2021-11-26 09:00:57 +01:00
|
|
|
use nu_protocol::engine::{EngineState, StateWorkingSet};
|
2021-09-03 00:58:15 +02:00
|
|
|
|
2021-10-02 04:59:11 +02:00
|
|
|
use crate::*;
|
2021-09-03 00:58:15 +02:00
|
|
|
|
2022-07-14 16:09:27 +02:00
|
|
|
pub fn create_default_context() -> EngineState {
|
2021-10-25 08:31:39 +02:00
|
|
|
let mut engine_state = EngineState::new();
|
2021-10-28 06:13:10 +02:00
|
|
|
|
2021-09-03 00:58:15 +02:00
|
|
|
let delta = {
|
2021-10-25 08:31:39 +02:00
|
|
|
let mut working_set = StateWorkingSet::new(&engine_state);
|
2021-09-03 00:58:15 +02:00
|
|
|
|
2021-10-10 06:13:15 +02:00
|
|
|
macro_rules! bind_command {
|
2021-12-11 00:14:28 +01:00
|
|
|
( $( $command:expr ),* $(,)? ) => {
|
2021-10-10 06:13:15 +02:00
|
|
|
$( working_set.add_decl(Box::new($command)); )*
|
|
|
|
};
|
|
|
|
}
|
2021-09-23 21:03:08 +02:00
|
|
|
|
2021-11-28 20:35:02 +01:00
|
|
|
// If there are commands that have the same name as default declarations,
|
|
|
|
// they have to be registered before the main declarations. This helps to make
|
|
|
|
// them only accessible if the correct input value category is used with the
|
|
|
|
// declaration
|
|
|
|
#[cfg(feature = "dataframe")]
|
2021-12-06 05:09:49 +01:00
|
|
|
add_dataframe_decls(&mut working_set);
|
2021-11-28 20:35:02 +01:00
|
|
|
|
2022-04-24 11:29:21 +02:00
|
|
|
// Database-related
|
|
|
|
// Adds all related commands to query databases
|
2022-11-23 01:58:11 +01:00
|
|
|
#[cfg(feature = "sqlite")]
|
2022-04-24 11:29:21 +02:00
|
|
|
add_database_decls(&mut working_set);
|
|
|
|
|
2021-12-11 04:07:39 +01:00
|
|
|
// Core
|
|
|
|
bind_command! {
|
2021-10-10 22:24:54 +02:00
|
|
|
Alias,
|
2022-08-26 21:48:48 +02:00
|
|
|
Ast,
|
New commands: `break`, `continue`, `return`, and `loop` (#7230)
# Description
This adds `break`, `continue`, `return`, and `loop`.
* `break` - breaks out a loop
* `continue` - continues a loop at the next iteration
* `return` - early return from a function call
* `loop` - loop forever (until the loop hits a break)
Examples:
```
for i in 1..10 {
if $i == 5 {
continue
}
print $i
}
```
```
for i in 1..10 {
if $i == 5 {
break
}
print $i
}
```
```
def foo [x] {
if true {
return 2
}
$x
}
foo 100
```
```
loop { print "hello, forever" }
```
```
[1, 2, 3, 4, 5] | each {|x|
if $x > 3 { break }
$x
}
```
# User-Facing Changes
Adds the above commands.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
2022-11-24 21:39:16 +01:00
|
|
|
Break,
|
2022-09-09 22:31:32 +02:00
|
|
|
Commandline,
|
2022-12-21 23:21:03 +01:00
|
|
|
Const,
|
New commands: `break`, `continue`, `return`, and `loop` (#7230)
# Description
This adds `break`, `continue`, `return`, and `loop`.
* `break` - breaks out a loop
* `continue` - continues a loop at the next iteration
* `return` - early return from a function call
* `loop` - loop forever (until the loop hits a break)
Examples:
```
for i in 1..10 {
if $i == 5 {
continue
}
print $i
}
```
```
for i in 1..10 {
if $i == 5 {
break
}
print $i
}
```
```
def foo [x] {
if true {
return 2
}
$x
}
foo 100
```
```
loop { print "hello, forever" }
```
```
[1, 2, 3, 4, 5] | each {|x|
if $x > 3 { break }
$x
}
```
# User-Facing Changes
Adds the above commands.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
2022-11-24 21:39:16 +01:00
|
|
|
Continue,
|
2021-11-19 06:00:29 +01:00
|
|
|
Debug,
|
2021-10-10 22:24:54 +02:00
|
|
|
Def,
|
2022-01-29 21:45:46 +01:00
|
|
|
DefEnv,
|
2021-11-19 06:00:29 +01:00
|
|
|
Describe,
|
2021-10-10 22:24:54 +02:00
|
|
|
Do,
|
2021-11-01 09:12:48 +01:00
|
|
|
Echo,
|
2022-02-05 15:39:51 +01:00
|
|
|
ErrorMake,
|
2022-03-19 19:58:01 +01:00
|
|
|
ExportAlias,
|
2021-11-16 00:16:06 +01:00
|
|
|
ExportCommand,
|
2021-10-10 22:24:54 +02:00
|
|
|
ExportDef,
|
2022-01-29 21:45:46 +01:00
|
|
|
ExportDefEnv,
|
2022-03-19 19:58:01 +01:00
|
|
|
ExportExtern,
|
2022-07-29 10:57:10 +02:00
|
|
|
ExportUse,
|
2022-02-11 19:38:10 +01:00
|
|
|
Extern,
|
2021-10-10 22:24:54 +02:00
|
|
|
For,
|
|
|
|
Help,
|
2022-12-30 16:44:37 +01:00
|
|
|
HelpAliases,
|
|
|
|
HelpCommands,
|
|
|
|
HelpModules,
|
2022-11-27 09:03:17 +01:00
|
|
|
HelpOperators,
|
2021-10-10 22:24:54 +02:00
|
|
|
Hide,
|
2022-08-13 11:55:06 +02:00
|
|
|
HideEnv,
|
2021-10-10 22:24:54 +02:00
|
|
|
If,
|
2021-12-30 05:54:33 +01:00
|
|
|
Ignore,
|
2022-05-07 21:39:22 +02:00
|
|
|
Overlay,
|
2022-08-21 16:27:56 +02:00
|
|
|
OverlayUse,
|
2022-05-07 21:39:22 +02:00
|
|
|
OverlayList,
|
2022-05-26 16:47:04 +02:00
|
|
|
OverlayNew,
|
2022-08-21 16:27:56 +02:00
|
|
|
OverlayHide,
|
2021-12-11 04:07:39 +01:00
|
|
|
Let,
|
New commands: `break`, `continue`, `return`, and `loop` (#7230)
# Description
This adds `break`, `continue`, `return`, and `loop`.
* `break` - breaks out a loop
* `continue` - continues a loop at the next iteration
* `return` - early return from a function call
* `loop` - loop forever (until the loop hits a break)
Examples:
```
for i in 1..10 {
if $i == 5 {
continue
}
print $i
}
```
```
for i in 1..10 {
if $i == 5 {
break
}
print $i
}
```
```
def foo [x] {
if true {
return 2
}
$x
}
foo 100
```
```
loop { print "hello, forever" }
```
```
[1, 2, 3, 4, 5] | each {|x|
if $x > 3 { break }
$x
}
```
# User-Facing Changes
Adds the above commands.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
2022-11-24 21:39:16 +01:00
|
|
|
Loop,
|
2021-12-24 01:16:50 +01:00
|
|
|
Metadata,
|
2021-12-11 04:07:39 +01:00
|
|
|
Module,
|
2022-11-11 07:51:08 +01:00
|
|
|
Mut,
|
New commands: `break`, `continue`, `return`, and `loop` (#7230)
# Description
This adds `break`, `continue`, `return`, and `loop`.
