2024-05-13 15:37:53 +02:00
|
|
|
use crate::repl::tests::{fail_test, run_test, run_test_contains, run_test_with_env, TestResult};
|
2024-04-23 00:10:35 +02:00
|
|
|
use nu_test_support::{nu, nu_repl_code};
|
2022-04-11 20:18:46 +02:00
|
|
|
use std::collections::HashMap;
|
2021-12-25 20:39:42 +01:00
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn env_shorthand() -> TestResult {
|
2022-03-03 01:55:03 +01:00
|
|
|
run_test("FOO=BAR if false { 3 } else { 4 }", "4")
|
2021-12-25 20:39:42 +01:00
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn subcommand() -> TestResult {
|
|
|
|
run_test("def foo [] {}; def \"foo bar\" [] {3}; foo bar", "3")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn alias_1() -> TestResult {
|
|
|
|
run_test("def foo [$x] { $x + 10 }; alias f = foo; f 100", "110")
|
|
|
|
}
|
|
|
|
|
2023-01-15 16:03:57 +01:00
|
|
|
#[test]
|
|
|
|
fn ints_with_underscores() -> TestResult {
|
|
|
|
run_test("1_0000_0000_0000 + 10", "1000000000010")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn floats_with_underscores() -> TestResult {
|
|
|
|
run_test("3.1415_9265_3589_793 * 2", "6.283185307179586")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn bin_ints_with_underscores() -> TestResult {
|
|
|
|
run_test("0b_10100_11101_10010", "21426")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn oct_ints_with_underscores() -> TestResult {
|
|
|
|
run_test("0o2443_6442_7652_0044", "90422533333028")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn hex_ints_with_underscores() -> TestResult {
|
|
|
|
run_test("0x68__9d__6a", "6856042")
|
|
|
|
}
|
|
|
|
|
2021-12-25 20:39:42 +01:00
|
|
|
#[test]
|
|
|
|
fn alias_2() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
"def foo [$x $y] { $x + $y + 10 }; alias f = foo 33; f 100",
|
|
|
|
"143",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn alias_2_multi_word() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"def "foo bar" [$x $y] { $x + $y + 10 }; alias f = foo bar 33; f 100"#,
|
|
|
|
"143",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
Re-implement aliases (#8123)
# Description
This PR adds an alternative alias implementation. Old aliases still work
but you need to use `old-alias` instead of `alias`.
Instead of replacing spans in the original code and re-parsing, which
proved to be extremely error-prone and a constant source of panics, the
new implementation creates a new command that references the old
command. Consider the new alias defined as `alias ll = ls -l`. The
parser creates a new command called `ll` and remembers that it is
actually a `ls` command called with the `-l` flag. Then, when the parser
sees the `ll` command, it will translate it to `ls -l` and passes to it
any parameters that were passed to the call to `ll`. It works quite
similar to how known externals defined with `extern` are implemented.
The new alias implementation should work the same way as the old
aliases, including exporting from modules, referencing both known and
unknown externals. It seems to preserve custom completions and pipeline
metadata. It is quite robust in most cases but there are some rough
edges (see later).
Fixes https://github.com/nushell/nushell/issues/7648,
https://github.com/nushell/nushell/issues/8026,
https://github.com/nushell/nushell/issues/7512,
https://github.com/nushell/nushell/issues/5780,
https://github.com/nushell/nushell/issues/7754
No effect: https://github.com/nushell/nushell/issues/8122 (we might
revisit the completions code after this PR)
Should use custom command instead:
https://github.com/nushell/nushell/issues/6048
# User-Facing Changes
Since aliases are now basically commands, it has some new implications:
1. `alias spam = "spam"` (requires command call)
* **workaround**: use `alias spam = echo "spam"`
2. `def foo [] { 'foo' }; alias foo = ls -l` (foo defined more than
once)
* **workaround**: use different name (commands also have this
limitation)
4. `alias ls = (ls | sort-by type name -i)`
* **workaround**: Use custom command. _The common issue with this is
that it is currently not easy to pass flags through custom commands and
command referencing itself will lead to stack overflow. Both of these
issues are meant to be addressed._
5. TODO: Help messages, `which` command, `$nu.scope.aliases`, etc.
* Should we treat the aliases as commands or should they be separated
from regular commands?
