forked from extern/nushell
# Description Closes #6909. You can now add closures to your `color_config` themes. Whenever a value would be printed with `table`, the closure is run with the value piped-in. The closure must return either a {fg,bg,attr} record or a color name (`'light_red'` etc.). This returned style is used to colour the value. This is entirely backwards-compatible with existing config.nu files. Example code excerpt: ``` let my_theme = { header: green_bold bool: { if $in { 'light_cyan' } else { 'light_red' } } int: purple_bold filesize: { |e| if $e == 0b { 'gray' } else if $e < 1mb { 'purple_bold' } else { 'cyan_bold' } } duration: purple_bold date: { (date now) - $in | if $in > 1wk { 'cyan_bold' } else if $in > 1day { 'green_bold' } else { 'yellow_bold' } } range: yellow_bold string: { if $in =~ '^#\w{6}$' { $in } else { 'white' } } nothing: white ``` Example output with this in effect: 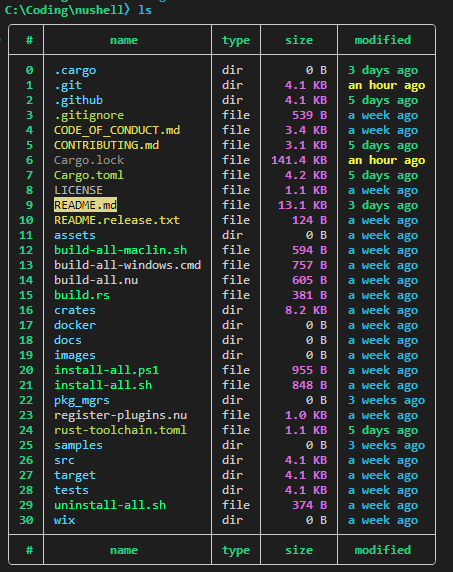 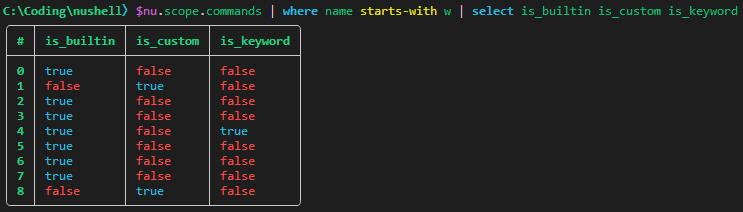 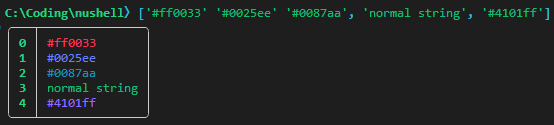 Slightly important notes: * Some color_config names, namely "separator", "empty" and "hints", pipe in `null` instead of a value. * Currently, doing anything non-trivial inside a closure has an understandably big perf hit. I currently do not actually recommend something like `string: { if $in =~ '^#\w{6}$' { $in } else { 'white' } }` for serious work, mainly because of the abundance of string-type data in the world. Nevertheless, lesser-used types like "date" and "duration" work well with this. * I had to do some reorganisation in order to make it possible to call `eval_block()` that late in table rendering. I invented a new struct called "StyleComputer" which holds the engine_state and stack of the initial `table` command (implicit or explicit). * StyleComputer has a `compute()` method which takes a color_config name and a nu value, and always returns the correct Style, so you don't have to worry about A) the color_config value was set at all, B) whether it was set to a closure or not, or C) which default style to use in those cases. * Currently, errors encountered during execution of the closures are thrown in the garbage. Any other ideas are welcome. (Nonetheless, errors result in a huge perf hit when they are encountered. I think what should be done is to assume something terrible happened to the user's config and invalidate the StyleComputer for that `table` run, thus causing subsequent output to just be Style::default().) * More thorough tests are forthcoming - ran into some difficulty using `nu!` to take an alternative config, and for some reason `let-env config =` statements don't seem to work inside `nu!` pipelines(???) * The default config.nu has not been updated to make use of this yet. Do tell if you think I should incorporate that into this. # User-Facing Changes See above. # Tests + Formatting Don't forget to add tests that cover your changes. Make sure you've run and fixed any issues with these commands: - `cargo fmt --all -- --check` to check standard code formatting (`cargo fmt --all` applies these changes) - `cargo clippy --workspace --features=extra -- -D warnings -D clippy::unwrap_used -A clippy::needless_collect` to check that you're using the standard code style - `cargo test --workspace --features=extra` to check that all tests pass # After Submitting If your PR had any user-facing changes, update [the documentation](https://github.com/nushell/nushell.github.io) after the PR is merged, if necessary. This will help us keep the docs up to date.