* `break` - breaks out a loop
* `continue` - continues a loop at the next iteration
* `return` - early return from a function call
* `loop` - loop forever (until the loop hits a break)
Examples:
```
for i in 1..10 {
if $i == 5 {
continue
}
print $i
}
```
```
for i in 1..10 {
if $i == 5 {
break
}
print $i
}
```
```
def foo [x] {
if true {
return 2
}
$x
}
foo 100
```
```
loop { print "hello, forever" }
```
```
[1, 2, 3, 4, 5] | each {|x|
if $x > 3 { break }
$x
}
```
# User-Facing Changes
Adds the above commands.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
2022-11-24 21:39:16 +01:00
|
|
|
Return,
|
2022-11-24 05:52:11 +01:00
|
|
|
Try,
|
2021-12-11 04:07:39 +01:00
|
|
|
Use,
|
2021-12-11 21:08:17 +01:00
|
|
|
Version,
|
2022-11-11 19:21:45 +01:00
|
|
|
While,
|
2021-12-11 04:07:39 +01:00
|
|
|
};
|
|
|
|
|
2022-05-13 13:48:47 +02:00
|
|
|
// Charts
|
|
|
|
bind_command! {
|
|
|
|
Histogram
|
|
|
|
}
|
|
|
|
|
2021-12-11 04:07:39 +01:00
|
|
|
// Filters
|
|
|
|
bind_command! {
|
|
|
|
All,
|
|
|
|
Any,
|
|
|
|
Append,
|
|
|
|
Collect,
|
2021-12-18 19:14:28 +01:00
|
|
|
Columns,
|
2021-12-23 04:08:39 +01:00
|
|
|
Compact,
|
2022-01-25 16:02:15 +01:00
|
|
|
Default,
|
2021-12-11 04:07:39 +01:00
|
|
|
Drop,
|
2021-12-14 20:54:27 +01:00
|
|
|
DropColumn,
|
2021-12-15 13:26:15 +01:00
|
|
|
DropNth,
|
2021-12-11 04:07:39 +01:00
|
|
|
Each,
|
2022-06-14 16:16:31 +02:00
|
|
|
EachWhile,
|
2021-12-19 20:11:57 +01:00
|
|
|
Empty,
|
2023-01-27 18:45:57 +01:00
|
|
|
Enumerate,
|
2021-12-31 00:41:18 +01:00
|
|
|
Every,
|
2022-12-10 18:23:24 +01:00
|
|
|
Filter,
|
2022-01-23 23:32:02 +01:00
|
|
|
Find,
|
2021-12-11 04:07:39 +01:00
|
|
|
First,
|
2021-12-17 21:44:51 +01:00
|
|
|
Flatten,
|
2021-12-11 04:07:39 +01:00
|
|
|
Get,
|
2022-03-07 02:01:29 +01:00
|
|
|
Group,
|
2022-01-21 21:28:21 +01:00
|
|
|
GroupBy,
|
2022-02-12 03:06:49 +01:00
|
|
|
Headers,
|
2022-03-17 18:55:02 +01:00
|
|
|
Insert,
|
2022-01-31 00:29:21 +01:00
|
|
|
SplitBy,
|
2022-04-07 22:49:28 +02:00
|
|
|
Take,
|
2022-01-22 00:50:26 +01:00
|
|
|
Merge,
|
2022-01-24 20:43:38 +01:00
|
|
|
Move,
|
2022-04-07 22:49:28 +02:00
|
|
|
TakeWhile,
|
|
|
|
TakeUntil,
|
2021-10-26 20:29:00 +02:00
|
|
|
Last,
|
2021-10-10 22:24:54 +02:00
|
|
|
Length,
|
|
|
|
Lines,
|
2021-10-26 03:30:53 +02:00
|
|
|
ParEach,
|
2021-12-07 09:46:21 +01:00
|
|
|
Prepend,
|
2021-10-30 19:51:20 +02:00
|
|
|
Range,
|
2022-01-08 01:40:40 +01:00
|
|
|
Reduce,
|
2021-12-05 04:09:45 +01:00
|
|
|
Reject,
|
2022-01-29 11:26:47 +01:00
|
|
|
Rename,
|
2021-11-07 19:18:27 +01:00
|
|
|
Reverse,
|
2022-02-12 12:11:54 +01:00
|
|
|
Roll,
|
|
|
|
RollDown,
|
|
|
|
RollUp,
|
|
|
|
RollLeft,
|
|
|
|
RollRight,
|
2022-01-29 21:47:28 +01:00
|
|
|
Rotate,
|
2021-10-10 22:24:54 +02:00
|
|
|
Select,
|
2021-11-07 02:19:57 +01:00
|
|
|
Shuffle,
|
2021-11-29 07:52:23 +01:00
|
|
|
Skip,
|
|
|
|
SkipUntil,
|
|
|
|
SkipWhile,
|
2022-04-01 00:43:16 +02:00
|
|
|
Sort,
|
2022-01-22 21:49:50 +01:00
|
|
|
SortBy,
|
2022-07-16 13:24:37 +02:00
|
|
|
SplitList,
|
2022-01-21 21:28:21 +01:00
|
|
|
Transpose,
|
2021-12-11 04:07:39 +01:00
|
|
|
Uniq,
|
2022-12-02 11:36:01 +01:00
|
|
|
UniqBy,
|
2022-03-17 01:13:34 +01:00
|
|
|
Upsert,
|
2022-03-17 18:55:02 +01:00
|
|
|
Update,
|
2022-01-30 22:41:05 +01:00
|
|
|
UpdateCells,
|
2022-12-23 19:49:19 +01:00
|
|
|
Values,
|
2021-12-11 04:07:39 +01:00
|
|
|
Where,
|
2022-03-07 02:01:29 +01:00
|
|
|
Window,
|
2021-12-11 04:07:39 +01:00
|
|
|
Wrap,
|
|
|
|
Zip,
|
|
|
|
};
|
|
|
|
|
2022-06-16 18:58:38 +02:00
|
|
|
// Misc
|
|
|
|
bind_command! {
|
|
|
|
History,
|
|
|
|
Tutor,
|
2022-09-19 21:30:04 +02:00
|
|
|
HistorySession,
|
2022-06-16 18:58:38 +02:00
|
|
|
};
|
|
|
|
|
2021-12-13 02:47:14 +01:00
|
|
|
// Path
|
|
|
|
bind_command! {
|
|
|
|
Path,
|
|
|
|
PathBasename,
|
|
|
|
PathDirname,
|
|
|
|
PathExists,
|
|
|
|
PathExpand,
|
|
|
|
PathJoin,
|
|
|
|
PathParse,
|
|
|
|
PathRelativeTo,
|
|
|
|
PathSplit,
|
|
|
|
PathType,
|
|
|
|
};
|
|
|
|
|
2021-12-11 04:07:39 +01:00
|
|
|
// System
|
|
|
|
bind_command! {
|
|
|
|
Benchmark,
|
2022-02-25 20:51:31 +01:00
|
|
|
Complete,
|
2023-02-09 20:59:38 +01:00
|
|
|
Explain,
|
2021-12-11 04:07:39 +01:00
|
|
|
External,
|
2022-06-26 13:53:06 +02:00
|
|
|
NuCheck,
|
2021-12-11 04:07:39 +01:00
|
|
|
Sys,
|
|
|
|
};
|
|
|
|
|
2022-10-07 20:54:36 +02:00
|
|
|
#[cfg(unix)]
|
|
|
|
bind_command! { Exec }
|
|
|
|
|
|
|
|
#[cfg(windows)]
|
|
|
|
bind_command! { RegistryQuery }
|
|
|
|
|
2022-09-01 02:34:26 +02:00
|
|
|
#[cfg(any(
|
|
|
|
target_os = "android",
|
|
|
|
target_os = "linux",
|
|
|
|
target_os = "macos",
|
|
|
|
target_os = "windows"
|
|
|
|
))]
|
|
|
|
bind_command! { Ps };
|
|
|
|
|
2022-03-29 13:10:43 +02:00
|
|
|
#[cfg(feature = "which-support")]
|
2022-01-20 19:02:53 +01:00
|
|
|
bind_command! { Which };
|
|
|
|
|
2021-12-11 04:07:39 +01:00
|
|
|
// Strings
|
|
|
|
bind_command! {
|
2021-12-16 00:08:12 +01:00
|
|
|
Char,
|
2021-12-24 08:22:11 +01:00
|
|
|
Decode,
|
2022-06-25 23:35:23 +02:00
|
|
|
Encode,
|
|
|
|
DecodeBase64,
|
|
|
|
EncodeBase64,
|
2022-01-30 13:52:24 +01:00
|
|
|
DetectColumns,
|