6. Needs better error message and syntax highlight for recursed alias
(`alias f = f`)
7. Can't create alias with the same name as existing command (`alias ls
= ls -a`)
* Might be possible to add support for it (not 100% sure)
8. Standalone `alias` doesn't list aliases anymore
9. Can't alias parser keywords (e.g., stuff like `alias ou = overlay
use` won't work)
* TODO: Needs a better error message when attempting to do so
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
2023-02-27 08:44:05 +01:00
|
|
|
#[ignore = "TODO: Allow alias to alias existing command with the same name"]
|
2022-02-15 23:36:24 +01:00
|
|
|
#[test]
|
|
|
|
fn alias_recursion() -> TestResult {
|
Re-implement aliases (#8123)
# Description
This PR adds an alternative alias implementation. Old aliases still work
but you need to use `old-alias` instead of `alias`.
Instead of replacing spans in the original code and re-parsing, which
proved to be extremely error-prone and a constant source of panics, the
new implementation creates a new command that references the old
command. Consider the new alias defined as `alias ll = ls -l`. The
parser creates a new command called `ll` and remembers that it is
actually a `ls` command called with the `-l` flag. Then, when the parser
sees the `ll` command, it will translate it to `ls -l` and passes to it
any parameters that were passed to the call to `ll`. It works quite
similar to how known externals defined with `extern` are implemented.
The new alias implementation should work the same way as the old
aliases, including exporting from modules, referencing both known and
unknown externals. It seems to preserve custom completions and pipeline
metadata. It is quite robust in most cases but there are some rough
edges (see later).
Fixes https://github.com/nushell/nushell/issues/7648,
https://github.com/nushell/nushell/issues/8026,
https://github.com/nushell/nushell/issues/7512,
https://github.com/nushell/nushell/issues/5780,
https://github.com/nushell/nushell/issues/7754
No effect: https://github.com/nushell/nushell/issues/8122 (we might
revisit the completions code after this PR)
Should use custom command instead:
https://github.com/nushell/nushell/issues/6048
# User-Facing Changes
Since aliases are now basically commands, it has some new implications:
1. `alias spam = "spam"` (requires command call)
* **workaround**: use `alias spam = echo "spam"`
2. `def foo [] { 'foo' }; alias foo = ls -l` (foo defined more than
once)
* **workaround**: use different name (commands also have this
limitation)
4. `alias ls = (ls | sort-by type name -i)`
* **workaround**: Use custom command. _The common issue with this is
that it is currently not easy to pass flags through custom commands and
command referencing itself will lead to stack overflow. Both of these
issues are meant to be addressed._
5. TODO: Help messages, `which` command, `$nu.scope.aliases`, etc.
* Should we treat the aliases as commands or should they be separated
from regular commands?
6. Needs better error message and syntax highlight for recursed alias
(`alias f = f`)
7. Can't create alias with the same name as existing command (`alias ls
= ls -a`)
* Might be possible to add support for it (not 100% sure)
8. Standalone `alias` doesn't list aliases anymore
9. Can't alias parser keywords (e.g., stuff like `alias ou = overlay
use` won't work)
* TODO: Needs a better error message when attempting to do so
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
2023-02-27 08:44:05 +01:00
|
|
|
run_test_contains(r#"alias ls = ls -a; ls"#, " ")
