forked from extern/nushell
# Description Add `xaccess`,`xupdate` and `xinsert` scripts to standard library. They allow accessing and manipulating data in new xml format https://github.com/nushell/nushell/pull/7947 with relative ease. Access some data in nushell xml structure: 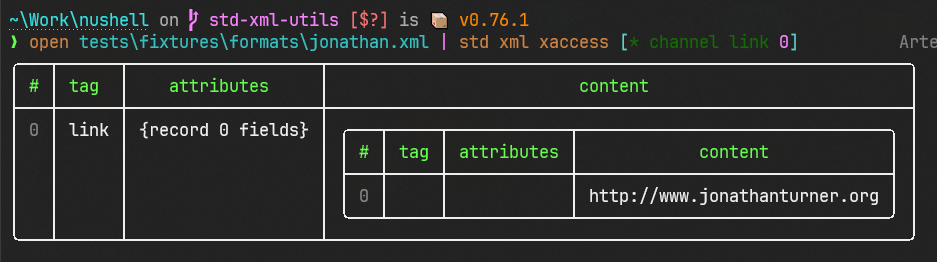 Update attributes of xml tags matching a path: 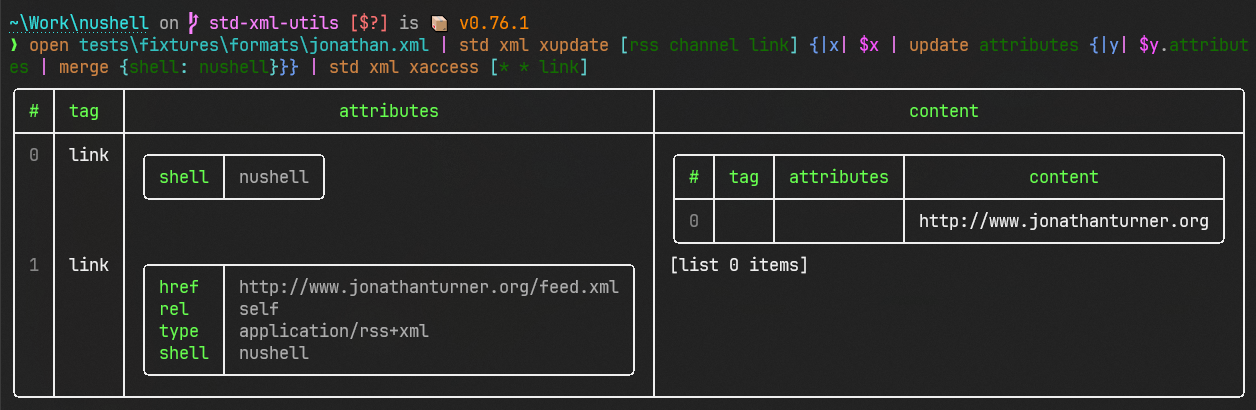 # User-Facing Changes New commands `std xaccess`, `std xupdate` and `std xinsert` # Tests + Formatting Don't forget to add tests that cover your changes. Make sure you've run and fixed any issues with these commands: - `cargo fmt --all -- --check` to check standard code formatting (`cargo fmt --all` applies these changes) - `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A clippy::needless_collect` to check that you're using the standard code style - `cargo test --workspace` to check that all tests pass > **Note** > from `nushell` you can also use the `toolkit` as follows > ```bash > use toolkit.nu # or use an `env_change` hook to activate it automatically > toolkit check pr > ``` # After Submitting If your PR had any user-facing changes, update [the documentation](https://github.com/nushell/nushell.github.io) after the PR is merged, if necessary. This will help us keep the docs up to date.
30 lines
2.2 KiB
Plaintext
30 lines
2.2 KiB
Plaintext
use std.nu "xaccess"
|
|
use std.nu "xupdate"
|
|
use std.nu "xinsert"
|
|
use std.nu "assert equal"
|
|
|
|
export def test_xml_xaccess [] {
|
|
let sample_xml = ('<a><b><c a="b"></c></b><c></c><d><e>z</e><e>x</e></d></a>' | from xml)
|
|
|
|
assert equal ($sample_xml | xaccess [a]) [$sample_xml]
|
|
assert equal ($sample_xml | xaccess [*]) [$sample_xml]
|
|
assert equal ($sample_xml | xaccess [* d e]) [[tag, attributes, content]; [e, {}, [[tag, attributes, content]; [null, null, z]]], [e, {}, [[tag, attributes, content]; [null, null, x]]]]
|
|
assert equal ($sample_xml | xaccess [* d e 1]) [[tag, attributes, content]; [e, {}, [[tag, attributes, content]; [null, null, x]]]]
|
|
assert equal ($sample_xml | xaccess [* * * {|e| $e.attributes != {}}]) [[tag, attributes, content]; [c, {a: b}, []]]
|
|
}
|
|
|
|
export def test_xml_xupdate [] {
|
|
let sample_xml = ('<a><b><c a="b"></c></b><c></c><d><e>z</e><e>x</e></d></a>' | from xml)
|
|
|
|
assert equal ($sample_xml | xupdate [*] {|x| $x | update attributes {i: j}}) ('<a i="j"><b><c a="b"></c></b><c></c><d><e>z</e><e>x</e></d></a>' | from xml)
|
|
assert equal ($sample_xml | xupdate [* d e *] {|x| $x | update content 'nushell'}) ('<a><b><c a="b"></c></b><c></c><d><e>nushell</e><e>nushell</e></d></a>' | from xml)
|
|
assert equal ($sample_xml | xupdate [* * * {|e| $e.attributes != {}}] {|x| $x | update content ['xml']}) {tag: a, attributes: {}, content: [[tag, attributes, content]; [b, {}, [[tag, attributes, content]; [c, {a: b}, [xml]]]], [c, {}, []], [d, {}, [[tag, attributes, content]; [e, {}, [[tag, attributes, content]; [null, null, z]]], [e, {}, [[tag, attributes, content]; [null, null, x]]]]]]}
|
|
}
|
|
|
|
export def test_xml_xinsert [] {
|
|
let sample_xml = ('<a><b><c a="b"></c></b><c></c><d><e>z</e><e>x</e></d></a>' | from xml)
|
|
|
|
assert equal ($sample_xml | xinsert [a] {tag: b attributes:{} content: []}) ('<a><b><c a="b"></c></b><c></c><d><e>z</e><e>x</e></d><b></b></a>' | from xml)
|
|
assert equal ($sample_xml | xinsert [a d *] {tag: null attributes: null content: 'n'} | to xml) '<a><b><c a="b"></c></b><c></c><d><e>zn</e><e>xn</e></d></a>'
|
|
assert equal ($sample_xml | xinsert [a *] {tag: null attributes: null content: 'n'}) ('<a><b><c a="b"></c>n</b><c>n</c><d><e>z</e><e>x</e>n</d></a>' | from xml)
|
|
} |