2021-12-11 04:07:39 +01:00
|
|
|
Format,
|
2022-05-10 13:35:14 +02:00
|
|
|
FileSize,
|
2021-12-11 04:07:39 +01:00
|
|
|
Parse,
|
|
|
|
Size,
|
2021-10-10 22:24:54 +02:00
|
|
|
Split,
|
|
|
|
SplitChars,
|
|
|
|
SplitColumn,
|
|
|
|
SplitRow,
|
2022-08-19 19:55:54 +02:00
|
|
|
SplitWords,
|
2021-11-09 02:59:44 +01:00
|
|
|
Str,
|
|
|
|
StrCamelCase,
|
2021-11-09 08:40:56 +01:00
|
|
|
StrCapitalize,
|
2021-11-09 02:59:44 +01:00
|
|
|
StrCollect,
|
2021-11-09 21:16:53 +01:00
|
|
|
StrContains,
|
2022-08-23 15:53:14 +02:00
|
|
|
StrDistance,
|
2021-11-09 21:16:53 +01:00
|
|
|
StrDowncase,
|
2021-11-10 01:51:55 +01:00
|
|
|
StrEndswith,
|
2022-09-11 10:48:27 +02:00
|
|
|
StrJoin,
|
2022-04-07 15:41:09 +02:00
|
|
|
StrReplace,
|
2021-12-11 04:07:39 +01:00
|
|
|
StrIndexOf,
|
2021-11-09 02:59:44 +01:00
|
|
|
StrKebabCase,
|
2021-12-11 04:07:39 +01:00
|
|
|
StrLength,
|
|
|
|
StrLpad,
|
2021-11-09 02:59:44 +01:00
|
|
|
StrPascalCase,
|
2021-12-11 04:07:39 +01:00
|
|
|
StrReverse,
|
|
|
|
StrRpad,
|
2021-11-09 02:59:44 +01:00
|
|
|
StrScreamingSnakeCase,
|
|
|
|
StrSnakeCase,
|
2021-11-16 00:16:56 +01:00
|
|
|
StrStartsWith,
|
2021-12-01 07:42:57 +01:00
|
|
|
StrSubstring,
|
2021-12-02 05:38:44 +01:00
|
|
|
StrTrim,
|
2022-05-18 15:57:20 +02:00
|
|
|
StrTitleCase,
|
2021-12-17 18:40:47 +01:00
|
|
|
StrUpcase
|
2021-12-11 04:07:39 +01:00
|
|
|
};
|
|
|
|
|
2022-07-30 11:25:44 +02:00
|
|
|
// Bits
|
|
|
|
bind_command! {
|
2022-07-30 14:34:11 +02:00
|
|
|
Bits,
|
|
|
|
BitsAnd,
|
|
|
|
BitsNot,
|
2022-07-30 20:26:37 +02:00
|
|
|
BitsOr,
|
|
|
|
BitsXor,
|
2022-08-05 15:40:01 +02:00
|
|
|
BitsRotateLeft,
|
|
|
|
BitsRotateRight,
|
2022-08-02 22:52:04 +02:00
|
|
|
BitsShiftLeft,
|
|
|
|
BitsShiftRight,
|
2022-07-30 11:25:44 +02:00
|
|
|
}
|
|
|
|
|
2022-07-04 12:51:07 +02:00
|
|
|
// Bytes
|
|
|
|
bind_command! {
|
2022-07-06 06:46:56 +02:00
|
|
|
Bytes,
|
2022-07-05 13:42:01 +02:00
|
|
|
BytesLen,
|
2022-07-06 15:25:37 +02:00
|
|
|
BytesStartsWith,
|
|
|
|
BytesEndsWith,
|
|
|
|
BytesReverse,
|
|
|
|
BytesReplace,
|
2022-07-09 04:42:31 +02:00
|
|
|
BytesAdd,
|
|
|
|
BytesAt,
|
|
|
|
BytesIndexOf,
|
2022-07-11 13:26:00 +02:00
|
|
|
BytesCollect,
|
|
|
|
BytesRemove,
|
|
|
|
BytesBuild,
|
2022-07-04 12:51:07 +02:00
|
|
|
}
|
|
|
|
|
2021-12-11 04:07:39 +01:00
|
|
|
// FileSystem
|
|
|
|
bind_command! {
|
|
|
|
Cd,
|
|
|
|
Cp,
|
|
|
|
Ls,
|
|
|
|
Mkdir,
|
|
|
|
Mv,
|
2021-12-24 20:24:55 +01:00
|
|
|
Open,
|
2023-01-03 19:47:37 +01:00
|
|
|
Start,
|
2021-12-11 04:07:39 +01:00
|
|
|
Rm,
|
2022-01-15 21:44:20 +01:00
|
|
|
Save,
|
2021-12-11 04:07:39 +01:00
|
|
|
Touch,
|
2022-04-04 22:45:01 +02:00
|
|
|
Glob,
|
2022-04-28 16:26:34 +02:00
|
|
|
Watch,
|
2021-12-11 04:07:39 +01:00
|
|
|
};
|
|
|
|
|
|
|
|
// Platform
|
|
|
|
bind_command! {
|
2021-12-17 18:40:47 +01:00
|
|
|
Ansi,
|
|
|
|
AnsiGradient,
|
2021-12-17 21:32:03 +01:00
|
|
|
AnsiStrip,
|
2023-01-15 16:23:37 +01:00
|
|
|
AnsiLink,
|
2021-12-11 04:07:39 +01:00
|
|
|
Clear,
|
2022-06-15 20:11:26 +02:00
|
|
|
Du,
|
2022-02-05 18:35:02 +01:00
|
|
|
KeybindingsDefault,
|
2022-01-16 05:28:28 +01:00
|
|
|
Input,
|
2022-02-05 18:35:02 +01:00
|
|
|
KeybindingsListen,
|
2022-02-04 07:47:18 +01:00
|
|
|
Keybindings,
|
2021-12-11 04:07:39 +01:00
|
|
|
Kill,
|
2022-02-05 18:35:02 +01:00
|
|
|
KeybindingsList,
|
2021-12-11 04:07:39 +01:00
|
|
|
Sleep,
|
2022-01-21 04:31:33 +01:00
|
|
|
TermSize,
|
2021-12-11 04:07:39 +01:00
|
|
|
};
|
|
|
|
|
|
|
|
// Date
|
|
|
|
bind_command! {
|
|
|
|
Date,
|
|
|
|
DateFormat,
|
|
|
|
DateHumanize,
|
|
|
|
DateListTimezones,
|
|
|
|
DateNow,
|
2022-04-01 10:09:30 +02:00
|
|
|
DateToRecord,
|
2021-12-11 04:07:39 +01:00
|
|
|
DateToTable,
|
|
|
|
DateToTimezone,
|
|
|
|
};
|
|
|
|
|
|
|
|
// Shells
|
|
|
|
bind_command! {
|
2022-01-05 05:35:50 +01:00
|
|
|
Enter,
|
2021-12-11 04:07:39 +01:00
|
|
|
Exit,
|
2022-01-05 05:35:50 +01:00
|
|
|
GotoShell,
|
|
|
|
NextShell,
|
|
|
|
PrevShell,
|
|
|
|
Shells,
|
2021-12-11 04:07:39 +01:00
|
|
|
};
|
|
|
|
|
|
|
|
// Formats
|
|
|
|
bind_command! {
|
|
|
|
From,
|
|
|
|
FromCsv,
|
|
|
|
FromEml,
|
|
|
|
FromIcs,
|
|
|
|
FromIni,
|
|
|
|
FromJson,
|
2022-02-20 22:26:41 +01:00
|
|
|
FromNuon,
|
2021-12-11 04:07:39 +01:00
|
|
|
FromOds,
|
|
|
|
FromSsv,
|
|
|
|
FromToml,
|
|
|
|
FromTsv,
|
|
|
|
FromUrl,
|
|
|
|
FromVcf,
|
|
|
|
FromXlsx,
|
|
|
|
FromXml,
|
|
|
|
FromYaml,
|
|
|
|
FromYml,
|
2021-10-29 08:26:29 +02:00
|
|
|
To,
|
2021-12-03 03:02:22 +01:00
|
|
|
ToCsv,
|
2021-12-10 02:16:35 +01:00
|
|
|
ToHtml,
|
2021-12-11 04:07:39 +01:00
|
|
|
ToJson,
|
2021-12-10 02:16:35 +01:00
|
|
|
ToMd,
|
2022-02-20 22:26:41 +01:00
|
|
|
ToNuon,
|
2022-05-04 21:12:23 +02:00
|
|
|
ToText,
|
2021-12-11 04:07:39 +01:00
|
|
|
ToToml,
|
|
|
|
ToTsv,
|
2021-12-11 21:08:17 +01:00
|
|
|
Touch,
|
|
|
|
Use,
|
2022-03-17 01:13:34 +01:00
|
|
|
Upsert,
|
2021-12-11 21:08:17 +01:00
|
|
|
Where,
|
2021-12-10 21:46:43 +01:00
|
|
|
ToXml,
|
2021-12-11 04:07:39 +01:00
|
|
|
ToYaml,
|
|
|
|
};
|
|
|
|
|
|
|
|
// Viewers
|
|
|
|
bind_command! {
|
|
|
|
Griddle,
|
|
|
|
Table,
|
[MVP][WIP] `less` like pager (#6984)
Run it as `explore`.
