages that contain the
binary, looks like:
```nushell
let-env config = {
# ...
hooks: {
command_not_found: {
|cmd_name| (
try {
let pkgs = (pkgfile --binaries --verbose $cmd_name)
(
$"(ansi $env.config.color_config.shape_external)($cmd_name)(ansi reset) " +
$"may be found in the following packages:\n($pkgs)"
)
} catch {
null
}
)
}
# ...
```
# User-Facing Changes
- Add a `command_not_found` function to `$env.config.hooks`.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
when running the tests inside nu-protocol we were getting a compiler
warning...
this PR removes the compiler warning from nu-protocol.
by adding
```rust
#[allow(unused_imports)]
```
Reverts nushell/nushell#8310
In anticipation that we may want to revert this PR. I'm starting the
process because of this issue.
This stopped working
```
let-env NU_LIB_DIRS = [
($nu.config-path | path dirname | path join 'scripts')
'C:\Users\username\source\repos\forks\nu_scripts'
($nu.config-path | path dirname)
]
```
You have to do this now instead.
```
const NU_LIB_DIRS = [
'C:\Users\username\AppData\Roaming\nushell\scripts'
'C:\Users\username\source\repos\forks\nu_scripts'
'C:\Users\username\AppData\Roaming\nushell'
]
```
In talking with @kubouch, he was saying that the `let-env` version
should keep working. Hopefully it's a small change.
# Description
Allow NU_LIBS_DIR and friends to be const they can be updated within the
same parse pass. This will allow us to remove having multiple config
files eventually.
Small implementation detail: I've changed `call.parser_info` to a
hashmap with string keys, so the information can have names rather than
indices, and we don't have to worry too much about the order in which we
put things into it.
Closes https://github.com/nushell/nushell/issues/8422
# User-Facing Changes
In a single file, users can now do stuff like
```
const NU_LIBS_DIR = ['/some/path/here']
source script.nu
```
and the source statement will use the value of NU_LIBS_DIR declared the
line before.
Currently, if there is no `NU_LIBS_DIR` const, then we fallback to using
the value of the `NU_LIBS_DIR` env-var, so there are no breaking changes
(unless someone named a const NU_LIBS_DIR for some reason).
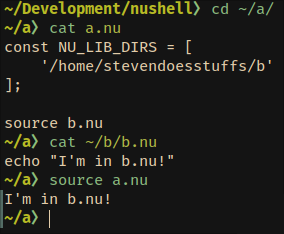
# Tests + Formatting
~~TODO: write tests~~ Done
# After Submitting
~~TODO: update docs~~ Will do when we update default_env.nu/merge
default_env.nu into default_config.nu.
# Description
_(Thank you for improving Nushell. Please, check our [contributing
guide](../CONTRIBUTING.md) and talk to the core team before making major
changes.)_
_(Description of your pull request goes here. **Provide examples and/or
screenshots** if your changes affect the user experience.)_
# User-Facing Changes
_(List of all changes that impact the user experience here. This helps
us keep track of breaking changes.)_
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
> **Note**
> from `nushell` you can also use the `toolkit` as follows
> ```bash
> use toolkit.nu # or use an `env_change` hook to activate it
automatically
> toolkit check pr
> ```
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
Resolves issue #8370
Adds the following flags to commands `from csv` and `from tsv`:
- `--flexible`: allow the number of fields in records to be variable
- `-c --comment`: a comment character to ignore lines starting with it
- `-q --quote`: a quote character to ignore separators in strings,
defaults to '\"'
- `-e --escape`: an escape character for strings containing the quote
character
Internally, the `Value` struct has an additional helper function
`as_char` which converts it to a single `char`
# User-Facing Changes
The single quoted string `'\t'` can no longer be used as a parameter for
the flag `--separator '\t'` as it is interpreted as a two-character
string. One needs to use from now on the flag with a double quoted
string like so: `-s "\t"` which correctly interprets the string as a
single `char`.
# Description
This fixes the `commandline` command when it's run with no arguments, so
it outputs the command being run. New line characters are included.
This allows for:
- [A way to get current command inside pre_execution hook · Issue #6264
· nushell/nushell](https://github.com/nushell/nushell/issues/6264)
- The possibility of *Atuin* to work work *Nushell*. *Atuin* hooks need
to know the current repl input before it is run.
# User-Facing Changes
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
This reverts commit dec0a2517f.
