# Description
As title, closes: #7921closes: #8273
# User-Facing Changes
when define a closure without pipe, nushell will raise error for now:
```
❯ let x = {ss ss}
Error: nu::parser::closure_missing_pipe
× Missing || inside closure
╭─[entry #2:1:1]
1 │ let x = {ss ss}
· ───┬───
· ╰── Parsing as a closure, but || is missing
╰────
help: Try add || to the beginning of closure
```
`any`, `each`, `all`, `where` command accepts closure, it forces user
input closure like `{||`, or parse error will returned.
```
❯ {major:2, minor:1, patch:4} | values | each { into string }
Error: nu::parser::closure_missing_pipe
× Missing || inside closure
╭─[entry #4:1:1]
1 │ {major:2, minor:1, patch:4} | values | each { into string }
· ───────┬───────
· ╰── Parsing as a closure, but || is missing
╰────
help: Try add || to the beginning of closure
```
`with-env`, `do`, `def`, `try` are special, they still remain the same,
although it says that it accepts a closure, but they don't need to be
written like `{||`, it's more likely a block but can capture variable
outside of scope:
```
❯ def test [input] { echo [0 1 2] | do { do { echo $input } } }; test aaa
aaa
```
Just realize that It's a big breaking change, we need to update config
and scripts...
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
This adds a new cell path literal syntax for use in any value position,
not just in a context where we expect a cell path.
This can be used to assign to a variable and then later use that
variable as a cell path.
Example:
```
> let cell_path = $.a.b
> {a: {b: 3}} | get $cell_path
3
```
# User-Facing Changes
This adds the syntax `$.a.b` to universally mean the cell path `a.b`,
even in a context that doesn't expect a cell path.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
> **Note**
> from `nushell` you can also use the `toolkit` as follows
> ```bash
> use toolkit.nu # or use an `env_change` hook to activate it
automatically
> toolkit check pr
> ```
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
Fix the recent parser panic with a single `{`
Introduced by https://github.com/nushell/nushell/pull/8470
# User-Facing Changes
_(List of all changes that impact the user experience here. This helps
us keep track of breaking changes.)_
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
> **Note**
> from `nushell` you can also use the `toolkit` as follows
> ```bash
> use toolkit.nu # or use an `env_change` hook to activate it
automatically
> toolkit check pr
> ```
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
Turning off implicit echo got tested before some of these changes, so
bring a few tests in-line with that functionality.
# User-Facing Changes
_(List of all changes that impact the user experience here. This helps
us keep track of breaking changes.)_
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
> **Note**
> from `nushell` you can also use the `toolkit` as follows
> ```bash
> use toolkit.nu # or use an `env_change` hook to activate it
automatically
> toolkit check pr
> ```
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
This fixes the `commandline` command when it's run with no arguments, so
it outputs the command being run. New line characters are included.
This allows for:
- [A way to get current command inside pre_execution hook · Issue #6264
· nushell/nushell](https://github.com/nushell/nushell/issues/6264)
- The possibility of *Atuin* to work work *Nushell*. *Atuin* hooks need
to know the current repl input before it is run.
# User-Facing Changes
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
https://github.com/orhun/halp finds the correct help command
by checking if `cmd --help` succeeds.
All standard utilities I've tried exit successfully on `--help`,
but not nushell.
This is a follow up from https://github.com/nushell/nushell/pull/7540.
Please provide feedback if you have the time!
## Summary
This PR lets you use `?` to indicate that a member in a cell path is
optional and Nushell should return `null` if that member cannot be
accessed.
Unlike the previous PR, `?` is now a _postfix_ modifier for cell path
members. A cell path of `.foo?.bar` means that `foo` is optional and
`bar` is not.
`?` does _not_ suppress all errors; it is intended to help in situations
where data has "holes", i.e. the data types are correct but something is
missing. Type mismatches (like trying to do a string path access on a
date) will still fail.
### Record Examples
```bash
{ foo: 123 }.foo # returns 123
{ foo: 123 }.bar # errors
{ foo: 123 }.bar? # returns null
{ foo: 123 } | get bar # errors
{ foo: 123 } | get bar? # returns null
{ foo: 123 }.bar.baz # errors
{ foo: 123 }.bar?.baz # errors because `baz` is not present on the result from `bar?`
{ foo: 123 }.bar.baz? # errors
{ foo: 123 }.bar?.baz? # returns null
```
### List Examples
```
〉[{foo: 1} {foo: 2} {}].foo
Error: nu:🐚:column_not_found
× Cannot find column
╭─[entry #30:1:1]
1 │ [{foo: 1} {foo: 2} {}].foo
· ─┬ ─┬─
· │ ╰── cannot find column 'foo'
· ╰── value originates here
╰────
〉[{foo: 1} {foo: 2} {}].foo?
╭───┬───╮
│ 0 │ 1 │
│ 1 │ 2 │
│ 2 │ │
╰───┴───╯
〉[{foo: 1} {foo: 2} {}].foo?.2 | describe
nothing
〉[a b c].4? | describe
nothing
〉[{foo: 1} {foo: 2} {}] | where foo? == 1
╭───┬─────╮
│ # │ foo │
├───┼─────┤
│ 0 │ 1 │
╰───┴─────╯
```
# Breaking changes
1. Column names with `?` in them now need to be quoted.
2. The `-i`/`--ignore-errors` flag has been removed from `get` and
`select`
1. After this PR, most `get` error handling can be done with `?` and/or
`try`/`catch`.
4. Cell path accesses like this no longer work without a `?`:
```bash
〉[{a:1 b:2} {a:3}].b.0
2
```
We had some clever code that was able to recognize that since we only
want row `0`, it's OK if other rows are missing column `b`. I removed
that because it's tricky to maintain, and now that query needs to be
written like:
```bash
〉[{a:1 b:2} {a:3}].b?.0
2
```
I think the regression is acceptable for now. I plan to do more work in
the future to enable streaming of cell path accesses, and when that
happens I'll be able to make `.b.0` work again.
# Description
The `spam` command is not always going to be missing, as is the case
with this sgml tool: https://linux.die.net/man/1/spam
Rename `spam` and `foo` to something more unique so we have a lower
chance of colliding with other names on the system.
fixes#8404
# User-Facing Changes
No impact. This only impacts testing
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
Fixes the crash when handing `{}#.}`
# User-Facing Changes
_(List of all changes that impact the user experience here. This helps
us keep track of breaking changes.)_
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
in https://github.com/nushell/nushell/issues/8311 and the discord
server, the idea of moving the default banner from the `rust` source to
the `nushell` standar library has emerged 😋
however, in order to do this, one need to have access to all the
variables used in the default banner => all of them are accessible
because known constants, except for the startup time of the shell, which
is not anywhere in the shell...
#### this PR adds exactly this, i.e. the new `startup_time` to the `$nu`
variable, which is computed to have the exact same value as the value
shown in the banner.
## the changes
in order to achieve this, i had to
- add `startup_time` as an `i64` to the `EngineState` => this is, to the
best of my knowledge, the easiest way to pass such an information around
down to where the banner startup time is computed and where the `$nu`
variable is evaluated
- add `startup-time` to the `$nu` variable and use the `EngineState`
getter for `startup_time` to show it as a `Value::Duration`
- pass `engine_state` as a `&mut`able argument from `main.rs` down to
`repl.rs` to allow the setter to change the value of `startup_time` =>
without this, the value would not change and would show `-1ns` as the
default value...
