This PR is an attempt to fix#8257 and fix#10985 (which is
duplicate-ish)
# Description
The parser currently doesn't know how to deal with colons appearing
while lexing whitespace-terminated tokens specifying a record value.
Most notably, this means you can't use datetime literals in record value
position (and as a consequence, `| to nuon | from nuon` roundtrips can
fail), but it also means that bare words containing colons cause a
non-useful error message.
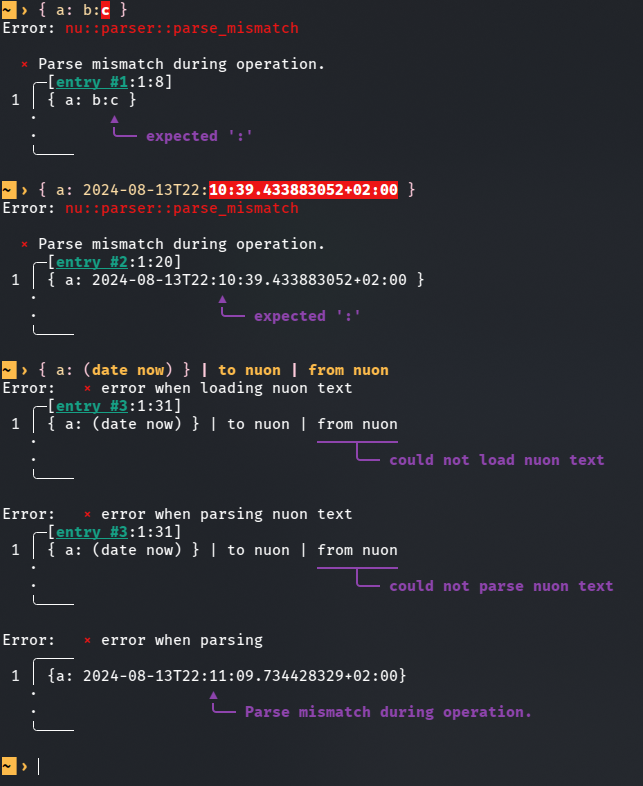
`parser::parse_record` calls `lex::lex` with the `:` colon character in
the `special_tokens` argument. This allows colons to terminate record
keys, but as a side effect, it also causes colons to terminate record
*values*. I added a new function `lex::lex_n_tokens`, which allows the
caller to drive the lexing process more explicitly, and used it in
`parser::parse_record` to let colons terminate record keys while not
giving them special treatment when appearing in record values.
This PR description previously said: *Another approach suggested in one
of the issues was to support an additional datetime literal format that
doesn't require colons. I like that that wouldn't require new
`lex::lex_internal` behaviour, but an advantage of my approach is that
it also newly allows for string record values given as bare words
containing colons. I think this eliminates another possible source of
confusion.* It was determined that this is undesirable, and in the
current state of this PR, bare word record values with colons are
rejected explicitly. The better error message is still a win.
# User-Facing Changes
In addition to the above, this PR also disables the use of "special"
(non-item) tokens in record key and value position, and the use of a
single bare `:` as a record key.
Examples of behaviour *before* this PR:
```nu
{ a: b } # Valid, same as { 'a': 'b' }
{ a: b:c } # Error: expected ':'
{ a: 2024-08-13T22:11:09 } # Error: expected ':'
{ :: 1 } # Valid, same as { ':': 1 }
{ ;: 1 } # Valid, same as { ';': 1 }
{ a: || } # Valid, same as { 'a': '||' }
```
Examples of behaviour *after* this PR:
```nu
{ a: b } # (Unchanged) Valid, same as { 'a': 'b' }
{ a: b:c } # Error: colon in bare word specifying record value
{ a: 2024-08-13T22:11:09 } # Valid, same as { a: (2024-08-13T22:11:09) }
{ :: 1 } # Error: colon in bare word specifying record key
{ ;: 1 } # Error: expected item in record key position
{ a: || } # Error: expected item in record value position
```
# Tests + Formatting
I added tests, but I'm not sure if they're sufficient and in the right
place.