#### example
```nu
ls | explore
```
Configuration points in `config.nu` file.
```
# A 'explore' utility config
explore_config: {
highlight: { bg: 'yellow', fg: 'black' }
status_bar: { bg: '#C4C9C6', fg: '#1D1F21' }
command_bar: { fg: '#C4C9C6' }
split_line: '#404040'
cursor: true
# selected_column: 'blue'
# selected_row: { fg: 'yellow', bg: '#C1C2A3' }
# selected_cell: { fg: 'white', bg: '#777777' }
# line_shift: false,
# line_index: false,
# line_head_top: false,
# line_head_bottom: false,
}
```
You can start without a pipeline and type `explore` and it'll give you a
few tips.
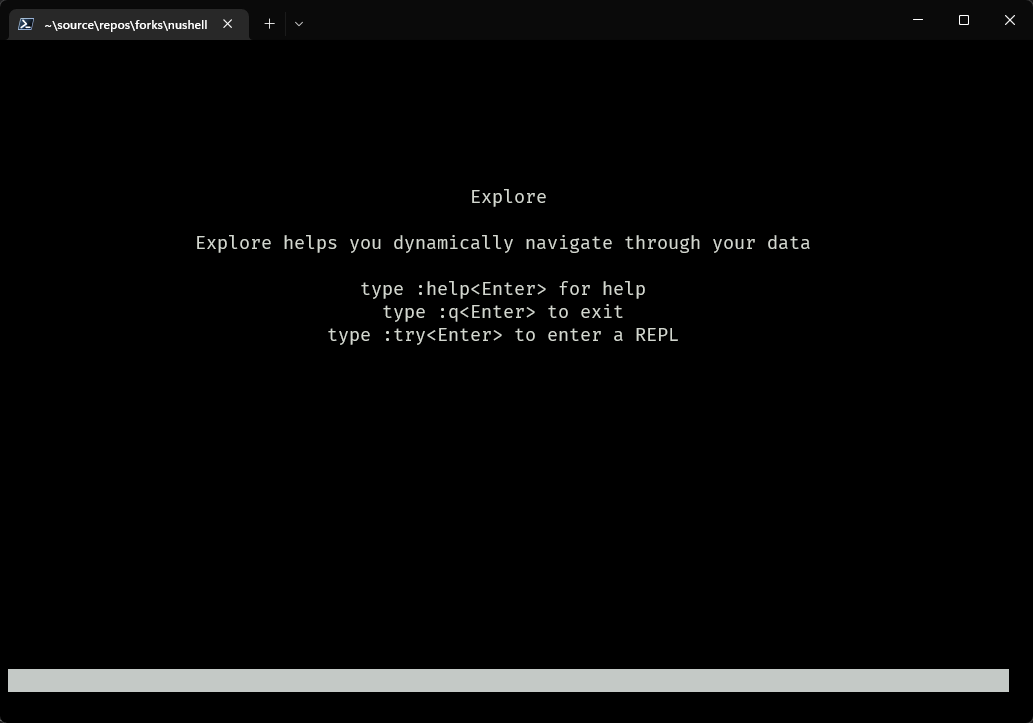
If you type `:help` you an see the help screen with some information on
what tui keybindings are available.
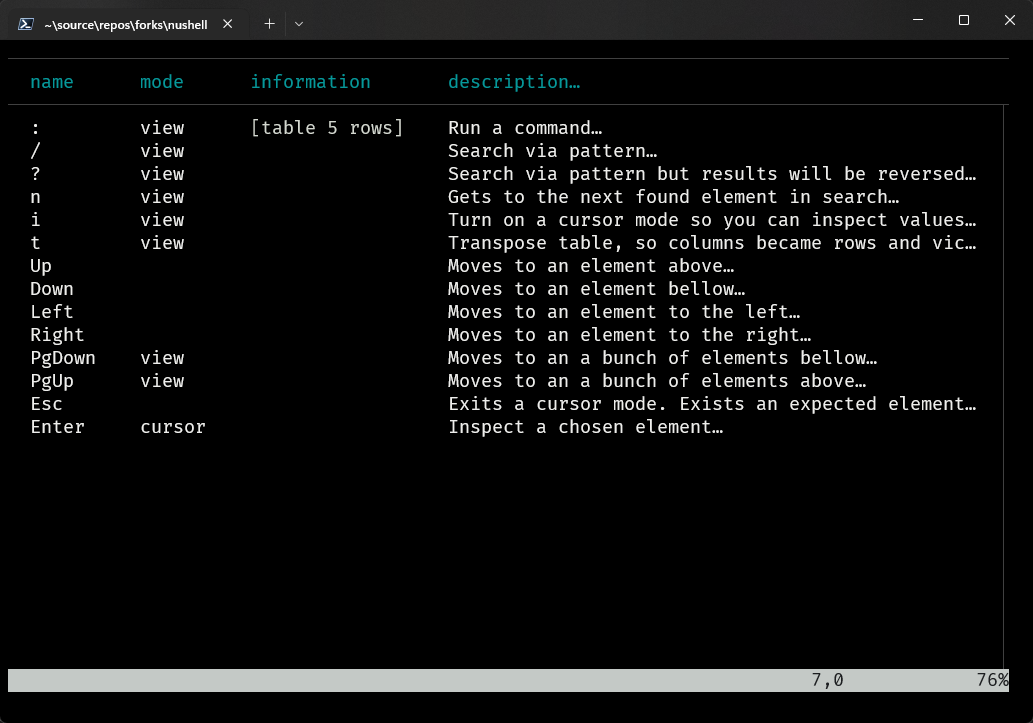
From the `:help` screen you can now hit `i` and that puts you in
`cursor` aka `inspection` mode and you can move the cursor left right up
down and it you put it on an area such as `[table 5 rows]` and hit the
enter key, you'll see something like this, which shows all the `:`
commands. If you hit `esc` it will take you to the previous screen.