498 lines
15 KiB
Rust
498 lines
15 KiB
Rust
mod common;
|
|
|
|
use common::{create_row as row, VecCells};
|
|
use nu_table::{TableConfig, TableTheme as theme};
|
|
|
|
#[test]
|
|
fn test_rounded() {
|
|
assert_eq!(
|
|
create_table(vec![row(4); 3], true, theme::rounded()),
|
|
"╭───┬───┬───┬───╮\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
├───┼───┼───┼───┤\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
╰───┴───┴───┴───╯"
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 2], true, theme::rounded()),
|
|
"╭───┬───┬───┬───╮\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
├───┼───┼───┼───┤\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
╰───┴───┴───┴───╯"
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 1], true, theme::rounded()),
|
|
"╭───┬───┬───┬───╮\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
╰───┴───┴───┴───╯"
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 1], false, theme::rounded()),
|
|
"╭───┬───┬───┬───╮\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
╰───┴───┴───┴───╯"
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 2], false, theme::rounded()),
|
|
"╭───┬───┬───┬───╮\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
╰───┴───┴───┴───╯"
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table_with_size(vec![row(4); 0], (0, 4), true, theme::rounded()),
|
|
""
|
|
);
|
|
}
|
|
|
|
#[test]
|
|
fn test_basic() {
|
|
assert_eq!(
|
|
create_table(vec![row(4); 3], true, theme::basic()),
|
|
"+---+---+---+---+\n\
|
|
| 0 | 1 | 2 | 3 |\n\
|
|
+---+---+---+---+\n\
|
|
| 0 | 1 | 2 | 3 |\n\
|
|
+---+---+---+---+\n\
|
|
| 0 | 1 | 2 | 3 |\n\
|
|
+---+---+---+---+"
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 2], true, theme::basic()),
|
|
"+---+---+---+---+\n\
|
|
| 0 | 1 | 2 | 3 |\n\
|
|
+---+---+---+---+\n\
|
|
| 0 | 1 | 2 | 3 |\n\
|
|
+---+---+---+---+"
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 1], true, theme::basic()),
|
|
"+---+---+---+---+\n\
|
|
| 0 | 1 | 2 | 3 |\n\
|
|
+---+---+---+---+"
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 1], false, theme::basic()),
|
|
"+---+---+---+---+\n\
|
|
| 0 | 1 | 2 | 3 |\n\
|
|
+---+---+---+---+"
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 2], false, theme::basic()),
|
|
"+---+---+---+---+\n\
|
|
| 0 | 1 | 2 | 3 |\n\
|
|
+---+---+---+---+\n\
|
|
| 0 | 1 | 2 | 3 |\n\
|
|
+---+---+---+---+"
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table_with_size(vec![row(4); 0], (0, 4), true, theme::basic()),
|
|
""
|
|
);
|
|
}
|
|
|
|
#[test]
|
|
fn test_reinforced() {
|
|
assert_eq!(
|
|
create_table(vec![row(4); 3], true, theme::reinforced()),
|
|
"┏───┬───┬───┬───┓\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
┗───┴───┴───┴───┛"
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 2], true, theme::reinforced()),
|
|
"┏───┬───┬───┬───┓\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
┗───┴───┴───┴───┛"
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 1], true, theme::reinforced()),
|
|
"┏───┬───┬───┬───┓\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