|
2022-02-15 23:36:24 +01:00
|
|
|
}
|
|
|
|
|
2021-12-25 20:39:42 +01:00
|
|
|
#[test]
|
|
|
|
fn block_param1() -> TestResult {
|
2022-02-17 12:40:24 +01:00
|
|
|
run_test("[3] | each { |it| $it + 10 } | get 0", "13")
|
2021-12-25 20:39:42 +01:00
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn block_param2() -> TestResult {
|
|
|
|
run_test("[3] | each { |y| $y + 10 } | get 0", "13")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn block_param3_list_iteration() -> TestResult {
|
2022-02-17 12:40:24 +01:00
|
|
|
run_test("[1,2,3] | each { |it| $it + 10 } | get 1", "12")
|
2021-12-25 20:39:42 +01:00
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn block_param4_list_iteration() -> TestResult {
|
|
|
|
run_test("[1,2,3] | each { |y| $y + 10 } | get 2", "13")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn range_iteration1() -> TestResult {
|
|
|
|
run_test("1..4 | each { |y| $y + 10 } | get 0", "11")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn range_iteration2() -> TestResult {
|
|
|
|
run_test("4..1 | each { |y| $y + 100 } | get 3", "101")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn simple_value_iteration() -> TestResult {
|
2022-02-17 12:40:24 +01:00
|
|
|
run_test("4 | each { |it| $it + 10 }", "14")
|
2021-12-25 20:39:42 +01:00
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn comment_multiline() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"def foo [] {
|
|
|
|
let x = 1 + 2 # comment
|
|
|
|
let y = 3 + 4 # another comment
|
|
|
|
$x + $y
|
|
|
|
}; foo"#,
|
|
|
|
"10",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn comment_skipping_1() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"let x = {
|
|
|
|
y: 20
|
|
|
|
# foo
|
|
|
|
}; $x.y"#,
|
|
|
|
"20",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn comment_skipping_2() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"let x = {
|
|
|
|
y: 20
|
|
|
|
# foo
|
|
|
|
z: 40
|
|
|
|
}; $x.z"#,
|
|
|
|
"40",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
2023-08-30 20:24:13 +02:00
|
|
|
#[test]
|
|
|
|
fn comment_skipping_in_pipeline_1() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"[1,2,3] | #comment
|
|
|
|
each { |$it| $it + 2 } | # foo
|
|
|
|
math sum #bar"#,
|
|
|
|
"12",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn comment_skipping_in_pipeline_2() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"[1,2,3] #comment
|
|
|
|
| #comment2
|
|
|
|
each { |$it| $it + 2 } #foo
|
|
|
|
| # bar
|
|
|
|
math sum #baz"#,
|
|
|
|
"12",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn comment_skipping_in_pipeline_3() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"[1,2,3] | #comment
|
|
|
|
#comment2
|
|
|
|
each { |$it| $it + 2 } #foo
|
|
|
|
| # bar
|
|
|
|
#baz
|
|
|
|
math sum #foobar"#,
|
|
|
|
"12",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
2021-12-25 20:39:42 +01:00
|
|
|
#[test]
|
|
|
|
fn bad_var_name() -> TestResult {
|
|
|
|
fail_test(r#"let $"foo bar" = 4"#, "can't contain")
|
|
|
|
}
|
|
|
|
|
2022-07-27 04:08:54 +02:00
|
|
|
#[test]
|
|
|
|
fn bad_var_name2() -> TestResult {
|
|
|
|
fail_test(r#"let $foo-bar = 4"#, "valid variable")
|
|
|
|
}
|
|
|
|
|
2023-08-01 18:21:40 +02:00
|
|
|
#[test]
|
|
|
|
fn assignment_with_no_var() -> TestResult {
|
|
|
|
let cases = [
|
|
|
|
"let = if $",
|
|
|
|
"mut = if $",
|
|
|
|
"const = if $",
|
|
|
|
"let = 'foo' | $in; $x | describe",
|
|
|
|
"mut = 'foo' | $in; $x | describe",
|
|
|
|
];
|
|
|
|
|
|
|
|
let expected = "valid variable";
|
|
|
|
|