# After Submitting
I don't think documentation changes are needed for this, but please let
me know if you disagree.
# Description
This makes assignment operations and `const` behave the same way `let`
and `mut` do, absorbing the rest of the pipeline.
Changes the lexer to be able to recognize assignment operators as a
separate token, and then makes the lite parser continue to push spans
into the same command regardless of any redirections or pipes if an
assignment operator is encountered. Because the pipeline is no longer
split up by the lite parser at this point, it's trivial to just parse
the right hand side as if it were a subexpression not contained within
parentheses.
# User-Facing Changes
Big breaking change. These are all now possible:
```nushell
const path = 'a' | path join 'b'
mut x = 2
$x = random int
$x = [1 2 3] | math sum
$env.FOO = random chars
```
In the past, these would have led to (an attempt at) bare word string
parsing. So while `$env.FOO = bar` would have previously set the
environment variable `FOO` to the string `"bar"`, it now tries to run
the command named `bar`, hence the major breaking change.
However, this is desirable because it is very consistent - if you see
the `=`, you can just assume it absorbs everything else to the right of
it.
# Tests + Formatting
Added tests for the new behaviour. Adjusted some existing tests that
depended on the right hand side of assignments being parsed as
barewords.
# After Submitting
- [ ] release notes (breaking change!)
# Description
Fixes the lexer to recognize `out>|`, `err>|`, `out+err>|`, etc.
Previously only the short-style forms were recognized, which was
inconsistent with normal file redirections.
I also integrated it all more into the normal lex path by checking `|`
in a special way, which should be more performant and consistent, and
cleans up the code a bunch.
Closes#13331.
# User-Facing Changes
- Adds `out>|` (error), `err>|`, `out+err>|`, `err+out>|` as recognized
forms of the pipe redirection.
# Tests + Formatting
All passing. Added tests for the new forms.
# After Submitting
- [ ] release notes
# Description
From the feedbacks from @amtoine , it's good to make nushell shows error
for `o>|` syntax.
# User-Facing Changes
## Before
```nushell
'foo' o>| print 07/09/2024 06:44:23 AM
Error: nu::parser::parse_mismatch
× Parse mismatch during operation.
╭─[entry #6:1:9]
1 │ 'foo' o>| print
· ┬
· ╰── expected redirection target
```
## After
```nushell
'foo' o>| print 07/09/2024 06:47:26 AM
Error: nu::parser::parse_mismatch
× Parse mismatch during operation.
╭─[entry #1:1:7]
1 │ 'foo' o>| print
· ─┬─
· ╰── expected `|`. Redirection stdout to pipe is the same as piping directly.
╰────
```
# Tests + Formatting
Added one test
---------
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
# Description
Fixes: #12795
The issue is caused by an empty position of `ParseError::UnexpectedEof`.
So no detailed message is displayed.
To fix the issue, I adjust the start of span to `span.end - 1`. In this
way, we can make sure that it never points to an empty position.
After lexing item, I also reorder the unclosed character checking . Now
it will be checking unclosed opening delimiters first.
# User-Facing Changes
After this pr, it outputs detailed error message for incomplete string
when running scripts.
## Before
```
❯ nu -c "'ab"
Error: nu::parser::unexpected_eof
× Unexpected end of code.
╭─[source:1:4]
1 │ 'ab
╰────
> ./target/debug/nu -c "r#'ab"
Error: nu::parser::unexpected_eof
× Unexpected end of code.
╭─[source:1:6]
1 │ r#'ab
╰────
```
## After
```
> nu -c "'ab"
Error: nu::parser::unexpected_eof
× Unexpected end of code.
╭─[source:1:3]
1 │ 'ab
· ┬
· ╰── expected closing '
╰────
> ./target/debug/nu -c "r#'ab"
Error: nu::parser::unexpected_eof
× Unexpected end of code.
╭─[source:1:5]
1 │ r#'ab
· ┬
· ╰── expected closing '#
╰────
```
# Tests + Formatting
Added some tests for incomplete string.