- the value of the startup time is computed in `evaluate_repl` in
`repl.rs`, only once at the beginning, and the same value is used in the
default banner 👌
# User-Facing Changes
one can now access to the same time as shown in the default banner with
```bash
$nu.startup-time
```
# Tests + Formatting
- 🟢 `cargo fmt --all`
- 🟢 `cargo clippy --workspace -- -D warnings -D
clippy::unwrap_used -A clippy::needless_collect`
- 🟢 `cargo test --workspace`
# After Submitting
```
$nothing
```
Add two rows in `$nu`, `$nu.is-interactive` and `$nu.is-login`, which
are true when nu is run in interactive and login mode respectively.
The `-i` flag now behaves a bit more like that of bash's, where the any
provided command or file is run without REPL but in "interactive mode".
This should entail sourcing interactive-mode config files, but since we
are planning on overhauling the config system soon, I'm holding off on
that. For now, all `-i` does is set `$nu.is-interactive` to be true.
About testing, I can't seem to find where cli-args get tested, so I
haven't written any new tests for this. Also I don't think there are any
docs that need updating. However if I'm wrong please tell me.
startup nushell with no config file or env file...
This PR gives the ability to start up nushell easily with no config or
env config files
simply by passing in
```rust
nu -n
```
or
```rust
nu --no-config-file
```
A bonus is that startup times for nushell decreases FIVE fold...
From about > 50ms to less than < 10ms on average on my mac
This will enable Part II which will hopefully be the ability to
to send this flag into the nu! macro and turn off loading of the config
files...
Remember when config files are enabled the nu-cmd-lang tests fail
because the
commands in the config files are a superset of the commands in
nu-cmd-lang...
In my preliminary tests before by zeroing out the config files the
nu-cmd-lang tests passed...
Independent of the cratification efforts I have always wanted a way
anyway to turn off loading
the config files when starting up nushell... So this accomplishes that
task...
Continuation of #8229
# Description
The `ShellError` enum at the moment is kind of messy.
Many variants are basic tuple structs where you always have to reference
the implementation with its macro invocation to know which field serves
which purpose.
Furthermore we have both variants that are kind of redundant or either
overly broad to be useful for the user to match on or overly specific
with few uses.
So I set out to start fixing the lacking documentation and naming to
make it feasible to critically review the individual usages and fix
those.
Furthermore we can decide to join or split up variants that don't seem
to be fit for purpose.
**Everyone:** Feel free to add review comments if you spot inconsistent
use of `ShellError` variants.
- Name fields of `SE::IncorrectValue`
- Merge and name fields on `SE::TypeMismatch`
- Name fields on `SE::UnsupportedOperator`
- Name fields on `AssignmentRequires*` and fix doc
- Name fields on `SE::UnknownOperator`
- Name fields on `SE::MissingParameter`
- Name fields on `SE::DelimiterError`
- Name fields on `SE::IncompatibleParametersSingle`
# User-Facing Changes
(None now, end goal more explicit and consistent error messages)
# Tests + Formatting
(No additional tests needed so far)
# Description
Removes the `env` command, as the `$env` is generally a much better
experience.
# User-Facing Changes
Breaking change: Removes `env`.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
This PR adds an alternative alias implementation. Old aliases still work
but you need to use `old-alias` instead of `alias`.
Instead of replacing spans in the original code and re-parsing, which
proved to be extremely error-prone and a constant source of panics, the
new implementation creates a new command that references the old
command. Consider the new alias defined as `alias ll = ls -l`. The
parser creates a new command called `ll` and remembers that it is
actually a `ls` command called with the `-l` flag. Then, when the parser
sees the `ll` command, it will translate it to `ls -l` and passes to it
any parameters that were passed to the call to `ll`. It works quite
similar to how known externals defined with `extern` are implemented.
The new alias implementation should work the same way as the old
aliases, including exporting from modules, referencing both known and
unknown externals. It seems to preserve custom completions and pipeline
metadata. It is quite robust in most cases but there are some rough
edges (see later).
Fixes https://github.com/nushell/nushell/issues/7648,
https://github.com/nushell/nushell/issues/8026,
https://github.com/nushell/nushell/issues/7512,
https://github.com/nushell/nushell/issues/5780,
https://github.com/nushell/nushell/issues/7754
No effect: https://github.com/nushell/nushell/issues/8122 (we might
revisit the completions code after this PR)
Should use custom command instead:
https://github.com/nushell/nushell/issues/6048
# User-Facing Changes
Since aliases are now basically commands, it has some new implications:
1. `alias spam = "spam"` (requires command call)
* **workaround**: use `alias spam = echo "spam"`
2. `def foo [] { 'foo' }; alias foo = ls -l` (foo defined more than
once)
* **workaround**: use different name (commands also have this
limitation)
4. `alias ls = (ls | sort-by type name -i)`
* **workaround**: Use custom command. _The common issue with this is
that it is currently not easy to pass flags through custom commands and
command referencing itself will lead to stack overflow. Both of these
issues are meant to be addressed._
5. TODO: Help messages, `which` command, `$nu.scope.aliases`, etc.
* Should we treat the aliases as commands or should they be separated
from regular commands?
6. Needs better error message and syntax highlight for recursed alias
(`alias f = f`)
7. Can't create alias with the same name as existing command (`alias ls
= ls -a`)
* Might be possible to add support for it (not 100% sure)
8. Standalone `alias` doesn't list aliases anymore
9. Can't alias parser keywords (e.g., stuff like `alias ou = overlay
use` won't work)
* TODO: Needs a better error message when attempting to do so
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
Should close#8035.
> **Note**
> this is my first technical PR for `nushell`
> - i might very well miss things
> - i tried to be as complete as possible about the changes
> - please require further changes if i did something wrong, i'm open to
any remark 😌
# Description
this PR adds, when it is defined in the `examples` method of the
`Command` implementations, the output of the examples to the output of
the `help` command.
this PR
- only modifies `crates/nu-engine/src/documentation.rs` and the
`get_documentation` function
- defines a new `WD` constant to print a **W**hite **D**immed `...`
- a `match` statement at the end of the example loop to
- print a white dimmed `...` when the example is not set, i.e. set to
`None` in the `examples` method of the `Command` implementation of a
command
- pretty print the output of the associated example `Value` when it has
been defined
> **Warning**
> LIMITATIONS:
> - i use snippets from `crates/nu-protocol/src/pipeline_data.rs`
> - the table creation from `pub PipelineData::print`, i.e. the `let
decl_id = ...;` and `let table = ...;` in the change
> - the table item printing from `PipelineData::write_all_and_flush`,
i.e. the `for item in table { ... }`
>
> ADDRESSED:
> - ~~the formatting of the output is not perfect and has to be fully
left aligned with the first column for now~~ (fixed with
[`5abeefd558c34ba9bae15e2f183ff4625442921e`..`a62be1b5a2c730959da5dbc028bb91ffe5093f63`](5abeefd558c34ba9bae15e2f183ff4625442921e..a62be1b5a2c730959da5dbc028bb91ffe5093f63))
> - ~~i'm using `.unwrap()` on both the changes above, not sure how to
handle this for now~~ (fixed for now thanks to 49f1dc080)
> - ~~the tests and `clippy` checks do not pass for now, see below~~
(`clippy` now is happy with 49f1dc080 and the tests pass with
11666bc715)
# User-Facing Changes
the output of the `help <command>` command is now augmented with the
outputs of the examples, when they are defined.