|
|
|
for case in cases {
|
|
|
|
fail_test(case, expected)?;
|
|
|
|
}
|
|
|
|
|
|
|
|
Ok(())
|
|
|
|
}
|
|
|
|
|
2021-12-25 20:39:42 +01:00
|
|
|
#[test]
|
|
|
|
fn long_flag() -> TestResult {
|
|
|
|
run_test(
|
2023-02-02 23:59:58 +01:00
|
|
|
r#"([a, b, c] | enumerate | each --keep-empty { |e| if $e.index != 1 { 100 }}).1 | to nuon"#,
|
|
|
|
"null",
|
2021-12-25 20:39:42 +01:00
|
|
|
)
|
|
|
|
}
|
2021-12-27 04:04:22 +01:00
|
|
|
|
2021-12-27 21:04:48 +01:00
|
|
|
#[test]
|
|
|
|
fn for_in_missing_var_name() -> TestResult {
|
|
|
|
fail_test("for in", "missing")
|
|
|
|
}
|
2022-01-02 06:27:58 +01:00
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn multiline_pipe_in_block() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"do {
|
|
|
|
echo hello |
|
|
|
|
str length
|
|
|
|
}"#,
|
|
|
|
"5",
|
|
|
|
)
|
|
|
|
}
|
2022-01-06 22:06:54 +01:00
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn bad_short_flag() -> TestResult {
|
|
|
|
fail_test(r#"def foo3 [-l?:int] { $l }"#, "short flag")
|
|
|
|
}
|
2022-01-10 03:52:01 +01:00
|
|
|
|
2022-01-19 15:58:12 +01:00
|
|
|
#[test]
|
|
|
|
fn quotes_with_equals() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"let query_prefix = "https://api.github.com/search/issues?q=repo:nushell/"; $query_prefix"#,
|
|
|
|
"https://api.github.com/search/issues?q=repo:nushell/",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn string_interp_with_equals() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"let query_prefix = $"https://api.github.com/search/issues?q=repo:nushell/"; $query_prefix"#,
|
|
|
|
"https://api.github.com/search/issues?q=repo:nushell/",
|
|
|
|
)
|
|
|
|
}
|
2022-01-21 17:39:55 +01:00
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn recursive_parse() -> TestResult {
|
|
|
|
run_test(r#"def c [] { c }; echo done"#, "done")
|
|
|
|
}
|
2022-01-22 19:24:47 +01:00
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn commands_have_usage() -> TestResult {
|
|
|
|
run_test_contains(
|
|
|
|
r#"
|
|
|
|
# This is a test
|
|
|
|
#
|
|
|
|
# To see if I have cool usage
|
|
|
|
def foo [] {}
|
|
|
|
help foo"#,
|
|
|
|
"cool usage",
|
|
|
|
)
|
|
|
|
}
|
2022-01-27 02:20:12 +01:00
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn equals_separates_long_flag() -> TestResult {
|
2022-11-09 23:19:02 +01:00
|
|
|
run_test(
|
A `fill` command to replace `str lpad` and `str rpad` (#7846)
# Description
The point of this command is to allow you to be able to format ints,
floats, filesizes, and strings with an alignment, padding, and a fill
character, as strings. It's meant to take the place of `str lpad` and
`str rpad`.
```
> help fill
Fill and Align
Search terms: display, render, format, pad, align
Usage:
> fill {flags}
Flags:
-h, --help - Display the help message for this command
-w, --width <Int> - The width of the output. Defaults to 1
-a, --alignment <String> - The alignment of the output. Defaults to Left (Left(l), Right(r), Center(c/m), MiddleRight(cr/mr))
-c, --character <String> - The character to fill with. Defaults to ' ' (space)
Signatures:
<number> | fill -> <string>
<string> | fill -> <string>
Examples:
Fill a string on the left side to a width of 15 with the character '─'
> 'nushell' | fill -a l -c '─' -w 15
Fill a string on the right side to a width of 15 with the character '─'
> 'nushell' | fill -a r -c '─' -w 15
Fill a string on both sides to a width of 15 with the character '─'
> 'nushell' | fill -a m -c '─' -w 15
Fill a number on the left side to a width of 5 with the character '0'