---------
Co-authored-by: Ian Manske <ian.manske@pm.me>
# Description
Fixes: #12744
This pr is moving raw string lex logic into `lex_item` function, so we
can use raw string inside subexpression, list, closure.
```nushell
> [r#'abc'#]
╭───┬─────╮
│ 0 │ abc │
╰───┴─────╯
> (r#'abc'#)
abc
> do {r#'aa'#}
aa
```
# Tests + Formatting
Done
# After Submitting
NaN
# Description
This PR adds raw string support by using `r#` at the beginning of single
quoted strings and `#` at the end.
Notice that escapes do not process, even within single quotes,
parentheses don't mean anything, $variables don't mean anything. It's
just a string.
```nushell
❯ echo r#'one\ntwo (blah) ($var)'#
one\ntwo (blah) ($var)
```
Notice how they work without `echo` or `print` and how they work without
carriage returns.
```nushell
❯ r#'adsfa'#
adsfa
❯ r##"asdfa'@qpejq'##
asdfa'@qpejq
❯ r#'asdfasdfasf
∙ foqwejfqo@'23rfjqf'#
```
They also have a special configurable color in the repl. (use single
quotes though)

They should work like rust raw literals and allow `r##`, `r###`,
`r####`, etc, to help with having one or many `#`'s in the middle of
your raw-string.
They should work with `let` as well.
```nushell
r#'some\nraw\nstring'# | str upcase
```
closes https://github.com/nushell/nushell/issues/5091
# User-Facing Changes
<!-- List of all changes that impact the user experience here. This
helps us keep track of breaking changes. -->
# Tests + Formatting
<!--
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect -A clippy::result_large_err` to check that
you're using the standard code style
- `cargo test --workspace` to check that all tests pass
- `cargo run -- -c "use std testing; testing run-tests --path
crates/nu-std"` to run the tests for the standard library
> **Note**
> from `nushell` you can also use the `toolkit` as follows
> ```bash
> use toolkit.nu # or use an `env_change` hook to activate it
automatically
> toolkit check pr
> ```
-->
# After Submitting
<!-- If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
-->
---------
Co-authored-by: WindSoilder <WindSoilder@outlook.com>
Co-authored-by: Ian Manske <ian.manske@pm.me>
- Fixes issue #11982
# Description
Expressions with unbalanced parenthesis [excess closing ')' parenthesis]
will throw an error instead of interpreting ')' as a string.
Solved he same way as closing braces '}' are handled.


# User-Facing Changes
- Trailing closing parentheses ')' which do not match the number of
opening parentheses '(' will lead to a parse error.
- From what I have found in the documentation this is the intended
behavior, thus no documentation has been updated on my part
# Tests + Formatting
- Two tests added in src/tests/test_parser.rs
- All previous tests are still passing
- cargo fmt, clippy and test have been run
Unable to get the following command run
- `cargo run -- -c "use std testing; testing run-tests --path
crates/nu-std"` to run the tests for the standard library

---------
Co-authored-by: Noak Jönsson <noakj@kth.se>
# Description
Close: #9673Close: #8277Close: #10944
This pr introduces the following syntax:
1. `e>|`, pipe stderr to next command. Example: `$env.FOO=bar nu
--testbin echo_env_stderr FOO e>| str length`
2. `o+e>|` and `e+o>|`, pipe both stdout and stderr to next command,
example: `$env.FOO=bar nu --testbin echo_env_mixed out-err FOO FOO e+o>|
str length`
Note: it only works for external commands. ~There is no different for
internal commands, that is, the following three commands do the same
things:~ Edit: it raises errors if we want to pipes for internal
commands
```
❯ ls e>| str length
Error: × `e>|` only works with external streams
╭─[entry #1:1:1]
1 │ ls e>| str length
· ─┬─
· ╰── `e>|` only works on external streams
╰────
❯ ls e+o>| str length
Error: × `o+e>|` only works with external streams
╭─[entry #2:1:1]
1 │ ls e+o>| str length
· ──┬──
· ╰── `o+e>|` only works on external streams
╰────
```
This can help us to avoid some strange issues like the following:
`$env.FOO=bar (nu --testbin echo_env_stderr FOO) e>| str length`
Which is hard to understand and hard to explain to users.