It breaks programs like `fzf`
# Description
Fixes: #8472
Fixes: #8313Reopen: #7690
# User-Facing Changes
_(List of all changes that impact the user experience here. This helps
us keep track of breaking changes.)_
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
> **Note**
> from `nushell` you can also use the `toolkit` as follows
> ```bash
> use toolkit.nu # or use an `env_change` hook to activate it
automatically
> toolkit check pr
> ```
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
This is a follow up from https://github.com/nushell/nushell/pull/7540.
Please provide feedback if you have the time!
## Summary
This PR lets you use `?` to indicate that a member in a cell path is
optional and Nushell should return `null` if that member cannot be
accessed.
Unlike the previous PR, `?` is now a _postfix_ modifier for cell path
members. A cell path of `.foo?.bar` means that `foo` is optional and
`bar` is not.
`?` does _not_ suppress all errors; it is intended to help in situations
where data has "holes", i.e. the data types are correct but something is
missing. Type mismatches (like trying to do a string path access on a
date) will still fail.
### Record Examples
```bash
{ foo: 123 }.foo # returns 123
{ foo: 123 }.bar # errors
{ foo: 123 }.bar? # returns null
{ foo: 123 } | get bar # errors
{ foo: 123 } | get bar? # returns null
{ foo: 123 }.bar.baz # errors
{ foo: 123 }.bar?.baz # errors because `baz` is not present on the result from `bar?`
{ foo: 123 }.bar.baz? # errors
{ foo: 123 }.bar?.baz? # returns null
```
### List Examples
```
〉[{foo: 1} {foo: 2} {}].foo
Error: nu:🐚:column_not_found
× Cannot find column
╭─[entry #30:1:1]
1 │ [{foo: 1} {foo: 2} {}].foo
· ─┬ ─┬─
· │ ╰── cannot find column 'foo'
· ╰── value originates here
╰────
〉[{foo: 1} {foo: 2} {}].foo?
╭───┬───╮
│ 0 │ 1 │
│ 1 │ 2 │
│ 2 │ │
╰───┴───╯
〉[{foo: 1} {foo: 2} {}].foo?.2 | describe
nothing
〉[a b c].4? | describe
nothing
〉[{foo: 1} {foo: 2} {}] | where foo? == 1
╭───┬─────╮
│ # │ foo │
├───┼─────┤
│ 0 │ 1 │
╰───┴─────╯
```
# Breaking changes
1. Column names with `?` in them now need to be quoted.
2. The `-i`/`--ignore-errors` flag has been removed from `get` and
`select`
1. After this PR, most `get` error handling can be done with `?` and/or
`try`/`catch`.
4. Cell path accesses like this no longer work without a `?`:
```bash
〉[{a:1 b:2} {a:3}].b.0
2
```
We had some clever code that was able to recognize that since we only
want row `0`, it's OK if other rows are missing column `b`. I removed
that because it's tricky to maintain, and now that query needs to be
written like:
```bash
〉[{a:1 b:2} {a:3}].b?.0
2
```
I think the regression is acceptable for now. I plan to do more work in
the future to enable streaming of cell path accesses, and when that
happens I'll be able to make `.b.0` work again.
# Description
The correction made here concerns the issue #8431. Indeed, the algorithm
initially proposed to remove elements of a `vector` performed a loop
with `remove` and an incident therefore appeared when several values
were equal because the deletion was done outside the length of the
vector:
```rust
let mut found = false;
for (i, col) in cols.clone().iter().enumerate() {
if col == col_name {
cols.remove(i);
vals.remove(i);
found = true;
}
}
```
Then, `[[a, a]; [1, 2]] | reject a: ` gave `thread 'main' panicked at
'removal index (is 1) should be < len (is 1)',
crates/nu-protocol/src/value/mod.rs:1213:54`.
The proposed correction is therefore the implementation of the
`retain_mut` utility dedicated to this functionality.
```rust
let mut found = false;
let mut index = 0;
cols.retain_mut(|col| {
if col == col_name {
found = true;
vals.remove(index);
false
} else {
index += 1;
true
}
});
```
# Description
Our `ShellError` at the moment has a `std::mem::size_of<ShellError>` of
136 bytes (on AMD64). As a result `Value` directly storing the struct
also required 136 bytes (thanks to alignment requirements).
This change stores the `Value::Error` `ShellError` on the heap.