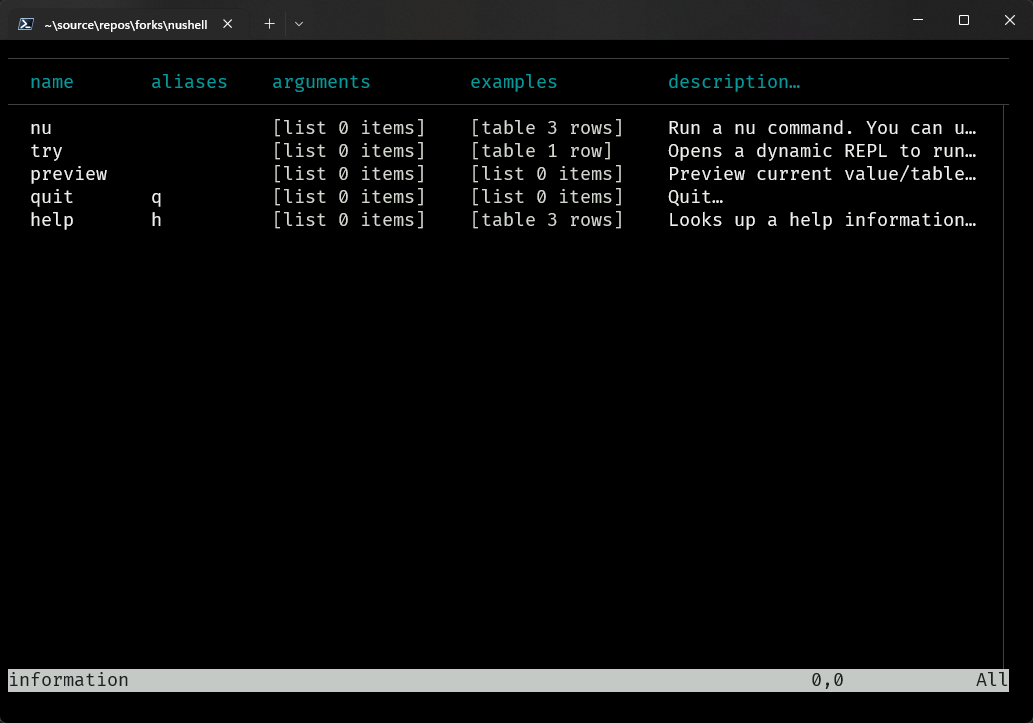
If you then type `:try` you'll get this type of window where you can
type in the top portion and see results in the bottom.
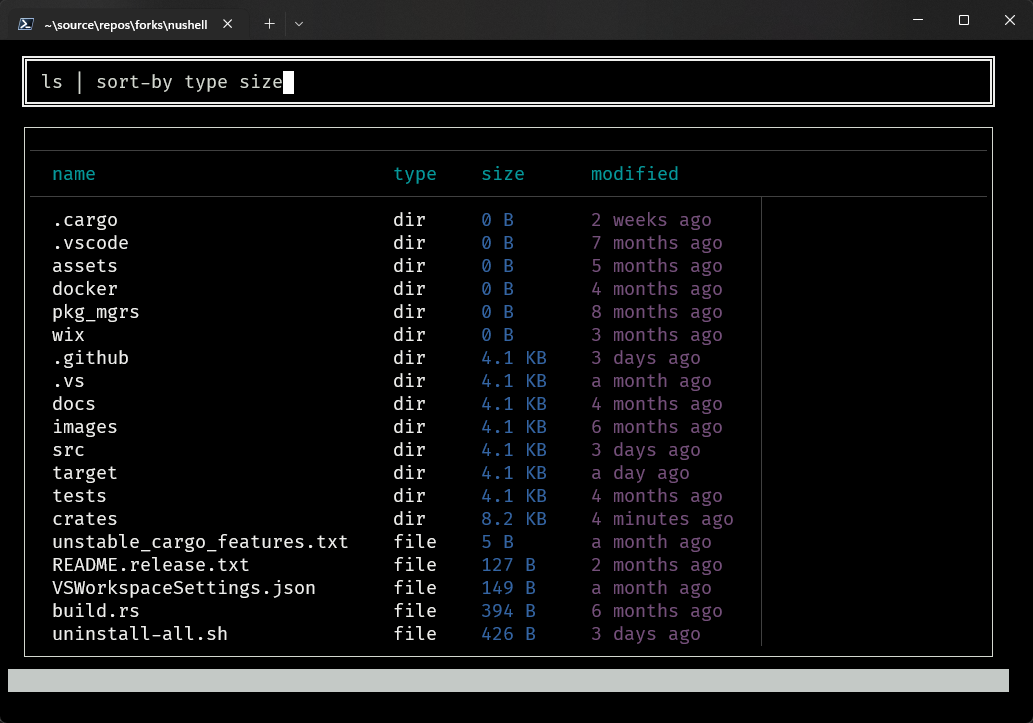
The `:nu` command is interesting because you can type pipelines like
`:nu ls | sort-by type size` or another pipeline of your choosing such
as `:nu sys` and that will show the table that looks like this, which
we're calling "table mode".
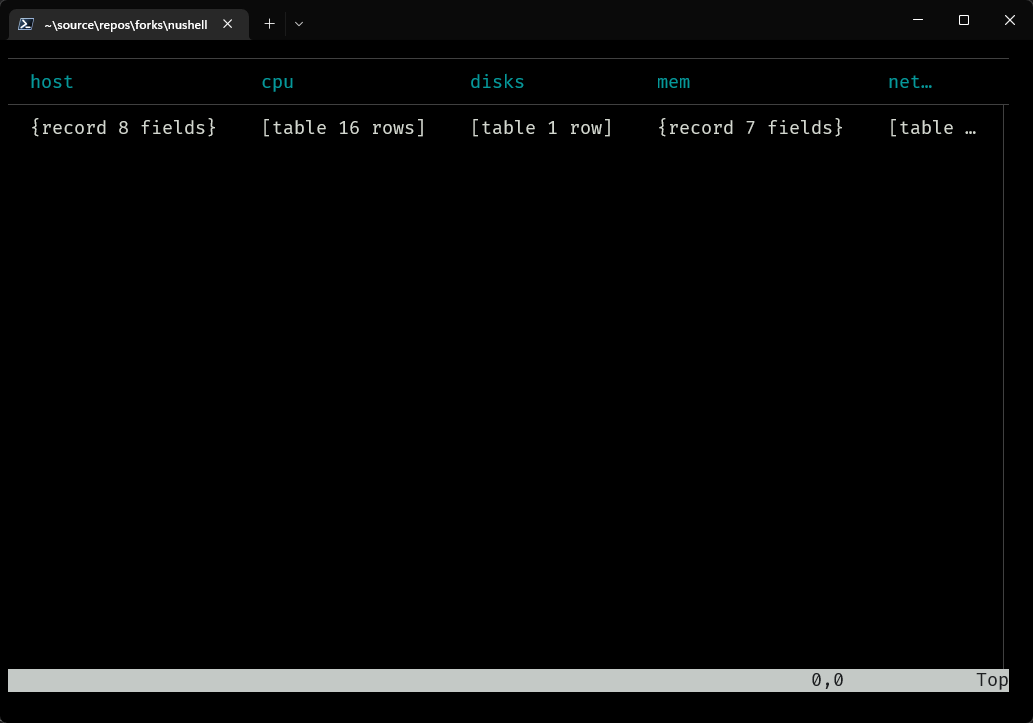
If you hit the `t` key it will now transpose the view to look like this.