# User-Facing Changes
Nan
# Tests + Formatting
To be done
# After Submitting
Maybe update documentation about these syntax.
# Description
Close: #10278
This pr introduces `o>>`, `e>>`, `o+e>>` to allow redirection to append
to a file.
Examples:
```nushell
echo abc o>> a.txt
echo abc o>> a.txt
cat asdf e>> a.txt
cat asdf e>> a.txt
cat asdf o+e>> a.txt
```
~~TODO:~~
~~1. currently internal commands with `o+e>` redirect to a variable is
broken: `let x = "a.txt"; echo abc o+e> $x`, not sure when it was
introduced...~~
~~2. redirect stdout and stderr with append mode doesn't supported yet:
`cat asdf o>>a.txt e>>b.ext`~~
~~For these 2 items, I'd like to fix them in different prs.~~
Already done in this pr
In the final match of `lex_item`, we'll return `Err(ParseError)` in rare
case, normally we'll return None.
So I think making error part mutable can reduce some code, and it's
better if we want to add more lex items.
<!--
if this PR closes one or more issues, you can automatically link the PR
with
them by using one of the [*linking
keywords*](https://docs.github.com/en/issues/tracking-your-work-with-issues/linking-a-pull-request-to-an-issue#linking-a-pull-request-to-an-issue-using-a-keyword),
e.g.
- this PR should close #xxxx
- fixes #xxxx
you can also mention related issues, PRs or discussions!
-->
# Description
- fixes: #5517
- fixes: #9250
For the following commands:
```
ls
# | le
| length
```
I found that it generates a bad lite parsing result:
```
LiteBlock {
block: [
LitePipeline {
commands: [
Command(None, LiteCommand { comments: [], parts: [Span { start: 138600, end: 138602 }] })
]
},
LitePipeline {
commands: [
Command(Some(Span { start: 138610, end: 138611 }),
LiteCommand { comments: [Span { start: 138603, end: 138609 }], parts: [Span { start: 138612, end: 138618 }] })
]
}
]
}
```
Which should contains only one `LitePipeline`, and the second
`LitePipeline` is generated because of `Eol` lex token:
```
[
Token { contents: Item, span: Span { start: 138600, end: 138602 } },
Token { contents: Eol, span: Span { start: 138602, end: 138603 } }, // it generates the second LitePipeline
Token { contents: Comment, span: Span { start: 138603, end: 138609 } },
Token { contents: Pipe, span: Span { start: 138610, end: 138611 } },
Token { contents: Item, span: Span { start: 138612, end: 138618 } }
]
```
To fix the issue, I remove the `Eol` token when we meet `Comment` right
after `Eol`, then it will generate a good LiteBlock, and everything will
work fine.
### After the fix:
Token:
```
[
Token { contents: Item, span: Span { start: 138618, end: 138620 } },
Token { contents: Comment, span: Span { start: 138622, end: 138628 } },
Token { contents: Pipe, span: Span { start: 138629, end: 138630 } },
Token { contents: Item, span: Span { start: 138631, end: 138637 } }
]
```
LiteBlock:
```
LiteBlock {
block: [
LitePipeline {
commands: [
Command(
None,
LiteCommand {
comments: [Span { start: 138622, end: 138628 }],
parts: [Span { start: 138618, end: 138620 }]
}
),
Command(
Some(Span { start: 138629, end: 138630 }),
LiteCommand { comments: [], parts: [Span { start: 138631, end: 138637 }] })] }] }
```
<!--
Thank you for improving Nushell. Please, check our [contributing
guide](../CONTRIBUTING.md) and talk to the core team before making major
changes.
Description of your pull request goes here. **Provide examples and/or
screenshots** if your changes affect the user experience.