┗───┴───┴───┴───┛"
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 1], false, theme::reinforced()),
|
|
"┏───┬───┬───┬───┓\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
┗───┴───┴───┴───┛"
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 2], false, theme::reinforced()),
|
|
"┏───┬───┬───┬───┓\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
┗───┴───┴───┴───┛"
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table_with_size(vec![row(4); 0], (0, 4), true, theme::reinforced()),
|
|
""
|
|
);
|
|
}
|
|
|
|
#[test]
|
|
fn test_compact() {
|
|
assert_eq!(
|
|
create_table(vec![row(4); 3], true, theme::compact()),
|
|
concat!(
|
|
"───┬───┬───┬───\n",
|
|
" 0 │ 1 │ 2 │ 3 \n",
|
|
"───┼───┼───┼───\n",
|
|
" 0 │ 1 │ 2 │ 3 \n",
|
|
" 0 │ 1 │ 2 │ 3 \n",
|
|
"───┴───┴───┴───",
|
|
)
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 2], true, theme::compact()),
|
|
concat!(
|
|
"───┬───┬───┬───\n",
|
|
" 0 │ 1 │ 2 │ 3 \n",
|
|
"───┼───┼───┼───\n",
|
|
" 0 │ 1 │ 2 │ 3 \n",
|
|
"───┴───┴───┴───",
|
|
)
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 1], true, theme::compact()),
|
|
concat!("───┬───┬───┬───\n", " 0 │ 1 │ 2 │ 3 \n", "───┴───┴───┴───",)
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 1], false, theme::compact()),
|
|
concat!("───┬───┬───┬───\n", " 0 │ 1 │ 2 │ 3 \n", "───┴───┴───┴───",)
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 2], false, theme::compact()),
|
|
concat!(
|
|
"───┬───┬───┬───\n",
|
|
" 0 │ 1 │ 2 │ 3 \n",
|
|
" 0 │ 1 │ 2 │ 3 \n",
|
|
"───┴───┴───┴───",
|
|
)
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table_with_size(vec![row(4); 0], (0, 4), true, theme::compact()),
|
|
""
|
|
);
|
|
}
|
|
|
|
#[test]
|
|
fn test_compact_double() {
|
|
assert_eq!(
|
|
create_table(vec![row(4); 3], true, theme::compact_double()),
|
|
concat!(
|
|
"═══╦═══╦═══╦═══\n",
|
|
" 0 ║ 1 ║ 2 ║ 3 \n",
|
|
"═══╬═══╬═══╬═══\n",
|
|
" 0 ║ 1 ║ 2 ║ 3 \n",
|
|
" 0 ║ 1 ║ 2 ║ 3 \n",
|
|
"═══╩═══╩═══╩═══",
|
|
)
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 2], true, theme::compact_double()),
|
|
concat!(
|
|
"═══╦═══╦═══╦═══\n",
|
|
" 0 ║ 1 ║ 2 ║ 3 \n",
|
|
"═══╬═══╬═══╬═══\n",
|
|
" 0 ║ 1 ║ 2 ║ 3 \n",
|
|
"═══╩═══╩═══╩═══",
|
|
)
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 1], true, theme::compact_double()),
|
|
concat!("═══╦═══╦═══╦═══\n", " 0 ║ 1 ║ 2 ║ 3 \n", "═══╩═══╩═══╩═══",)
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 1], false, theme::compact_double()),
|
|
concat!("═══╦═══╦═══╦═══\n", " 0 ║ 1 ║ 2 ║ 3 \n", "═══╩═══╩═══╩═══",)
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 2], false, theme::compact_double()),
|
|
concat!(
|
|
"═══╦═══╦═══╦═══\n",
|
|
" 0 ║ 1 ║ 2 ║ 3 \n",
|
|
" 0 ║ 1 ║ 2 ║ 3 \n",
|
|
"═══╩═══╩═══╩═══",
|
|
)
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table_with_size(vec![row(4); 0], (0, 4), true, theme::compact_double()),
|
|
""
|
|
);
|
|
}
|
|
|
|
#[test]
|
|
fn test_heavy() {
|
|
assert_eq!(
|
|
create_table(vec![row(4); 3], true, theme::heavy()),
|
|
"┏━━━┳━━━┳━━━┳━━━┓\n\
|
|