- `with-env`
```bash
> help with-env
...
Examples:
Set the MYENV environment variable
> with-env [MYENV "my env value"] { $env.MYENV }
my env value
Set by primitive value list
> with-env [X Y W Z] { $env.X }
Y
Set by single row table
> with-env [[X W]; [Y Z]] { $env.W }
Z
Set by key-value record
> with-env {X: "Y", W: "Z"} { [$env.X $env.W] }
╭───┬───╮
│ 0 │ Y │
│ 1 │ Z │
╰───┴───╯
```
instead of the previous
```bash
> help with-env
...
Examples:
Set the MYENV environment variable
> with-env [MYENV "my env value"] { $env.MYENV }
Set by primitive value list
> with-env [X Y W Z] { $env.X }
Set by single row table
> with-env [[X W]; [Y Z]] { $env.W }
Set by key-value record
> with-env {X: "Y", W: "Z"} { [$env.X $env.W] }
```
- `merge`
```bash
> help merge
...
Examples:
Add an 'index' column to the input table
> [a b c] | wrap name | merge ( [1 2 3] | wrap index )
╭───┬──────╮
│ # │ name │
├───┼──────┤
│ 1 │ a │
│ 2 │ b │
│ 3 │ c │
╰───┴──────╯
Merge two records
> {a: 1, b: 2} | merge {c: 3}
╭───┬───╮
│ a │ 1 │
│ b │ 2 │
│ c │ 3 │
╰───┴───╯
Merge two tables, overwriting overlapping columns
> [{columnA: A0 columnB: B0}] | merge [{columnA: 'A0*'}]
╭───┬─────────┬─────────╮
│ # │ columnA │ columnB │
├───┼─────────┼─────────┤
│ 0 │ A0* │ B0 │
╰───┴─────────┴─────────╯
```
instead of the previous
```bash
> help merge
...
Examples:
Add an 'index' column to the input table
> [a b c] | wrap name | merge ( [1 2 3] | wrap index )
Merge two records
> {a: 1, b: 2} | merge {c: 3}
Merge two tables, overwriting overlapping columns
> [{columnA: A0 columnB: B0}] | merge [{columnA: 'A0*'}]
```
# Description
`bytes starts-with` converts the input into a `Value` before running
.starts_with to find if the binary matches. This has two side effects:
it makes the code simpler, only dealing in whole values, and simplifying
a lot of input pipeline handling and value transforming it would
otherwise have to do. _Especially_ in the presence of a cell path to
drill into. It also makes buffers the entire input into memory, which
can take up a lot of memory when dealing with large files, especially if
you only want to check the first few bytes (like for a magic number).
This PR adds a special branch on PipelineData::ExternalStream with a
streaming version of starts_with.
# User-Facing Changes
Opening large files and running bytes starts-with on them will not take
a long time.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# Drawbacks
Streaming checking is more complicated, and there may be bugs. I tested
it with multiple chunks with string data and binary data and it seems to
work alright up to 8k and over bytes, though.
The existing `operate` method still exists because the way it handles
cell paths and values is complicated. This causes some "code
duplication", or at least some intent duplication, between the value
code and the streaming code. This might be worthwhile considering the
performance gains (approaching infinity on larger inputs).
Another thing to consider is that my ExternalStream branch considers
string data as valid input. The operate branch only parses Binary
values, so it would fail. `open` is kind of unpredictable on whether it
returns string data or binary data, even when passing `--raw`. I think
this can be a problem but not really one I'm trying to tackle in this
PR, so, it's worth considering.
Fixes#7615
# Description
When calling external commands, we create a table from the pipeline data
to handle external commands expecting paginated input. When a binary
value is made into a table, we convert the vector of bytes representing
the binary bytes into a pretty formatted string. This results in the
pretty formatted string being sent to external commands instead of the
actual binary bytes. By checking whether the stdout of the call is being
redirected, we can decide whether to send the raw binary bytes or the
pretty formatted output when creating a table command.
# User-Facing Changes
When passing binary values to external commands, the external command
will receive the actual bytes instead of the pretty printed string. Use
cases that don't involve piping a binary value into an external command
are unchanged.
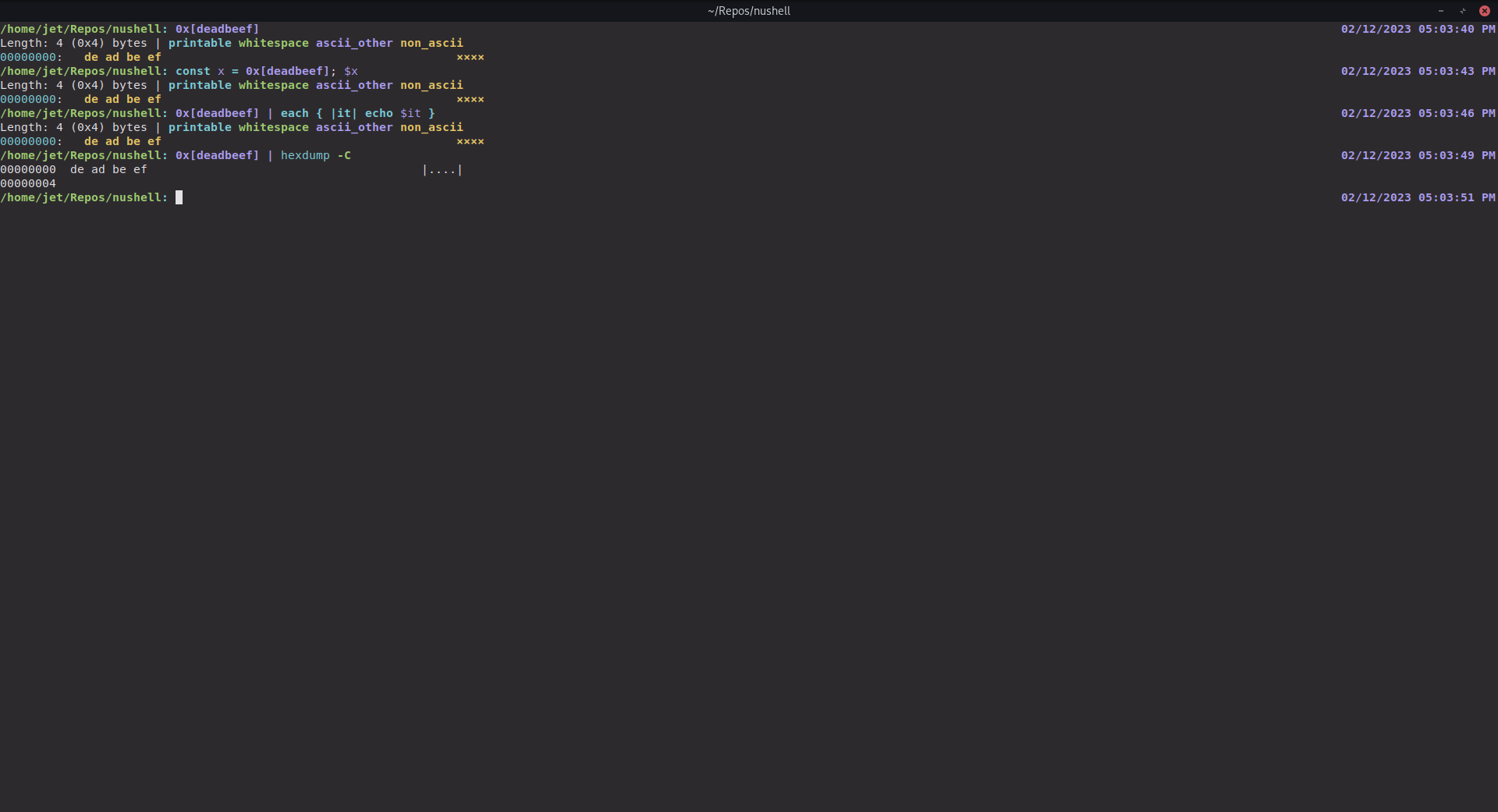
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
cargo fmt --all -- --check to check standard code formatting (cargo fmt
--all applies these changes)
cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect to check that you're using the standard code
style
cargo test --workspace to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
This one fixes env not being hidden inside closure, reported in the
conversation under https://github.com/nushell/nushell/issues/6593https://github.com/nushell/nushell/issues/6593https://github.com/nushell/nushell/issues/7937 still persist. These
seems a bit more involved and might need hidden env tracking also in the
engine state... I'm not yet sure what's causing it.