Pro:
- Value now needs just 80 bytes
- Should be 1 cacheline less (still at least 2 cachelines)
Con:
- More small heap allocations when dealing with `Value::Error`
- More heap fragmentation
- Potential for additional required memcopies
# Further code changes
Includes a small refactor of `try` due to a type mismatch in its large
match.
# User-Facing Changes
None for regular users.
Plugin authors may have to update their matches on `Value` if they use
`nu-protocol`
Needs benchmarking to see if there is a benefit in real world workloads.
**Update** small improvements in runtime for workloads with high volume
of values. Significant reduction in maximum resident set size, when many
values are held in memory.
# Tests + Formatting
# Description
This works around a bug introduced by #8058
We should revisit the original fix, as it makes some assumptions about
how stdout redirection is used by `table`. We use the stdout by default
for table regularly during a repl session, so we should instead
special-case case for handling externals.
# User-Facing Changes
Restores the original default `table` behaviour for binary data
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
in https://github.com/nushell/nushell/issues/8311 and the discord
server, the idea of moving the default banner from the `rust` source to
the `nushell` standar library has emerged 😋
however, in order to do this, one need to have access to all the
variables used in the default banner => all of them are accessible
because known constants, except for the startup time of the shell, which
is not anywhere in the shell...
#### this PR adds exactly this, i.e. the new `startup_time` to the `$nu`
variable, which is computed to have the exact same value as the value
shown in the banner.
## the changes
in order to achieve this, i had to
- add `startup_time` as an `i64` to the `EngineState` => this is, to the
best of my knowledge, the easiest way to pass such an information around
down to where the banner startup time is computed and where the `$nu`
variable is evaluated
- add `startup-time` to the `$nu` variable and use the `EngineState`
getter for `startup_time` to show it as a `Value::Duration`
- pass `engine_state` as a `&mut`able argument from `main.rs` down to
`repl.rs` to allow the setter to change the value of `startup_time` =>
without this, the value would not change and would show `-1ns` as the
default value...
- the value of the startup time is computed in `evaluate_repl` in
`repl.rs`, only once at the beginning, and the same value is used in the
default banner 👌
# User-Facing Changes
one can now access to the same time as shown in the default banner with
```bash
$nu.startup-time
```
# Tests + Formatting
- 🟢 `cargo fmt --all`
- 🟢 `cargo clippy --workspace -- -D warnings -D
clippy::unwrap_used -A clippy::needless_collect`
- 🟢 `cargo test --workspace`
# After Submitting
```
$nothing
```
Add two rows in `$nu`, `$nu.is-interactive` and `$nu.is-login`, which
are true when nu is run in interactive and login mode respectively.
The `-i` flag now behaves a bit more like that of bash's, where the any
provided command or file is run without REPL but in "interactive mode".
This should entail sourcing interactive-mode config files, but since we
are planning on overhauling the config system soon, I'm holding off on
that. For now, all `-i` does is set `$nu.is-interactive` to be true.
About testing, I can't seem to find where cli-args get tested, so I
haven't written any new tests for this. Also I don't think there are any
docs that need updating. However if I'm wrong please tell me.
# Description
Hides https://github.com/nushell/nushell/pull/7925 from config and
disables by default. Option is still present in config, just hidden.
# User-Facing Changes
Users can no longer find `table.show_empty` in config and it is set to
false by default.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
Fix for data ambiguity noted in #8244.
Basic change is to use nanosecond resolution for unix timestamps (stored
in type Int). Previously, a timestamp might have seconds, milliseconds
or nanoseconds, but it turned out there were overlaps in data ranges
between different resolutions, so there wasn't always a unique mapping
back to date/time.
Due to higher precision, the *range* of dates that timestamps can map to
is restricted. Unix timestamps with seconds resolution and 64 bit
storage can cover all dates from the Big Bang to eternity. Timestamps
with seconds resolution and 32 bit storage can only represent dates from
1901-12-13 through 2038-01-19. The nanoseconds resolution and 64 bit
storage used with this fix can represent dates from 1677-09-21T00:12:44
to 2262-04-11T23:47:16, something of a compromise.
# User-Facing Changes
_(List of all changes that impact the user experience here. This helps
us keep track of breaking changes.)_
## `<datetime> | into int`
Converts to nanosecond resolution
```rust
〉date now | into int
1678084730502126846
```
This is the number of non-leap nanoseconds after the unix epoch date:
1970-01-01T00:00:00+00:00.