┃ 0 ┃ 1 ┃ 2 ┃ 3 ┃\n\
|
|
┣━━━╋━━━╋━━━╋━━━┫\n\
|
|
┃ 0 ┃ 1 ┃ 2 ┃ 3 ┃\n\
|
|
┃ 0 ┃ 1 ┃ 2 ┃ 3 ┃\n\
|
|
┗━━━┻━━━┻━━━┻━━━┛"
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 2], true, theme::heavy()),
|
|
"┏━━━┳━━━┳━━━┳━━━┓\n\
|
|
┃ 0 ┃ 1 ┃ 2 ┃ 3 ┃\n\
|
|
┣━━━╋━━━╋━━━╋━━━┫\n\
|
|
┃ 0 ┃ 1 ┃ 2 ┃ 3 ┃\n\
|
|
┗━━━┻━━━┻━━━┻━━━┛"
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 1], true, theme::heavy()),
|
|
"┏━━━┳━━━┳━━━┳━━━┓\n\
|
|
┃ 0 ┃ 1 ┃ 2 ┃ 3 ┃\n\
|
|
┗━━━┻━━━┻━━━┻━━━┛"
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 1], false, theme::heavy()),
|
|
"┏━━━┳━━━┳━━━┳━━━┓\n\
|
|
┃ 0 ┃ 1 ┃ 2 ┃ 3 ┃\n\
|
|
┗━━━┻━━━┻━━━┻━━━┛"
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 2], false, theme::heavy()),
|
|
"┏━━━┳━━━┳━━━┳━━━┓\n\
|
|
┃ 0 ┃ 1 ┃ 2 ┃ 3 ┃\n\
|
|
┃ 0 ┃ 1 ┃ 2 ┃ 3 ┃\n\
|
|
┗━━━┻━━━┻━━━┻━━━┛"
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table_with_size(vec![row(4); 0], (0, 4), true, theme::heavy()),
|
|
""
|
|
);
|
|
}
|
|
|
|
#[test]
|
|
fn test_light() {
|
|
assert_eq!(
|
|
create_table(vec![row(4); 3], true, theme::light()),
|
|
concat!(
|
|
" 0 1 2 3 \n",
|
|
"───────────────\n",
|
|
" 0 1 2 3 \n",
|
|
" 0 1 2 3 ",
|
|
)
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 2], true, theme::light()),
|
|
concat!(" 0 1 2 3 \n", "───────────────\n", " 0 1 2 3 ")
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 1], true, theme::light()),
|
|
concat!(" 0 1 2 3 ")
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 1], false, theme::light()),
|
|
concat!(" 0 1 2 3 ")
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 2], false, theme::light()),
|
|
concat!(" 0 1 2 3 \n", " 0 1 2 3 ")
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table_with_size(vec![row(4); 0], (0, 4), true, theme::light()),
|
|
""
|
|
);
|
|
}
|
|
|
|
#[test]
|
|
fn test_none() {
|
|
assert_eq!(
|
|
create_table(vec![row(4); 3], true, theme::none()),
|
|
concat!(" 0 1 2 3 \n", " 0 1 2 3 \n", " 0 1 2 3 ")
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 2], true, theme::none()),
|
|
concat!(" 0 1 2 3 \n", " 0 1 2 3 ")
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 1], true, theme::none()),
|
|
concat!(" 0 1 2 3 ")
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 1], false, theme::none()),
|
|
concat!(" 0 1 2 3 ")
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 2], true, theme::none()),
|
|
concat!(" 0 1 2 3 \n", " 0 1 2 3 ")
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table_with_size(vec![row(4); 0], (0, 4), true, theme::none()),
|
|
""
|
|
);
|
|
}
|
|
|
|
#[test]
|
|
fn test_thin() {
|
|
assert_eq!(
|
|
create_table(vec![row(4); 3], true, theme::thin()),
|
|
"┌───┬───┬───┬───┐\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
├───┼───┼───┼───┤\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
├───┼───┼───┼───┤\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
└───┴───┴───┴───┘"
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 2], true, theme::thin()),
|
|
"┌───┬───┬───┬───┐\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