Also re-enables some env-related tests and removes unused Value clone.
# User-Facing Changes
Just a bugfix
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
The point of this command is to allow you to be able to format ints,
floats, filesizes, and strings with an alignment, padding, and a fill
character, as strings. It's meant to take the place of `str lpad` and
`str rpad`.
```
> help fill
Fill and Align
Search terms: display, render, format, pad, align
Usage:
> fill {flags}
Flags:
-h, --help - Display the help message for this command
-w, --width <Int> - The width of the output. Defaults to 1
-a, --alignment <String> - The alignment of the output. Defaults to Left (Left(l), Right(r), Center(c/m), MiddleRight(cr/mr))
-c, --character <String> - The character to fill with. Defaults to ' ' (space)
Signatures:
<number> | fill -> <string>
<string> | fill -> <string>
Examples:
Fill a string on the left side to a width of 15 with the character '─'
> 'nushell' | fill -a l -c '─' -w 15
Fill a string on the right side to a width of 15 with the character '─'
> 'nushell' | fill -a r -c '─' -w 15
Fill a string on both sides to a width of 15 with the character '─'
> 'nushell' | fill -a m -c '─' -w 15
Fill a number on the left side to a width of 5 with the character '0'
> 1 | fill --alignment right --character 0 --width 5
Fill a filesize on the left side to a width of 5 with the character '0'
> 1kib | fill --alignment middle --character 0 --width 10
```
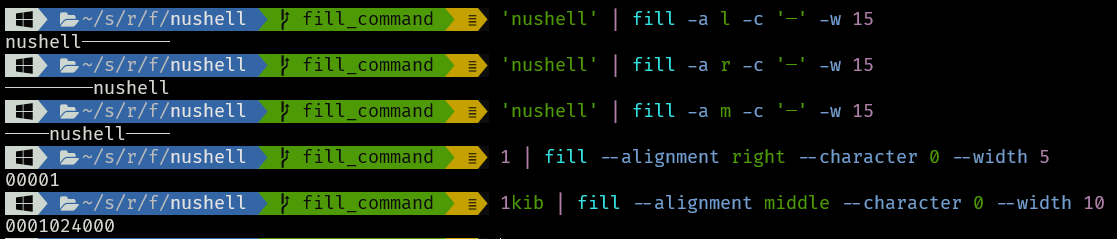
# User-Facing Changes
Deprecated `str lpad` and `str rpad`.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
As usual, Rust optimizes a bit less if more function boundaries are
introduced.
However, in my opinion, being able to comprehend the decision tree beats
a couple of string allocations.
# Description
_(Thank you for improving Nushell. Please, check our [contributing
guide](../CONTRIBUTING.md) and talk to the core team before making major
changes.)_
I opened this PR to unify the run command method. It's mainly to improve
consistency across the tree.
# User-Facing Changes
None.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
Remove `--numbered` from ~~`for`~~, `each`, `par-each`, `reduce` and
`each while`. These all provide indexes (numbering) via the optional
second param to their closures.
EDIT: Closes#6986.
# User-Facing Changes
Every command that had `--numbered` listed as "deprecated" in their help
docs is affected.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
---------
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
# Description
Use the `use_ansi_coloring` configuration point to decide whether the
output will have colors, where possible.
Related: https://github.com/nushell/nushell/issues/7676

- [x] `grid -c`
- [x] `perf()`
# User-Facing Changes
_(List of all changes that impact the user experience here. This helps
us keep track of breaking changes.)_
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
Fixes#7301.
# User-Facing Changes
`return` can now be used in scripts without explicit `def main`.
# Tests + Formatting
Don't forget to add tests that cover your changes. (I'm not sure how to
test this.)
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
Lint: `clippy::uninlined_format_args`
More readable in most situations.
(May be slightly confusing for modifier format strings
https://doc.rust-lang.org/std/fmt/index.html#formatting-parameters)
Alternative to #7865
# User-Facing Changes
None intended
# Tests + Formatting
(Ran `cargo +stable clippy --fix --workspace -- -A clippy::all -D
clippy::uninlined_format_args` to achieve this. Depends on Rust `1.67`)
# Description
Support extended unicode escapes in strings with same syntax as Rust:
`"\u{6e}"`.
# User-Facing Changes
New syntax in string literals, `\u{NNNNNN}`, to go along with the
existing `\uNNNN`.
New syntax accepts 1-6 hex digits and rejects values greater than
0x10FFFF (max Unicode char)..
_(List of all changes that impact the user experience here. This helps
us keep track of breaking changes.)_
Won't break existing scripts, since this is new syntax.
We might consider deprecating `char -u`, since users can now embed
unicode chars > 0xFFFF with the new escape.
# Tests + Formatting
Several unit tests and one integration test added.
- [x] `cargo fmt --all -- --check` to check standard code formatting
(`cargo fmt --all` applies these changes)
Done
- [x] `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
Done
- [x] `cargo test --workspace` to check that all tests pass
Done
# After Submitting
- [ ] If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
This PR changes the old performance logging with `Instant` timers. I'm
not sure if this is the best way to do it but it does help reveal where
time is being spent on startup. This is what it looks like when you
launch nushell with `cargo run -- --log-level info`. I'm using the
`info` log level exclusively for performance monitoring at this point.