Conversion fails for dates outside the supported range:
```rust
〉1492-10-12 | into int
Error: nu:🐚:incorrect_value
× Incorrect value.
╭─[entry #51:1:1]
1 │ 1492-10-12 | into int
· ────┬───
· ╰── DateTime out of timestamp range 1677-09-21T00:12:43 and 2262-04-11T23:47:16
╰────
```
## `<int> | into datetime`
Can no longer fail or produce incorrect results for any 64-bit input:
```rust
〉0 | into datetime
Thu, 01 Jan 1970 00:00:00 +0000 (53 years ago)
〉"7fffffffffffffff" | into int -r 16 | into datetime
Fri, 11 Apr 2262 23:47:16 +0000 (in 239 years)
〉("7fffffffffffffff" | into int -r 16) * -1 | into datetime
Tue, 21 Sep 1677 00:12:43 +0000 (345 years ago)
```
## `<date> | date to-record` and `<date> | date to-table`
Now both have a `nanosecond` field.
```rust
〉"7fffffffffffffff" | into int -r 16 | into datetime | date to-record
╭────────────┬───────────╮
│ year │ 2262 │
│ month │ 4 │
│ day │ 11 │
│ hour │ 23 │
│ minute │ 47 │
│ second │ 16 │
│ nanosecond │ 854775807 │
│ timezone │ +00:00 │
╰────────────┴───────────╯
〉"7fffffffffffffff" | into int -r 16 | into datetime | date to-table
╭───┬──────┬───────┬─────┬──────┬────────┬────────┬────────────┬──────────╮
│ # │ year │ month │ day │ hour │ minute │ second │ nanosecond │ timezone │
├───┼──────┼───────┼─────┼──────┼────────┼────────┼────────────┼──────────┤
│ 0 │ 2262 │ 4 │ 11 │ 23 │ 47 │ 16 │ 854775807 │ +00:00 │
╰───┴──────┴───────┴─────┴──────┴────────┴────────┴────────────┴──────────╯
```
This change was not mandated by the OP problem, but it is nice to be
able to see the nanosecond bits that were present in Nushell `date` type
all along.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
Fixes#8341.
The `CustomValue::to_json()` function is an odd duck; it defaults to
returning `null`, and no `CustomValue` implementations override it to do
anything useful. I forgot to implement `to_json()` for `SQLiteDatabase`,
so `open foo.db | to json` was returning `null`.
To fix this, I've removed `CustomValue::to_json()` and now `to json`
will collect a `CustomValue` into a regular `Value` before doing a JSON
conversion.
Continuation of #8229 and #8326
# Description
The `ShellError` enum at the moment is kind of messy.
Many variants are basic tuple structs where you always have to reference
the implementation with its macro invocation to know which field serves
which purpose.
Furthermore we have both variants that are kind of redundant or either
overly broad to be useful for the user to match on or overly specific
with few uses.
So I set out to start fixing the lacking documentation and naming to
make it feasible to critically review the individual usages and fix
those.
Furthermore we can decide to join or split up variants that don't seem
to be fit for purpose.
# Call to action
**Everyone:** Feel free to add review comments if you spot inconsistent
use of `ShellError` variants.
# User-Facing Changes
(None now, end goal more explicit and consistent error messages)
# Tests + Formatting
(No additional tests needed so far)
# Commits (so far)
- Remove `ShellError::FeatureNotEnabled`
- Name fields on `SE::ExternalNotSupported`
- Name field on `SE::InvalidProbability`
- Name fields on `SE::NushellFailed` variants
- Remove unused `SE::NushellFailedSpannedHelp`
- Name field on `SE::VariableNotFoundAtRuntime`
- Name fields on `SE::EnvVarNotFoundAtRuntime`
- Name fields on `SE::ModuleNotFoundAtRuntime`
- Remove usused `ModuleOrOverlayNotFoundAtRuntime`
- Name fields on `SE::OverlayNotFoundAtRuntime`
- Name field on `SE::NotFound`
Continuation of #8229
# Description
The `ShellError` enum at the moment is kind of messy.
Many variants are basic tuple structs where you always have to reference
the implementation with its macro invocation to know which field serves
which purpose.
Furthermore we have both variants that are kind of redundant or either
overly broad to be useful for the user to match on or overly specific
with few uses.
So I set out to start fixing the lacking documentation and naming to
make it feasible to critically review the individual usages and fix
those.
Furthermore we can decide to join or split up variants that don't seem
to be fit for purpose.