├───┼───┼───┼───┤\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
└───┴───┴───┴───┘"
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 1], true, theme::thin()),
|
|
"┌───┬───┬───┬───┐\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
└───┴───┴───┴───┘"
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 1], false, theme::thin()),
|
|
"┌───┬───┬───┬───┐\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
└───┴───┴───┴───┘"
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 2], false, theme::thin()),
|
|
"┌───┬───┬───┬───┐\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
├───┼───┼───┼───┤\n\
|
|
│ 0 │ 1 │ 2 │ 3 │\n\
|
|
└───┴───┴───┴───┘"
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table_with_size(vec![row(4); 0], (0, 4), true, theme::thin()),
|
|
""
|
|
);
|
|
}
|
|
|
|
#[test]
|
|
fn test_with_love() {
|
|
assert_eq!(
|
|
create_table(vec![row(4); 3], true, theme::with_love()),
|
|
concat!(
|
|
"❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤\n",
|
|
" 0 ❤ 1 ❤ 2 ❤ 3 \n",
|
|
"❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤\n",
|
|
" 0 ❤ 1 ❤ 2 ❤ 3 \n",
|
|
" 0 ❤ 1 ❤ 2 ❤ 3 \n",
|
|
"❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤",
|
|
)
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 2], true, theme::with_love()),
|
|
concat!(
|
|
"❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤\n",
|
|
" 0 ❤ 1 ❤ 2 ❤ 3 \n",
|
|
"❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤\n",
|
|
" 0 ❤ 1 ❤ 2 ❤ 3 \n",
|
|
"❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤",
|
|
)
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 1], true, theme::with_love()),
|
|
concat!("❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤\n", " 0 ❤ 1 ❤ 2 ❤ 3 \n", "❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤",)
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 1], false, theme::with_love()),
|
|
concat!("❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤\n", " 0 ❤ 1 ❤ 2 ❤ 3 \n", "❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤",)
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table(vec![row(4); 2], false, theme::with_love()),
|
|
concat!(
|
|
"❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤\n",
|
|
" 0 ❤ 1 ❤ 2 ❤ 3 \n",
|
|
" 0 ❤ 1 ❤ 2 ❤ 3 \n",
|
|
"❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤",
|
|
)
|
|
);
|
|
|
|
assert_eq!(
|
|
create_table_with_size(vec![row(4); 0], (0, 4), true, theme::with_love()),
|
|
""
|
|
);
|
|
}
|
|
|
|
fn create_table(data: VecCells, with_header: bool, theme: theme) -> String {
|
|
let config = TableConfig::new(theme, with_header, false, false);
|
|
let out = common::create_table(data, config, usize::MAX);
|
|
|
|
out.expect("not expected to get None")
|
|
}
|
|
|
|
fn create_table_with_size(
|
|
data: VecCells,
|
|
size: (usize, usize),
|
|
with_header: bool,
|
|
theme: theme,
|
|
) -> String {
|
|
let config = TableConfig::new(theme, with_header, false, false);
|
|
|
|
let table = nu_table::Table::new(data, size);
|
|
|
|
table
|
|
.draw(config, usize::MAX)
|
|
.expect("not expected to get None")
|
|
}
|