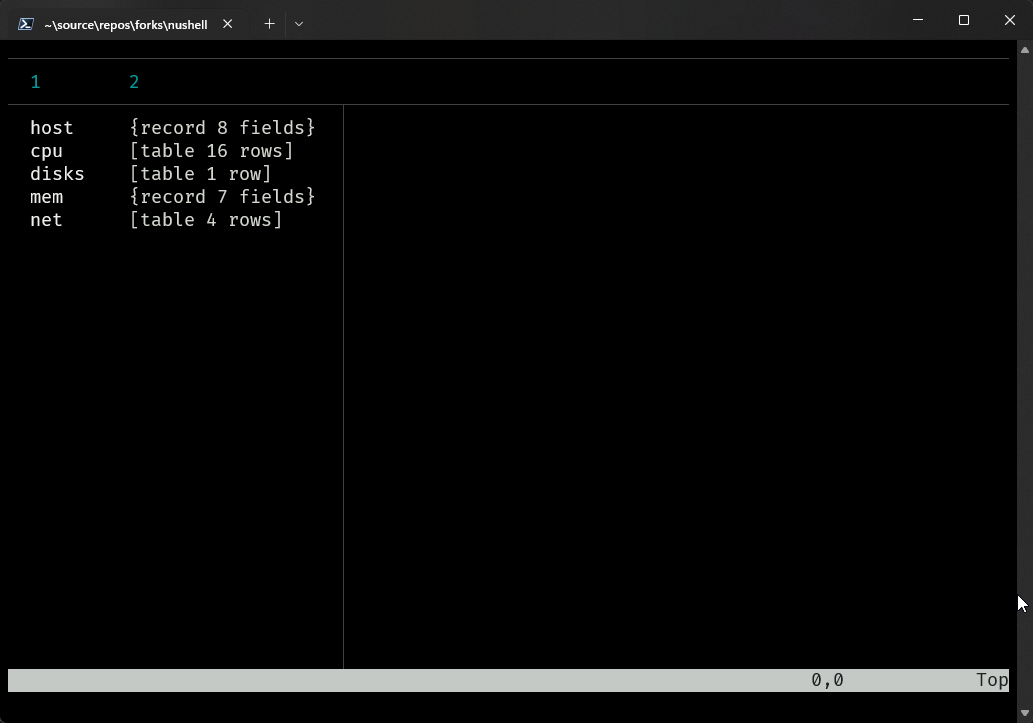
In table mode or transposed table mode you can use the `i` key to
inspect any collapsed field like `{record 8 fields}`, `[table 16 rows]`,
`[list x]`, etc.
One of the original benefits was that when you're in a view that has a
lot of columns, `explore` gives you the ability to scroll left, right,
up, and down.
`explore` is also smart enough to know when you're in table mode versus
preview mode. If you do `open Cargo.toml | explore` you get this.
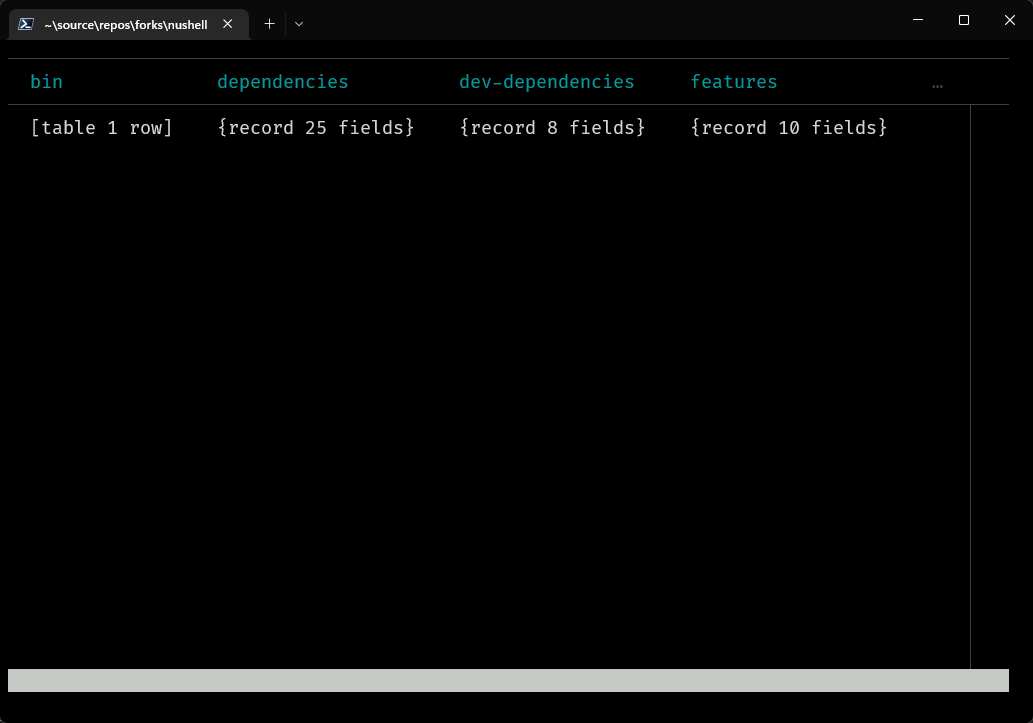
If you type `open --raw Cargo.toml | explore` you get this where you can
scroll left, right, up, down. This is called preview mode.
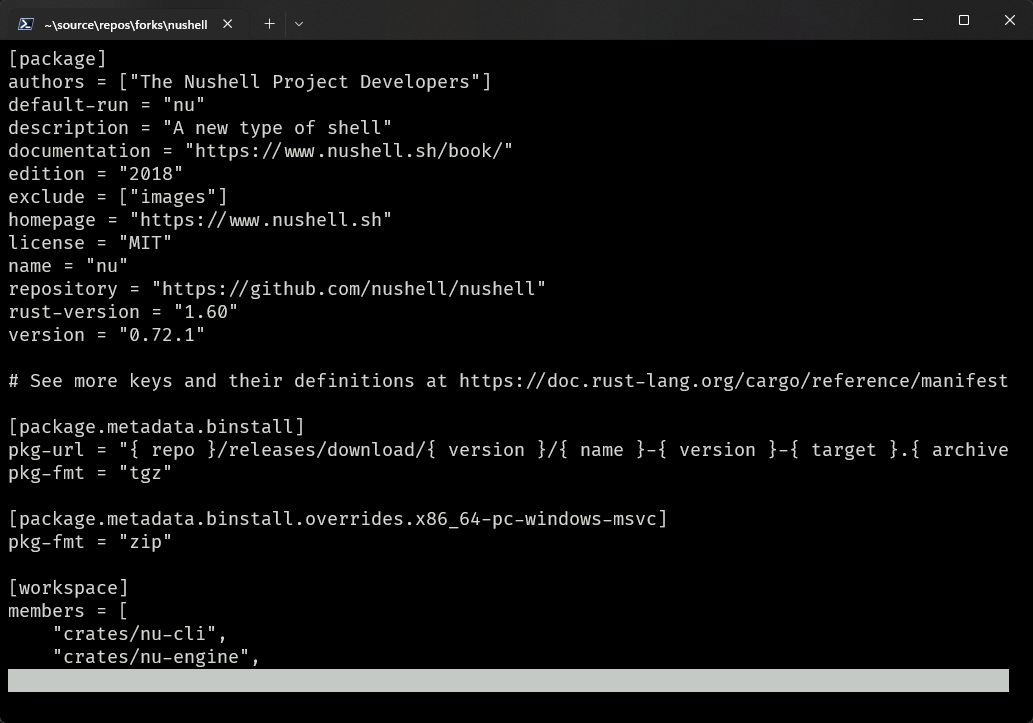
When you're in table mode, you can also type `:preview`. So, with `open
--raw Cargo.toml | explore`, if you type `:preview`, it will look like
this.