-->
# User-Facing Changes
<!-- List of all changes that impact the user experience here. This
helps us keep track of breaking changes. -->
# Tests + Formatting
<!--
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect -A clippy::result_large_err` to check that
you're using the standard code style
- `cargo test --workspace` to check that all tests pass
- `cargo run -- crates/nu-std/tests/run.nu` to run the tests for the
standard library
> **Note**
> from `nushell` you can also use the `toolkit` as follows
> ```bash
> use toolkit.nu # or use an `env_change` hook to activate it
automatically
> toolkit check pr
> ```
-->
# After Submitting
<!-- If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
-->
# Description
This is a pretty heavy refactor of the parser to support multiple parser
errors. It has a few issues we should address before landing:
- [x] In some cases, error quality has gotten worse `1 / "bob"` for
example
- [x] if/else isn't currently parsing correctly
- probably others
# User-Facing Changes
This may have error quality degradation as we adjust to the new error
reporting mechanism.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
- `cargo run -- crates/nu-utils/standard_library/tests.nu` to run the
tests for the standard library
> **Note**
> from `nushell` you can also use the `toolkit` as follows
> ```bash
> use toolkit.nu # or use an `env_change` hook to activate it
automatically
> toolkit check pr
> ```
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
this pr refines #8270 and closes#8109
# description
examples:
the original syntax is okay
```nu
def okay [nums: list] {} # the type of list will be list<any>
```
empty annotations are allowed in any variation
the last two may be caught by a future formatter,
but do not affect `nu` code currently
```nu
def okay [nums: list<>] {} # okay
def okay [nums: list< >] {} # weird but also okay
def okay [nums: list<
>] {} # also weird but okay
```
types are allowed (See [notes](#notes) below)
```nu
def okay [nums: list<int>] {} # `test [a b c]` will throw an error
def okay [nums: list< int > {} # any amount of space within the angle brackets is okay
def err [nums: list <int>] {} # this is not okay, `nums` and `<int>` will be parsed as
# two separate params,
```
nested annotations are allowed in many variations
```nu
def okay [items: list<list<int>>] {}
def okay [items: list<list>] {}
```
any unterminated annotation is caught
```nu
Error: nu::parser::unexpected_eof
× Unexpected end of code.
╭─[source:1:1]
1 │ def err [nums: list<int] {}
· ▲
· ╰── expected closing >
╰────
```
unknown types are flagged
```nu
Error: nu::parser::unknown_type
× Unknown type.
╭─[source:1:1]
1 │ def err [nums: list<str>] {}
· ─┬─
· ╰── unknown type
╰────
Error: nu::parser::unknown_type
× Unknown type.
╭─[source:1:1]
1 │ def err [nums: list<int, string>] {}
· ─────┬─────
· ╰── unknown type
╰────
```
# notes
the error message for mismatched types in not as intuitive
```nu
Error: nu::parser::parse_mismatch
× Parse mismatch during operation.
╭─[source:1:1]
1 │ def err [nums: list<int>] {}; err [a b c]
· ┬
· ╰── expected int
╰────
```
it should be something like this
```nu
Error: nu::parser::parse_mismatch
× Parse mismatch during operation.
╭─[source:1:1]
1 │ def err [nums: list<int>] {}; err [a b c]
· ──┬──
· ╰── expected list<int>
╰────
```
this is currently not implemented
# Description
Previously `nix run nixpkgs#hello` was lexed as `Item, Item, Item,
Comment`, however, `#hello` is *not* supposed to be a comment here and
should be parsed as part of the third `Item`.
This change introduces this behavior by not interrupting the parse of
the current token upon seeing a `#`.
Thank you so much for considering this, I think many `nix` users will be
grateful for this change and I think this will lead to more adaptation
in the ecosystem.