**Everyone:** Feel free to add review comments if you spot inconsistent
use of `ShellError` variants.
- Name fields of `SE::IncorrectValue`
- Merge and name fields on `SE::TypeMismatch`
- Name fields on `SE::UnsupportedOperator`
- Name fields on `AssignmentRequires*` and fix doc
- Name fields on `SE::UnknownOperator`
- Name fields on `SE::MissingParameter`
- Name fields on `SE::DelimiterError`
- Name fields on `SE::IncompatibleParametersSingle`
# User-Facing Changes
(None now, end goal more explicit and consistent error messages)
# Tests + Formatting
(No additional tests needed so far)
# Description
The `ShellError` enum at the moment is kind of messy.
Many variants are basic tuple structs where you always have to reference
the implementation with its macro invocation to know which field serves
which purpose.
Furthermore we have both variants that are kind of redundant or either
overly broad to be useful for the user to match on or overly specific
with few uses.
So I set out to start fixing the lacking documentation and naming to
make it feasible to critically review the individual usages and fix
those.
Furthermore we can decide to join or split up variants that don't seem
to be fit for purpose.
Feel free to add review comments if you spot inconsistent use of
`ShellError` variants.
- Name fields on `ShellError::OperatorOverflow`
- Name fields on `ShellError::PipelineMismatch`
- Add doc to `ShellError::OnlySupportsThisInputType`
- Name `ShellError::OnlySupportsThisInputType`
- Name field on `ShellError::PipelineEmpty`
- Comment about issues with `TypeMismatch*`
- Fix a few `exp_input_type`s
- Name fields on `ShellError::InvalidRange`
# User-Facing Changes
(None now, end goal more explicit and consistent error messages)
# Tests + Formatting
(No additional tests needed so far)
# Description
This change fixes the bug associated with an incorrect span in
`type_mismatch` error message as described in #7288
The `span` argument in the method `into_value` was not being used to
convert a `PipelineData::Value` type so when called in
[eval_expression](https://github.com/nushell/nushell/blob/main/crates/nu-engine/src/eval.rs#L514-L515),
the original expression's span was not being used to overwrite the
result of `eval_subexpression`.
# User-Facing Changes
Using the example described in the issue, the whole bracketed
subexpression is correctly underlined.
Behavior before change:
```
let val = 10
($val | into string) + $val
Error: nu:🐚:type_mismatch
× Type mismatch during operation.
╭─[entry #2:1:1]
1 │ ($val | into string) + $val
· ─────┬───── ┬ ──┬─
· │ │ ╰── int
· │ ╰── type mismatch for operator
· ╰── string
╰────
```
Behavior after change:
```
let val = 10
($val | into string) + $val
Error: nu:🐚:type_mismatch
× Type mismatch during operation.
╭─[entry #2:1:1]
1 │ ($val | into string) + $val
· ──────────┬───────── ┬ ──┬─
· │ │ ╰── int
· │ ╰── type mismatch for operator
· ╰── string
╰────
```
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
This PR adds an alternative alias implementation. Old aliases still work
but you need to use `old-alias` instead of `alias`.
Instead of replacing spans in the original code and re-parsing, which
proved to be extremely error-prone and a constant source of panics, the
new implementation creates a new command that references the old
command. Consider the new alias defined as `alias ll = ls -l`. The
parser creates a new command called `ll` and remembers that it is
actually a `ls` command called with the `-l` flag. Then, when the parser
sees the `ll` command, it will translate it to `ls -l` and passes to it
any parameters that were passed to the call to `ll`. It works quite
similar to how known externals defined with `extern` are implemented.
The new alias implementation should work the same way as the old
aliases, including exporting from modules, referencing both known and
unknown externals. It seems to preserve custom completions and pipeline
metadata. It is quite robust in most cases but there are some rough
edges (see later).
Fixes https://github.com/nushell/nushell/issues/7648,
https://github.com/nushell/nushell/issues/8026,
https://github.com/nushell/nushell/issues/7512,
https://github.com/nushell/nushell/issues/5780,
https://github.com/nushell/nushell/issues/7754
No effect: https://github.com/nushell/nushell/issues/8122 (we might
revisit the completions code after this PR)
Should use custom command instead:
https://github.com/nushell/nushell/issues/6048
# User-Facing Changes
Since aliases are now basically commands, it has some new implications:
1. `alias spam = "spam"` (requires command call)
* **workaround**: use `alias spam = echo "spam"`
2. `def foo [] { 'foo' }; alias foo = ls -l` (foo defined more than
once)
* **workaround**: use different name (commands also have this
limitation)
4. `alias ls = (ls | sort-by type name -i)`
* **workaround**: Use custom command. _The common issue with this is
that it is currently not easy to pass flags through custom commands and
command referencing itself will lead to stack overflow. Both of these
issues are meant to be addressed._
5. TODO: Help messages, `which` command, `$nu.scope.aliases`, etc.
* Should we treat the aliases as commands or should they be separated
from regular commands?