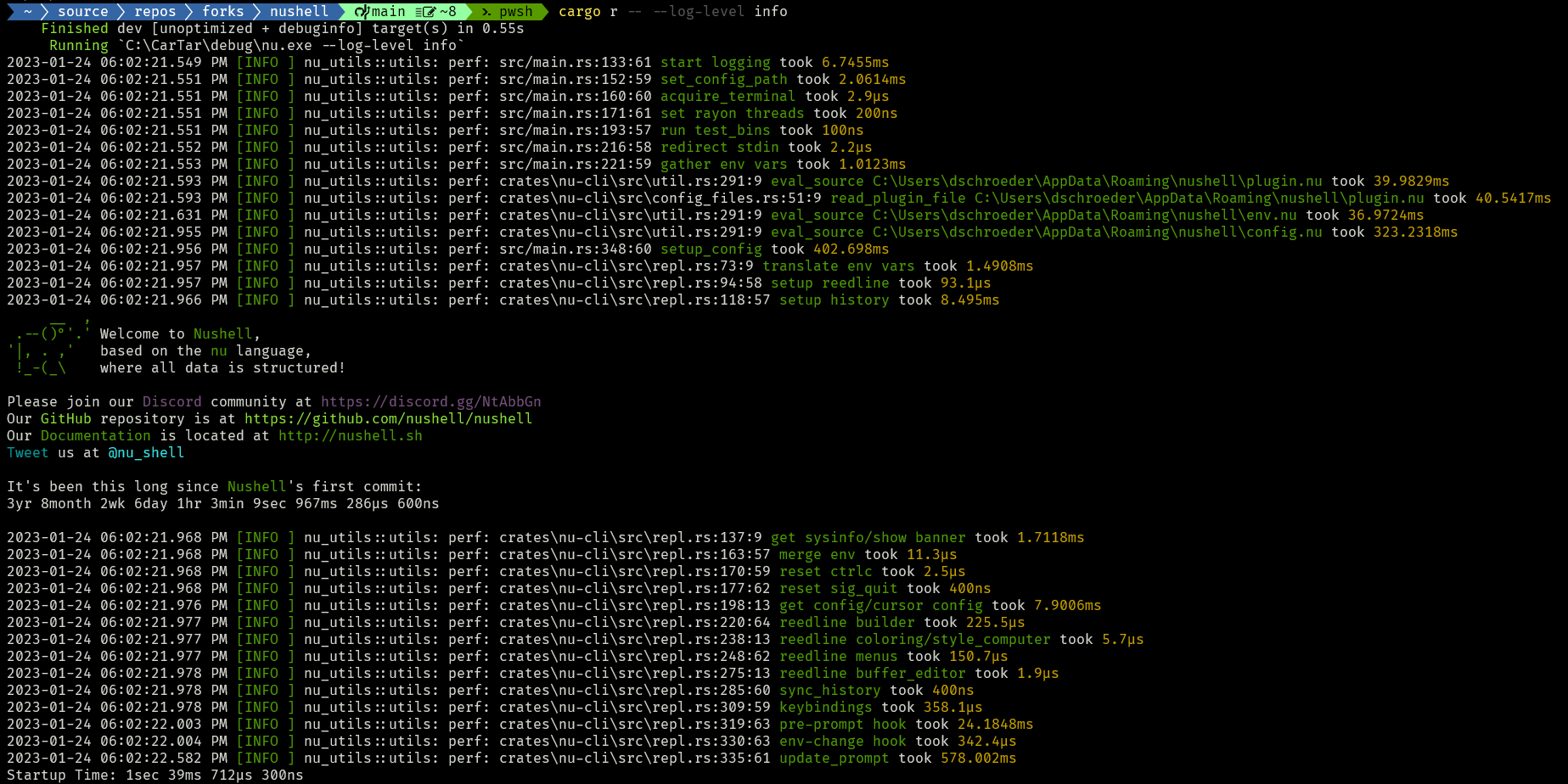
## After Startup
Since you're in the repl, you can continue running commands. Here's the
output of `ls`, for instance.
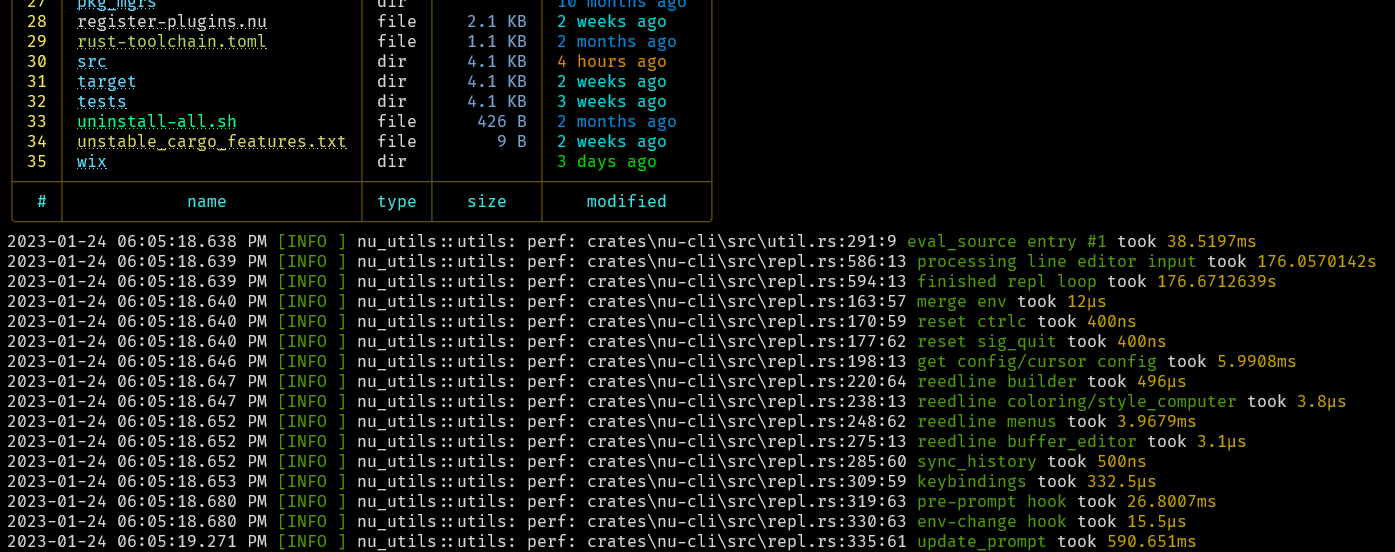
Note that the above screenshots are in debug mode, so they're much
slower than release.
# User-Facing Changes
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
This PR shows the startup time and decreases the banner. This startup
time output can be disabled with the `show_banner: false` setting in the
config. This is the startup in debug mode.