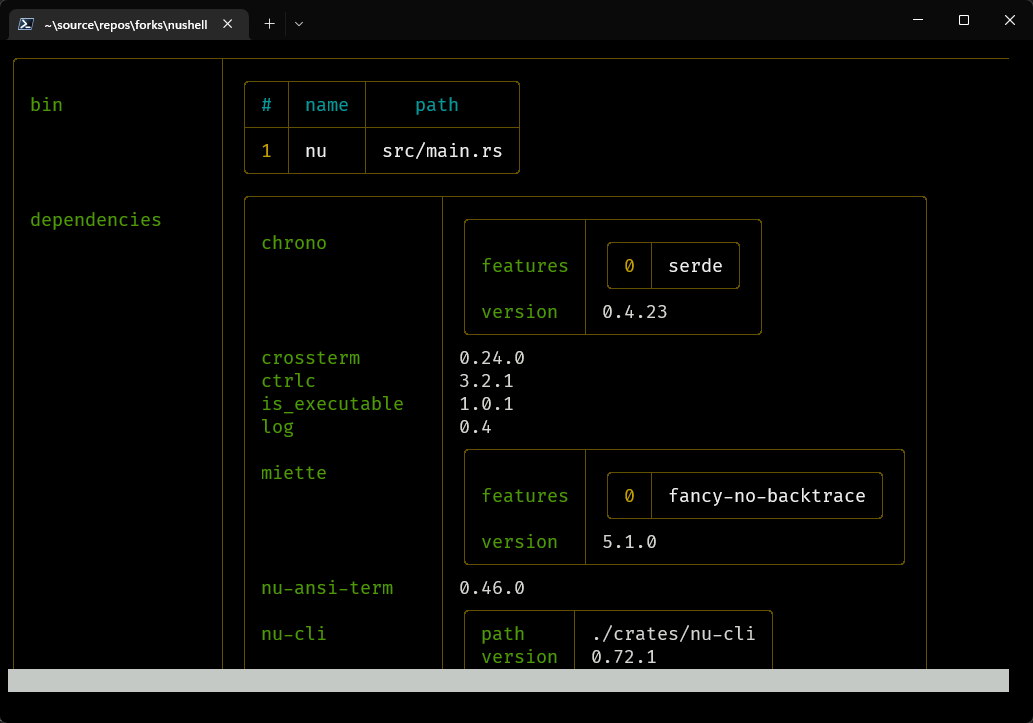
Signed-off-by: Maxim Zhiburt <zhiburt@gmail.com>
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2022-12-01 16:32:10 +01:00
|
|
|
Explore,
|
2021-12-11 04:07:39 +01:00
|
|
|
};
|
|
|
|
|
|
|
|
// Conversions
|
|
|
|
bind_command! {
|
2022-01-16 00:50:11 +01:00
|
|
|
Fmt,
|
2021-12-11 04:07:39 +01:00
|
|
|
Into,
|
2021-12-19 21:11:28 +01:00
|
|
|
IntoBool,
|
2021-12-11 04:07:39 +01:00
|
|
|
IntoBinary,
|
|
|
|
IntoDatetime,
|
|
|
|
IntoDecimal,
|
2022-03-03 14:16:04 +01:00
|
|
|
IntoDuration,
|
2021-12-11 04:07:39 +01:00
|
|
|
IntoFilesize,
|
|
|
|
IntoInt,
|
2022-11-26 16:00:47 +01:00
|
|
|
IntoRecord,
|
2021-12-11 04:07:39 +01:00
|
|
|
IntoString,
|
|
|
|
};
|
|
|
|
|
|
|
|
// Env
|
|
|
|
bind_command! {
|
2022-01-16 00:50:11 +01:00
|
|
|
Env,
|
2022-08-18 22:24:39 +02:00
|
|
|
ExportEnv,
|
2021-12-11 04:07:39 +01:00
|
|
|
LetEnv,
|
2022-01-16 00:50:11 +01:00
|
|
|
LoadEnv,
|
2022-08-31 22:32:56 +02:00
|
|
|
SourceEnv,
|
2021-11-04 03:32:35 +01:00
|
|
|
WithEnv,
|
2022-05-22 05:13:58 +02:00
|
|
|
ConfigNu,
|
|
|
|
ConfigEnv,
|
2022-05-22 19:31:57 +02:00
|
|
|
ConfigMeta,
|
2022-08-01 03:44:33 +02:00
|
|
|
ConfigReset,
|
2021-12-11 04:07:39 +01:00
|
|
|
};
|
|
|
|
|
|
|
|
// Math
|
|
|
|
bind_command! {
|
|
|
|
Math,
|
|
|
|
MathAbs,
|
|
|
|
MathAvg,
|
|
|
|
MathCeil,
|
|
|
|
MathFloor,
|
|
|
|
MathMax,
|
|
|
|
MathMedian,
|
|
|
|
MathMin,
|
|
|
|
MathMode,
|
|
|
|
MathProduct,
|
|
|
|
MathRound,
|
|
|
|
MathSqrt,
|
|
|
|
MathStddev,
|
|
|
|
MathSum,
|
|
|
|
MathVariance,
|
2022-11-27 16:28:27 +01:00
|
|
|
MathSin,
|
|
|
|
MathCos,
|
|
|
|
MathTan,
|
2022-11-27 16:29:38 +01:00
|
|
|
MathSinH,
|
|
|
|
MathCosH,
|
|
|
|
MathTanH,
|
2022-11-27 20:22:07 +01:00
|
|
|
MathArcSin,
|
|
|
|
MathArcCos,
|
|
|
|
MathArcTan,
|
2022-12-01 15:32:43 +01:00
|
|
|
MathArcSinH,
|
|
|
|
MathArcCosH,
|
|
|
|
MathArcTanH,
|
2022-11-27 16:25:51 +01:00
|
|
|
MathPi,
|
2022-12-09 10:27:19 +01:00
|
|
|
MathTau,
|
2022-11-27 16:25:51 +01:00
|
|
|
MathEuler,
|
2022-11-27 21:16:53 +01:00
|
|
|
MathLn,
|
2022-12-09 11:20:42 +01:00
|
|
|
MathLog,
|
2021-12-11 04:07:39 +01:00
|
|
|
};
|
|
|
|
|
2021-12-12 20:42:04 +01:00
|
|
|
// Network
|
|
|
|
bind_command! {
|
2023-01-20 19:38:30 +01:00
|
|
|
Http,
|
|
|
|
HttpGet,
|
|
|
|
HttpPost,
|
2021-12-12 20:42:04 +01:00
|
|
|
Url,
|
2023-01-15 19:16:29 +01:00
|
|
|
UrlBuildQuery,
|
2023-01-05 19:24:38 +01:00
|
|
|
UrlEncode,
|
Feat/7725 url join (#7823)
# Description
Added command: `url join`.
Closes: #7725
# User-Facing Changes
```
Converts a record to url
Search terms: scheme, username, password, hostname, port, path, query, fragment
Usage:
> url join
Flags:
-h, --help - Display the help message for this command
Signatures:
<record> | url join -> <string>
Examples:
Outputs a url representing the contents of this record
> {
"scheme": "http",
"username": "",
"password": "",
"host": "www.pixiv.net",
"port": "",
"path": "/member_illust.php",
"query": "mode=medium&illust_id=99260204",
"fragment": "",
"params":
{
"mode": "medium",
"illust_id": "99260204"
}
} | url join
Outputs a url representing the contents of this record
> {
"scheme": "http",
"username": "user",
"password": "pwd",
"host": "www.pixiv.net",
"port": "1234",
"query": "test=a",
"fragment": ""
} | url join
Outputs a url representing the contents of this record
> {
"scheme": "http",
"host": "www.pixiv.net",
"port": "1234",
"path": "user",
"fragment": "frag"
} | url join
```
# Questions
- Which parameters should be required? Currenlty are: `scheme` and
`host`.