6. Needs better error message and syntax highlight for recursed alias
(`alias f = f`)
7. Can't create alias with the same name as existing command (`alias ls
= ls -a`)
* Might be possible to add support for it (not 100% sure)
8. Standalone `alias` doesn't list aliases anymore
9. Can't alias parser keywords (e.g., stuff like `alias ou = overlay
use` won't work)
* TODO: Needs a better error message when attempting to do so
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
This PR addresses issue #2047 in order to enable autocomplete
functionality when using sudo for executing commands. I'e done a couple
of auxiliary checks such as ignoring whitespace and the last pipe in
order to determine the last command.
# User-Facing Changes
The only user facing change should be the autocomplete working.
# Tests + Formatting
All tests and formatting pass.
# Screenshots
<img width="454" alt="image"
src="https://user-images.githubusercontent.com/4399118/219404037-6cce4358-68a9-42bb-a09b-2986b10fa6cc.png">
# Suggestions welcome
I still don't know the in's and out's if nushell very well, any
suggestions for improvements are welcome.
# Description
Fixes#8002, which expands ranges `1..3` to expand to array-like when
saving and converting to json. Now,
```
> 1..3 | save foo.json
# foo.json
[
1,
2,
3
]
> 1..3 | to json
[
1,
2,
3
]
```
# User-Facing Changes
_(List of all changes that impact the user experience here. This helps
us keep track of breaking changes.)_
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- [X] `cargo fmt --all -- --check` to check standard code formatting
(`cargo fmt --all` applies these changes)
- [X] `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- [X] `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
issue #8167
Remove the `(link)` to the docs for `ShellError` and `ParseError`. As
per discussion on the issue, the nu-parser version didn't update on the
docs causing deadlinks for those errors, which brought the usefullness
of these links into question and it was decided to remove all of them
that `derive(diagnostic)`.
# User-Facing Changes
Before:
```
/home/rdevenney/projects/open_source/nushell〉ls | get name}
Error: nu::parser::unbalanced_delimiter (link)
× Unbalanced delimiter.
╭─[entry #1:1:1]
1 │ ls | get name}
· ▲
· ╰── unbalanced { and }
╰────
```
After:
```
/home/rdevenney/projects/open_source/nushell〉ls | get name}
Error: nu::parser::unbalanced_delimiter
× Unbalanced delimiter.
╭─[entry #1:1:1]
1 │ ls | get name}
· ▲
· ╰── unbalanced { and }
╰────
```
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
This PR fixes a bug where a default list in a custom command parameter
wasn't being accepted. The reason was because it was comparing specific
types of list like `list<any>` != `list<string>`. So, this PR attempts
to fix that.
### Before
```
> def f [param: list = [one]] { echo $param }
Error: nu::parser::assignment_mismatch (link)
× Default value wrong type
╭─[entry #1:1:1]
1 │ def f [param: list = [one]] { echo $param }
· ──┬──
· ╰── default value not list<any>
╰────
```
### After
```
> def f [param: list = [one]] {echo $param}
> f
╭───┬─────╮
│ 0 │ one │
╰───┴─────╯
```
closes#8092
# User-Facing Changes
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
Before landing:
- [x] `reedline 0.16.0` is out and pinned.
- [x] all fixes and features necessary are landed.
In the meantime:
- [ ] feed the release notes with relevant features and breaking changes
# Description
As title, I found this feature is useful to me too :)
Closes: #8039
# User-Facing Changes
```
❯ 3 * "ab"
ababab
❯ 3 * [1 2 3]
╭───┬───╮
│ 0 │ 1 │
│ 1 │ 2 │
│ 2 │ 3 │
│ 3 │ 1 │
│ 4 │ 2 │
│ 5 │ 3 │
│ 6 │ 1 │
│ 7 │ 2 │
│ 8 │ 3 │
╰───┴───╯
```
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.