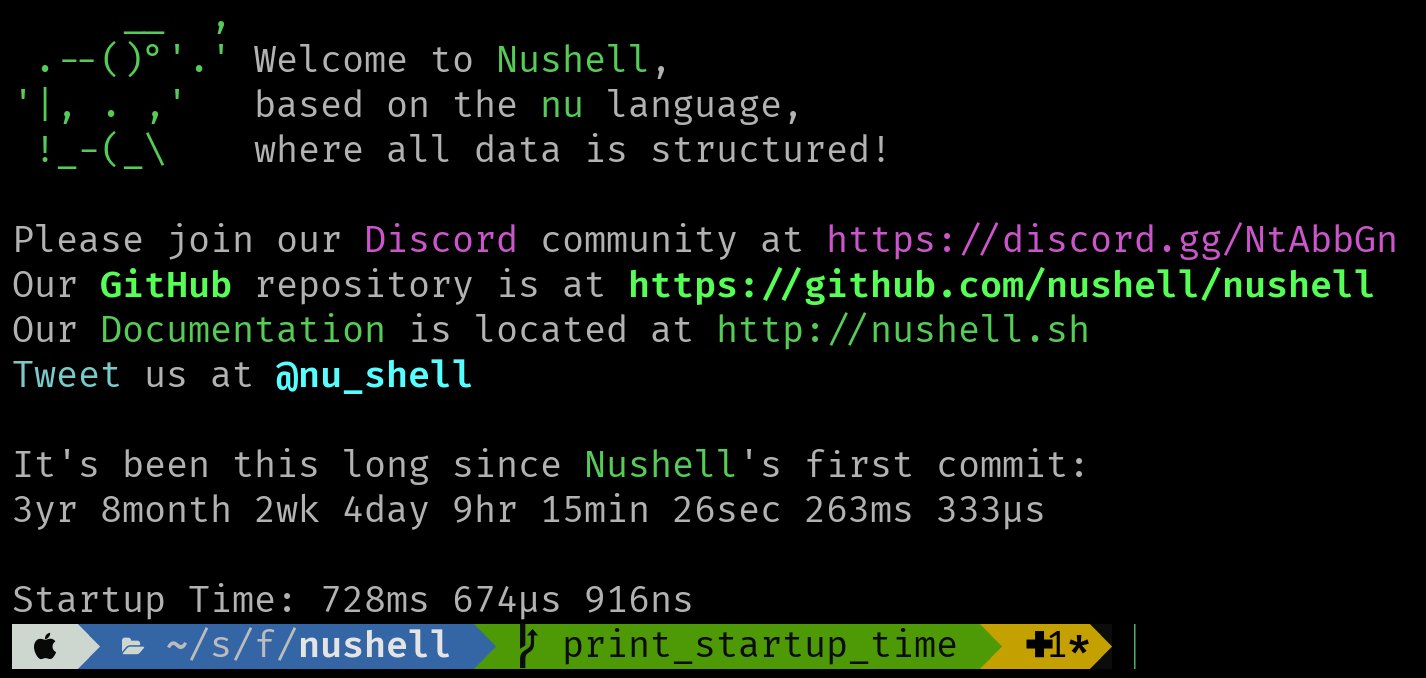
On my mac in release mode
```
Startup Time: 368ms 429µs 83ns
```
On my mac without a config as `nu --config foo --env-config foo`
```
Startup Time: 11ms 663µs 791ns
```
I could really go either way on this. If people don't like this change,
we don't have to merge it.
# User-Facing Changes
Startup Time
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
This PR makes changes that allow underscores in numbers.
Example:
```nu
# allows underscores to be placed arbitrarily to enhance readability.
let pi = 3.1415_9265_3589_793
# works with integers
let num = 1_000_000_000_000
let fav_color = 0x68_9d_6a
```
# Description
_(Description of your pull request goes here. **Provide examples and/or
screenshots** if your changes affect the user experience.)_
I implemented the status bar we talk about yesterday. The idea was
inspired by the progress bar of `wget`.
I decided to go for the second suggestion by `@Reilly`
> 2. add an Option<usize> or whatever to RawStream (and ListStream?) for
situations where you do know the length ahead of time
For now only works with the command `save` but after the approve of this
PR we can see how we can implement it on commands like `cp` and `mv`
When using `fetch` nushell will check if there is any `content-length`
attribute in the request header. If so, then `fetch` will send it
through the new `Option` variable in the `RawStream` to the `save`.
If we know the total size we show the progress bar

but if we don't then we just show the stats like: data already saved,
bytes per second, and time lapse.


Please let me know If I need to make any changes and I will be happy to
do it.
# User-Facing Changes
A new flag (`--progress` `-p`) was added to the `save` command
Examples:
```nu
fetch https://github.com/torvalds/linux/archive/refs/heads/master.zip | save --progress -f main.zip
fetch https://releases.ubuntu.com/22.04.1/ubuntu-22.04.1-desktop-amd64.iso | save --progress -f main.zip
open main.zip --raw | save --progress main.copy
```
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
-
I am getting some errors and its weird because the errors are showing up
in files i haven't touch. Is this normal?
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
Co-authored-by: Reilly Wood <reilly.wood@icloud.com>
src/main.rs has a dependency on BufferedReader
which is currently located in nu_command.
I am moving BufferedReader to a more relevant
location (crate) which will allow / eliminate main's dependency
on nu_command in a benchmark / testing environment...
now that @rgwood has landed benches I want
to start experimenting with benchmarks related
to the parser.
For benchmark purposes when dealing with parsing
you need a very simple set of commands that show
how well the parser is doing, in other words
just the core commands... Not all of nu_command...
Having a smaller nu binary when running the benchmark CI
would enable building nushell quickly, yet still show us
how well the parser is performing...
Once this PR lands the only dependency main will have
on nu_command is create_default_context ---
meaning for benchmark purposes we can swap in a tiny
crate of commands instead of the gigantic nu_command
which has its "own" create_default_context...
It will also enable other crates going forward to
use BufferedReader. Right now it is not accessible
to other lower level crates because it is located in a
"top of the stack crate".
Add recursion limit to `def` and `block`.
Summary of this PR , it will detect if `def` call itself or not .
Then execute by using `stack` which I think best choice to use with this
design and core as it is available in all crates and mutable and
calculate the recursion limit on calling `def`.
Set 50 as recursion limit on `Config`.
Add some tests too .
Fixes#5899
Co-authored-by: Reilly Wood <reilly.wood@icloud.com>
# Description
Closes#7554
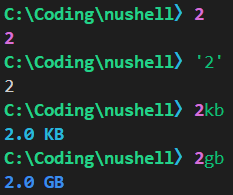
# User-Facing Changes
See above.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
Co-authored-by: Reilly Wood <26268125+rgwood@users.noreply.github.com>
The ordering of flags in `nu --help` was a bit of a mess; I think it
grew organically over time. Related commands (like
`--config`/`--env-config`/`--plugin-config`) weren't grouped together,
common flags weren't near the top, and we weren't following alphabetical
ordering.
### Before
```bash
Flags:
-h, --help - Display the help message for this command
--stdin - redirect standard input to a command (with `-c`) or a script file
-l, --login - start as a login shell
-i, --interactive - start as an interactive shell
-v, --version - print the version
--testbin <String> - run internal test binary
-c, --commands <String> - run the given commands and then exit
--config <String> - start with an alternate config file
--env-config <String> - start with an alternate environment config file
--log-level <String> - log level for diagnostic logs (error, warn, info, debug, trace). Off by default
--log-target <String> - set the target for the log to output. stdout, stderr(default), mixed or file
-e, --execute <String> - run the given commands and then enter an interactive shell
-t, --threads <Int> - threads to use for parallel commands
-m, --table-mode <String> - the table mode to use. rounded is default.
--plugin-config <String> - start with an alternate plugin signature file
```
### After
```bash
Flags:
-h, --help - Display the help message for this command
-c, --commands <String> - run the given commands and then exit
-e, --execute <String> - run the given commands and then enter an interactive shell
-i, --interactive - start as an interactive shell
-l, --login - start as a login shell
-m, --table-mode <String> - the table mode to use. rounded is default.