2023-01-22 19:49:40 +01:00
|
|
|
UrlJoin,
|
2022-11-19 19:14:29 +01:00
|
|
|
UrlParse,
|
2022-06-22 05:27:58 +02:00
|
|
|
Port,
|
2021-12-12 20:42:04 +01:00
|
|
|
}
|
|
|
|
|
2021-12-11 04:07:39 +01:00
|
|
|
// Random
|
|
|
|
bind_command! {
|
|
|
|
Random,
|
2021-12-12 20:42:04 +01:00
|
|
|
RandomBool,
|
|
|
|
RandomChars,
|
|
|
|
RandomDecimal,
|
|
|
|
RandomDice,
|
|
|
|
RandomInteger,
|
|
|
|
RandomUuid,
|
2021-12-11 04:07:39 +01:00
|
|
|
};
|
|
|
|
|
|
|
|
// Generators
|
|
|
|
bind_command! {
|
|
|
|
Cal,
|
2022-01-16 14:52:41 +01:00
|
|
|
Seq,
|
2022-01-14 23:07:28 +01:00
|
|
|
SeqDate,
|
2022-05-06 17:40:02 +02:00
|
|
|
SeqChar,
|
2021-12-11 04:07:39 +01:00
|
|
|
};
|
|
|
|
|
|
|
|
// Hash
|
|
|
|
bind_command! {
|
2021-12-11 00:14:28 +01:00
|
|
|
Hash,
|
|
|
|
HashMd5::default(),
|
|
|
|
HashSha256::default(),
|
2021-12-11 04:07:39 +01:00
|
|
|
};
|
2021-09-03 04:15:01 +02:00
|
|
|
|
2022-01-30 23:05:25 +01:00
|
|
|
// Experimental
|
|
|
|
bind_command! {
|
2022-06-06 13:55:23 +02:00
|
|
|
IsAdmin,
|
view span & view files commands (#7989)
# Description
This PR does the following:
1. Adds a new command called `view span` - which shows what is at the
location of the span parameters
2. Adds a new command called `view` - which just lists all the `view`
commands.
3. Renames `view-source` to `view source`.
4. Adds a new command called `view files` - which shows you what files
are loaded into nushell's EngineState memory.
5. Added a `Category::Debug` and put these commands (and others) into
it. (`inspect` needs to be added to it, but it's not landed yet)
Spans are important to nushell. One of their uses is to show where
errors are. For instance, in this example, the leader lines pointing to
parts of the command line are able to point to `10`, `/`, and `"bob"`
because each of those items have a span.
```
> 10 / "bob"
Error: nu::parser::unsupported_operation (link)
× Types mismatched for operation.
╭─[entry #8:1:1]
1 │ 10 / "bob"
· ─┬ ┬ ──┬──
· │ │ ╰── string
· │ ╰── doesn't support these values.
· ╰── int
╰────
help: Change int or string to be the right types and try again.
```
# Examples
## view span
Example:
```
> $env.config | get keybindings | first | debug -r
... bunch of stuff
span: Span {
start: 68065,
end: 68090,
},
},
],
span: Span {
start: 68050,
end: 68101,
},
},
],
span: Span {
start: 67927,
end: 68108,
},
}
```
To view the last span:
```
> view span 67927 68108
{
name: clear_everything
modifier: control
keycode: char_l
mode: emacs
event: [
{ send: clearscrollback }
]
}
```
> To view the 2nd to last span:
```
view span 68065 68090
{ send: clearscrollback }
```
> To view the 3rd to last span:
```
view span 68050 68101
[
{ send: clearscrollback }
]
```
## view files
```
> view files
╭────┬───────────────────────────────────────────────────────┬────────┬────────┬───────╮
│ # │ filename │ start │ end │ size │
├────┼───────────────────────────────────────────────────────┼────────┼────────┼───────┤
│ 0 │ source │ 0 │ 2 │ 2 │
│ 1 │ Host Environment Variables │ 2 │ 6034 │ 6032 │
│ 2 │ C:\Users\a_username\AppData\Roaming\nushell\plugin.nu │ 6034 │ 31236 │ 25202 │
│ 3 │ C:\Users\a_username\AppData\Roaming\nushell\env.nu │ 31236 │ 44961 │ 13725 │
│ 4 │ C:\Users\a_username\AppData\Roaming\nushell\config.nu │ 44961 │ 76134 │ 31173 │
│ 5 │ defs.nu │ 76134 │ 91944 │ 15810 │
│ 6 │ prompt\oh-my.nu │ 91944 │ 111523 │ 19579 │
│ 7 │ weather\get-weather.nu │ 111523 │ 125556 │ 14033 │
│ 8 │ .zoxide.nu │ 125556 │ 127504 │ 1948 │
│ 9 │ source │ 127504 │ 127561 │ 57 │
│ 10 │ entry #1 │ 127561 │ 127585 │ 24 │
│ 11 │ entry #2 │ 127585 │ 127595 │ 10 │
╰────┴───────────────────────────────────────────────────────┴────────┴────────┴───────╯
```
`entry #x` will be each command you type in the repl (i think). so, it
may be good to filter those out sometimes.
```
> view files | where filename !~ entry
╭───┬───────────────────────────────────────────────────────┬────────┬────────┬───────╮
│ # │ filename │ start │ end │ size │
├───┼───────────────────────────────────────────────────────┼────────┼────────┼───────┤
│ 0 │ source │ 0 │ 2 │ 2 │
│ 1 │ Host Environment Variables │ 2 │ 6034 │ 6032 │
│ 2 │ C:\Users\a_username\AppData\Roaming\nushell\plugin.nu │ 6034 │ 31236 │ 25202 │
│ 3 │ C:\Users\a_username\AppData\Roaming\nushell\env.nu │ 31236 │ 44961 │ 13725 │
│ 4 │ C:\Users\a_username\AppData\Roaming\nushell\config.nu │ 44961 │ 76134 │ 31173 │
│ 5 │ defs.nu │ 76134 │ 91944 │ 15810 │
│ 6 │ prompt\oh-my.nu │ 91944 │ 111523 │ 19579 │
│ 7 │ weather\get-weather.nu │ 111523 │ 125556 │ 14033 │
│ 8 │ .zoxide.nu │ 125556 │ 127504 │ 1948 │
│ 9 │ source │ 127504 │ 127561 │ 57 │
╰───┴───────────────────────────────────────────────────────┴────────┴────────┴───────╯
```
# User-Facing Changes
I renamed `view-source` to `view source` just to make a group of
commands. No functionality has changed in `view source`.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
2023-02-09 18:35:23 +01:00
|
|
|
View,
|
|
|
|
ViewFiles,
|
|
|
|
ViewSource,
|
|
|
|
ViewSpan,
|
2022-01-30 23:05:25 +01:00
|
|
|
};
|
|
|
|
|
2022-02-10 13:55:19 +01:00
|
|
|
// Deprecated
|
|
|
|
bind_command! {
|
2022-08-08 16:46:59 +02:00
|
|
|
HashBase64,
|
2022-08-31 22:32:56 +02:00
|
|
|
Source,
|
2022-02-13 03:30:37 +01:00
|
|
|
StrDatetimeDeprecated,
|
2022-02-10 13:55:19 +01:00
|
|
|
StrDecimalDeprecated,
|
|
|
|
StrIntDeprecated,
|
2022-04-07 15:41:09 +02:00
|
|
|
StrFindReplaceDeprecated,
|
2023-01-04 23:50:18 +01:00
|
|
|
MathEvalDeprecated,
|
2022-02-10 13:55:19 +01:00
|
|
|
};
|
|
|
|
|
2021-11-02 21:56:00 +01:00
|
|
|
#[cfg(feature = "plugin")]
|
|
|
|
bind_command!(Register);
|
|
|
|
|
2021-09-03 00:58:15 +02:00
|
|
|
working_set.render()
|
|
|
|
};
|
|
|
|
|
2022-07-14 16:09:27 +02:00
|
|
|
if let Err(err) = engine_state.merge_delta(delta) {
|
2023-01-30 02:37:54 +01:00
|
|
|
eprintln!("Error creating default context: {err:?}");
|
2022-07-14 16:09:27 +02:00
|
|
|
}
|
2021-09-03 00:58:15 +02:00
|
|
|
|
|
|
|
engine_state
|
2021-01-01 03:12:16 +01:00
|
|
|
}
|