- closes#8137 and #6335
# User-Facing Changes
- code like `somecode# bla` and `somecode#bla` will not be parsed as
`somecode, comment` but as `somecode#bla`, hence this is a breaking
change for all users who didn't put a space before a comment introducing
token (`#`)
# Tests + Formatting
I've added tests that cover this behavior in `test_lex.rs`
- [x] `cargo fmt --all -- --check` to check standard code formatting
(`cargo fmt --all` applies these changes)
- [x] `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- [x] `cargo test --workspace` to check that all tests pass
# After Submitting
> If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
I think this is expected behavior in most other shells, so the
documentation was lacking for not documenting the unexpected behavior
before and hence now is automatically more complete >D
Reverts nushell/nushell#7448
Some surprising behavior in how we do this. For example:
```
〉if (true || false) { print "yes!" } else { print "no!" }
no!
〉if (true or false) { print "yes!" } else { print "no!" }
yes!
```
This means for folks who are using the old `||`, they possibly get the
wrong answer once they upgrade. I don't think we can ship with that as
it will catch too many people by surprise and just make it easier to
write buggy code.
# Description
We got some feedback from folks used to other shells that `try/catch`
isn't quite as convenient as things like `||`. This PR adds `&&` as a
synonym for `;` and `||` as equivalent to what `try/catch` would do.
# User-Facing Changes
Adds `&&` and `||` pipeline operators.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
Adds improved errors for when a user uses a bashism that nu doesn't
support.
fixes#7237
Examples:
```
Error: nu::parser::shell_andand (link)
× The '&&' operator is not supported in Nushell
╭─[entry #1:1:1]
1 │ ls && ls
· ─┬
· ╰── instead of '&&', use ';' or 'and'
╰────
help: use ';' instead of the shell '&&', or 'and' instead of the boolean '&&'
```
```
Error: nu::parser::shell_oror (link)
× The '||' operator is not supported in Nushell
╭─[entry #8:1:1]
1 │ ls || ls
· ─┬
· ╰── instead of '||', use 'try' or 'or'
╰────
help: use 'try' instead of the shell '||', or 'or' instead of the boolean '||'
```
```
Error: nu::parser::shell_err (link)
× The '2>' shell operation is 'err>' in Nushell.
╭─[entry #9:1:1]
1 │ foo 2> bar.txt
· ─┬
· ╰── use 'err>' instead of '2>' in Nushell
╰────
```
```
Error: nu::parser::shell_outerr (link)
× The '2>&1' shell operation is 'out+err>' in Nushell.
╭─[entry #10:1:1]
1 │ foo 2>&1 bar.txt
· ──┬─
· ╰── use 'out+err>' instead of '2>&1' in Nushell
╰────
help: Nushell redirection will write all of stdout before stderr.
```
# User-Facing Changes
**BREAKING CHANGES**
This removes the `&&` and `||` operators. We previously supported by
`&&`/`and` and `||`/`or`. With this change, only `and` and `or` are
valid boolean operators.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
Also enforce this by #[non_exhaustive] span such that going forward we
cannot, in debug builds (1), construct invalid spans.
The motivation for this stems from #6431 where I've seen crashes due to
invalid slice indexing.
My hope is this will mitigate such senarios
1. https://github.com/nushell/nushell/pull/6431#issuecomment-1278147241
# Description
(description of your pull request here)
# Tests
Make sure you've done the following:
- [ ] Add tests that cover your changes, either in the command examples,
the crate/tests folder, or in the /tests folder.
- [ ] Try to think about corner cases and various ways how your changes
could break. Cover them with tests.
- [ ] If adding tests is not possible, please document in the PR body a
minimal example with steps on how to reproduce so one can verify your
change works.
Make sure you've run and fixed any issues with these commands:
- [x] `cargo fmt --all -- --check` to check standard code formatting
(`cargo fmt --all` applies these changes)
- [ ] `cargo clippy --workspace --features=extra -- -D warnings -D
clippy::unwrap_used -A clippy::needless_collect` to check that you're
using the standard code style
- [ ] `cargo test --workspace --features=extra` to check that all the
tests pass
# Documentation
- [ ] If your PR touches a user-facing nushell feature then make sure
that there is an entry in the documentation
(https://github.com/nushell/nushell.github.io) for the feature, and
update it if necessary.
This adds new pipeline connectors called out> and err> which redirect either stdout or stderr to a file. You can also use out+err> (or err+out>) to redirect both streams into a file.