-t, --threads <Int> - threads to use for parallel commands
-v, --version - print the version
--config <String> - start with an alternate config file
--env-config <String> - start with an alternate environment config file
--plugin-config <String> - start with an alternate plugin signature file
--log-level <String> - log level for diagnostic logs (error, warn, info, debug, trace). Off by default
--log-target <String> - set the target for the log to output. stdout, stderr(default), mixed or file
--stdin - redirect standard input to a command (with `-c`) or a script file
--testbin <String> - run internal test binary
```
The new ordering:
1. Groups commands with short flags together, sorted alphabetically by
short flag
1. Groups commands with only long flags together, sorted alphabetically
(with the exception of `--plugin-config` so we can keep related flags
together)
Conveniently, this puts the very commonly used `-c` at the top and the
very rarely used `--testbin` at the bottom.
# Description
This closes#7498, as well as fixes an issue reported in
https://github.com/nushell/nushell/pull/7002#issuecomment-1368340773
BEFORE:
```
〉[{foo: 'bar'} {}] | get foo
Error: nu:🐚:column_not_found (link)
× Cannot find column
╭─[entry #5:1:1]
1 │ [{foo: 'bar'} {}] | get foo
· ────────┬──────── ─┬─
· │ ╰── value originates here
· ╰── cannot find column 'Empty cell'
╰────
〉[{foo: 'bar'} {}].foo
╭───┬─────╮
│ 0 │ bar │
│ 1 │ │
╰───┴─────╯
```
AFTER:
```
〉[{foo: 'bar'} {}] | get foo
Error: nu:🐚:column_not_found (link)
× Cannot find column
╭─[entry #1:1:1]
1 │ [{foo: 'bar'} {}] | get foo
· ─┬ ─┬─
· │ ╰── cannot find column 'foo'
· ╰── value originates here
╰────
〉[{foo: 'bar'} {}].foo
Error: nu:🐚:column_not_found (link)
× Cannot find column
╭─[entry #3:1:1]
1 │ [{foo: 'bar'} {}].foo
· ─┬ ─┬─
· │ ╰── cannot find column 'foo'
· ╰── value originates here
╰────
```
EDIT: This also changes the semantics of `get`/`select` `-i` somewhat.
I've decided to leave it like this because it works more intuitively
with `default` and `compact`.
BEFORE:
```
〉[{a:1} {b:2} {a:3}] | select -i foo | to nuon
null
```
AFTER:
```
〉[{a:1} {b:2} {a:3}] | select -i foo | to nuon
[[foo]; [null], [null], [null]]
```
# User-Facing Changes
See above. EDIT: the issue with holes in cases like ` [{foo: 'bar'}
{}].foo.0` versus ` [{foo: 'bar'} {}].0.foo` has been resolved.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
Closes#7273.
Also slightly edits/tidies up parser.rs.
# User-Facing Changes
`^` is now forbidden in `def` and `def-env` command names. EDIT: also
`alias`.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
Inspired by #7592
For brevity use `Value::test_{string,int,float,bool}`
Includes fixes to commands that were abusing `Span::test_data` in their
implementation. Now the call span is used where possible or the explicit
`Span::unknonw` is used.
## Command fixes
- Fix abuse of `Span::test_data()` in `query_xml`
- Fix abuse of `Span::test_data()` in `term size`
- Fix abuse of `Span::test_data()` in `seq date`
- Fix two abuses of `Span::test_data` in `nu-cli`
- Change `Span::test_data` to `Span::unknown` in `keybindings listen`
- Add proper call span to `registry query`
- Fix span use in `nu_plugin_query`
- Fix span assignment in `select`
- Use `Span::unknown` instead of `test_data` in more places
## Other
- Use `Value::test_int`/`test_float()` consistently
- More `test_string` and `test_bool`
- Fix unused imports
# User-Facing Changes
Some commands may now provide more helpful spans for downstream use in
errors
# Description
Purely for consistency, various remaining instances of `$nothing`
(almost all of which were in test code) have been changed to `null`.
Now, the only place that refers to `$nothing` is the parser code which
implements it.
# User-Facing Changes
The default config.nu now uses `null` in certain places where it used
`$nothing`.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
Just change the description of the `--stdin` flag as shown in `nu
--help`:
"redirect the stdin" -> "redirect standard input to a command (with
`-c`) or a script file"
The old description was a little too terse and I had to look in the code
to see what it was doing.
# Description
Env config can be mutated by `=`, this pr is to add a few tests
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
This PR changes some commands that previously accepted row conditions
(like `$it > 5`) as parameter to accept closures instead. The reasons
are:
a) The commands would need to move into parser keywords in the future
while they feel more like commands to be implemented in Nushell code as
a part of standard library.
b) In scripts, it is useful to store the predicate condition in a
variable which you can't do with row conditions.
c) These commands are not used that often to benefit enough from the
shorter row condition syntax
# User-Facing Changes
The following commands now accept **closure** instead of a **row
condition**:
- `take until`
- `take while`
- `skip until`
- `skip while`
- `any`
- `all`
This is a part of an effort to move away from shape-directed parsing.
Related PR: https://github.com/nushell/nushell/pull/7365
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
Fixes https://github.com/nushell/nushell/issues/6708
The error message of environment variable not found could change
depending on the `$env` content which can produce random failures on
different systems. This PR hopefully makes the tests more resilient.
# User-Facing Changes
None
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
Address part of feature request #7337, add a small command `into
cellpath` to allow string -> cellpath auto-conversion, with this change,
we could run
```
let p = 'ls.use_ls_colors'
$env.config | upsert ($p | nito cellpath) false
```
<img width="710" alt="image"
src="https://user-images.githubusercontent.com/85712372/206782818-3024b34f-150b-482d-aebc-9426ef6a1cf9.png">
Note - This pr only covers `String` -> `CellPath`, any other conversions
should be considered as expected?
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- [x] `cargo fmt --all -- --check` to check standard code formatting
(`cargo fmt --all` applies these changes)
- [x] `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- [x] `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
While perusing Value.rs, I noticed the `Value::int()`, `Value::float()`,
`Value::boolean()` and `Value::string()` constructors, which seem
designed to make it easier to construct various Values, but which aren't
used often at all in the codebase. So, using a few find-replaces
regexes, I increased their usage. This reduces overall LOC because
structures like this:
```
Value::Int {
val: a,
span: head
}
```
are changed into
```
Value::int(a, head)
```
and are respected as such by the project's formatter.
There are little readability concerns because the second argument to all
of these is `span`, and it's almost always extremely obvious which is
the span at every callsite.
# User-Facing Changes
None.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
Adds improved errors for when a user uses a bashism that nu doesn't
support.
fixes#7237
Examples:
```
Error: nu::parser::shell_andand (link)
× The '&&' operator is not supported in Nushell
╭─[entry #1:1:1]
1 │ ls && ls
· ─┬
· ╰── instead of '&&', use ';' or 'and'
╰────
help: use ';' instead of the shell '&&', or 'and' instead of the boolean '&&'
```
```
Error: nu::parser::shell_oror (link)
× The '||' operator is not supported in Nushell
╭─[entry #8:1:1]
1 │ ls || ls
· ─┬
· ╰── instead of '||', use 'try' or 'or'
╰────
help: use 'try' instead of the shell '||', or 'or' instead of the boolean '||'
```
```
Error: nu::parser::shell_err (link)
× The '2>' shell operation is 'err>' in Nushell.
╭─[entry #9:1:1]
1 │ foo 2> bar.txt
· ─┬
· ╰── use 'err>' instead of '2>' in Nushell
╰────
```
```
Error: nu::parser::shell_outerr (link)
× The '2>&1' shell operation is 'out+err>' in Nushell.
╭─[entry #10:1:1]
1 │ foo 2>&1 bar.txt
· ──┬─
· ╰── use 'out+err>' instead of '2>&1' in Nushell
╰────
help: Nushell redirection will write all of stdout before stderr.
```
# User-Facing Changes
**BREAKING CHANGES**
This removes the `&&` and `||` operators. We previously supported by
`&&`/`and` and `||`/`or`. With this change, only `and` and `or` are
valid boolean operators.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
fixes#7384
This is a stop-gap fix until we remove type-directed parsing. With this,
you can create a `OneOf` shape that can be one of a list of syntax
shapes. This gives you a little more control than you get with `Any`,
allowing you to add `Block` without breaking other parsing rules.
# User-Facing Changes
`else` block will no longer capture variables as it will now use a block
instead of a closure.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
This fix changes pipelines to allow them to actually be empty. Mapping
over empty pipelines gives empty pipelines. Empty pipelines immediately
return `None` when iterated.
This removes a some of where `Span::new(0, 0)` was coming from, though
there are other cases where we still use it.
# User-Facing Changes
None
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
We already have the binary `bit-xor` and the shortcircuiting logical
`or`(`||`) and `and`(`&&`).
This introduces `xor` as a compact form for both brevity and clarity.
You can express the operation through `not`/`and`/`or` with a slight
risk of introducing bugs through typos.
Operator precedence
`and` > `xor` > `or`
Added logic and precedence tests.
# Description
As title, when execute external sub command, auto-trimming end
new-lines, like how fish shell does.
And if the command is executed directly like: `cat tmp`, the result
won't change.
Fixes: #6816Fixes: #3980
Note that although nushell works correctly by directly replace output of
external command to variable(or other places like string interpolation),
it's not friendly to user, and users almost want to use `str trim` to
trim trailing newline, I think that's why fish shell do this
automatically.
If the pr is ok, as a result, no more `str trim -r` is required when
user is writing scripts which using external commands.
# User-Facing Changes
Before:
<img width="523" alt="img"
src="https://user-images.githubusercontent.com/22256154/202468810-86b04dbb-c147-459a-96a5-e0095eeaab3d.png">
After:
<img width="505" alt="img"
src="https://user-images.githubusercontent.com/22256154/202468599-7b537488-3d6b-458e-9d75-d85780826db0.png">
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace --features=extra -- -D warnings -D
clippy::unwrap_used -A clippy::needless_collect` to check that you're
using the standard code style
- `cargo test --workspace --features=extra` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
This adds new pipeline connectors called out> and err> which redirect either stdout or stderr to a file. You can also use out+err> (or err+out>) to redirect both streams into a file.
Alters `all`, `any`, `each while`, `each`, `insert`, `par-each`, `reduce`, `update`, `upsert` and `where`,
so that their blocks take an optional parameter containing the index.
* add signature information when help on one command
* tell user that one command support operated on cell paths
Also, make type output to be more friendly, like `record<>` should just be `record`
And the same to `table<>`, which should be `table`
* simplify code
* don't show signatures for parser keyword
* update comment
* output arg syntax shape as type, so it's the same as describe command
* fix string when no positional args
* update signature body
* update
* add help signature test
* fix arg output format for composed data type like list or record
* fix clippy
* add comment
* New "display_output" hook.
* Fix unrelated "clippy" complaint in nu-tables crate.
* Fix code-formattng and style issues in "display_output" hook
* Enhance eval_hook to return PipelineData.
This allows a hook (including display_output) to return a value.
Co-authored-by: JT <547158+jntrnr@users.noreply.github.com>
* Remove unnecessary `#[allow]` annots
Reduce the number of lint exceptions that are not necessary with the
current state of the code (or more recent toolchain)
* Remove dead code from `FileStructure` in nu-command
* Replace `allow(unused)` with relevant feature switch
* Deal with `needless_collect` with annotations
* Change hack for needless_collect in `from json`
This change obviates the need for `allow(needless_collect)`
Removes a pessimistic allocation for empty strings, but increases
allocation size to `Value`
Probably not really worth it.
* Revert "Deal with `needless_collect` with annotations"
This reverts commit 05aca98445.
The previous state seems to better from a performance perspective as a
`Vec<String>` is lighter weight than `Vec<Value>`
* Revert "Revert "Try again: in unix like system, set foreground process while running external command (#6273)" (#6542)"
This reverts commit 2bb367f570.
* Make foreground job control hopefully work correctly
These changes are mostly inspired by the glibc manual.
* Fix typo in external command description
* Only restore tty control to shell when no fg procs are left; reuse pgrp
* Rework terminal acquirement code to be like fish
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
* remove export_env command
* remove several export env usage in test code
* adjust hiding relative test case
* fix clippy
* adjust tests
* update tests
* unignore these tests to expose ut failed
* using `use` instead of `overlay use` in some tests
* Revert "using `use` instead of `overlay use` in some tests"
This reverts commit 2ae24b24c3.
* Revert "adjust hiding relative test case"
This reverts commit 4369af6d05.
* Bring back module example
* Revert "update tests"
This reverts commit 6ae94ef513.
* Fix tests
* "Fix" a test
* Remove remaining deprecated env functionality
* Re-enable environment hiding for `hide`
To not break virtualenv since the overlay update is not merged yet
* Fix hiding env in `hide` and ignore some tests
Co-authored-by: kubouch <kubouch@gmail.com>
* Copy lev_distance.rs from the rust compiler
* Minor changes to code from rust compiler
* "Did you mean" suggestions: test instrumented to generate markdown report
* Did you mean suggestions: delete test instrumentation
* Fix tests
* Fix test
`foo` has a genuine match: `for`
* Improve tests
* Initialize join.rs as a copy of collect.rs
* Evolve StrCollect into StrJoin
* Replace 'str collect' with 'str join' everywhere
git ls-files | lines | par-each { |it| sed -i 's,str collect,str join,g' $it }
* Deprecate 'str collect'
* Revert "Deprecate 'str collect'"
This reverts commit 959d14203e.
* Change `str collect` help message to say that it is deprecated
We cannot remove `str collect` currently (i.e. via
`nu_protocol::ShellError::DeprecatedCommand` since a prominent project
uses the API:
b85542c31c/src/virtualenv/activation/nushell/activate.nu (L43)
Rename `all?`, `any?` and `empty?` to `all`, `any` and `is-empty` for sake of simplicity and consistency.
- More understandable for newcomers, that these commands are no special to others.
- `?` syntax did not really aprove readability. For me it made it worse.
- We can reserve `?` syntax for any other nushell feature.
* Add test cases for $nu.config-path change
Part of #6366
Signed-off-by: nibon7 <nibon7@163.com>
* do not start a new process to test default config path
Signed-off-by: nibon7 <nibon7@163.com>
Signed-off-by: nibon7 <nibon7@163.com>
* Revert "Fix intermittent test crash (#6268)"
This reverts commit 555d9ee763.
* make a working version again
* try second impl
* add
* fmt
* check stdin is atty before acquire stdin
* add libc
* clean comment
* fix typo
* Add hide-env to hide env vars; Cleanup tests
Also, there were some old unalias tests that I converted to hide.
* Add missing file
* Re-enable hide for env vars
* Fix test
* Rename did you mean error back
It was causing random tests to break