> 1 | fill --alignment right --character 0 --width 5
Fill a filesize on the left side to a width of 5 with the character '0'
> 1kib | fill --alignment middle --character 0 --width 10
```
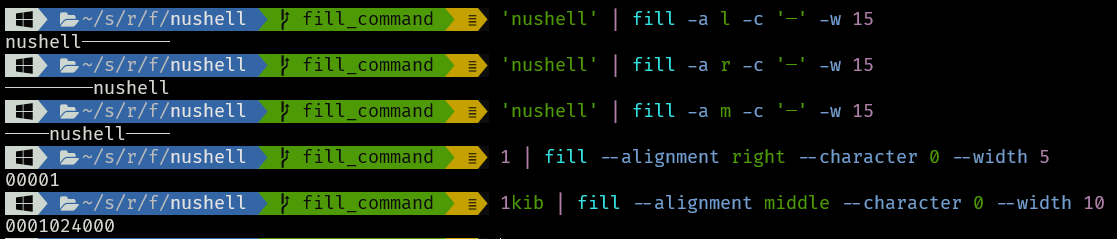
# User-Facing Changes
Deprecated `str lpad` and `str rpad`.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
2023-02-09 21:56:52 +01:00
|
|
|
r#"'nushell' | fill --alignment right --width=10 --character='-'"#,
|
2022-11-09 23:19:02 +01:00
|
|
|
"---nushell",
|
|
|
|
)
|
2022-01-27 02:20:12 +01:00
|
|
|
}
|
2022-02-09 19:41:41 +01:00
|
|
|
|
|
|
|
#[test]
|
2023-06-30 21:57:51 +02:00
|
|
|
fn assign_expressions() -> TestResult {
|
2022-04-11 20:18:46 +02:00
|
|
|
let env = HashMap::from([("VENV_OLD_PATH", "Foobar"), ("Path", "Quux")]);
|
|
|
|
run_test_with_env(
|
2023-06-30 21:57:51 +02:00
|
|
|
r#"$env.Path = (if ($env | columns | "VENV_OLD_PATH" in $in) { $env.VENV_OLD_PATH } else { $env.Path }); echo $env.Path"#,
|
2022-04-11 20:18:46 +02:00
|
|
|
"Foobar",
|
|
|
|
&env,
|
2022-02-09 19:41:41 +01:00
|
|
|
)
|
|
|
|
}
|
2022-02-10 17:09:08 +01:00
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn string_interpolation_paren_test() -> TestResult {
|
|
|
|
run_test(r#"$"('(')(')')""#, "()")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn string_interpolation_paren_test2() -> TestResult {
|
|
|
|
run_test(r#"$"('(')test(')')""#, "(test)")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn string_interpolation_paren_test3() -> TestResult {
|
|
|
|
run_test(r#"$"('(')("test")test(')')""#, "(testtest)")
|
|
|
|
}
|
2022-02-11 00:15:15 +01:00
|
|
|
|
2022-03-15 17:09:30 +01:00
|
|
|
#[test]
|
|
|
|
fn string_interpolation_escaping() -> TestResult {
|
|
|
|
run_test(r#"$"hello\nworld" | lines | length"#, "2")
|
|
|
|
}
|
|
|
|
|
2022-02-11 00:15:15 +01:00
|
|
|
#[test]
|
|
|
|
fn capture_multiple_commands() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"
|
|
|
|
let CONST_A = 'Hello'
|
|
|
|
|
|
|
|
def 'say-hi' [] {
|
|
|
|
echo (call-me)
|
|
|
|
}
|
|
|
|
|
|
|
|
def 'call-me' [] {
|
|
|
|
echo $CONST_A
|
|
|
|
}
|
|
|
|
|
2022-09-11 10:48:27 +02:00
|
|
|
[(say-hi) (call-me)] | str join
|
2022-02-21 14:38:15 +01:00
|
|
|
|
2022-02-11 00:15:15 +01:00
|
|
|
"#,
|
|
|
|
"HelloHello",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn capture_multiple_commands2() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"
|
|
|
|
let CONST_A = 'Hello'
|
|
|
|
|
|
|
|
def 'call-me' [] {
|
|
|
|
echo $CONST_A
|
|
|
|
}
|
|
|
|
|
|
|
|
def 'say-hi' [] {
|
|
|
|
echo (call-me)
|
|
|
|
}
|
|
|
|
|
2022-09-11 10:48:27 +02:00
|
|
|
[(say-hi) (call-me)] | str join
|
2022-02-21 14:38:15 +01:00
|
|
|
|
2022-02-11 00:15:15 +01:00
|
|
|
"#,
|
|
|
|
"HelloHello",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn capture_multiple_commands3() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"
|
|
|
|
let CONST_A = 'Hello'
|
|
|
|
|
|
|
|
def 'say-hi' [] {
|
|
|
|
echo (call-me)
|
|
|
|
}
|
|
|
|
|
|
|
|
def 'call-me' [] {
|
|
|
|
echo $CONST_A
|
|
|
|
}
|
|
|
|
|
2022-09-11 10:48:27 +02:00
|
|
|
[(call-me) (say-hi)] | str join
|
2022-02-21 14:38:15 +01:00
|
|
|
|
2022-02-11 00:15:15 +01:00
|
|
|
"#,
|
|
|
|
"HelloHello",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn capture_multiple_commands4() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"
|
|
|
|
let CONST_A = 'Hello'
|
|
|
|
|
|
|
|
def 'call-me' [] {
|
|
|
|
echo $CONST_A
|
|
|
|
}
|
|
|
|
|
|
|
|
def 'say-hi' [] {
|
|
|
|
echo (call-me)
|
|
|
|
}
|
|
|
|
|
2022-09-11 10:48:27 +02:00
|
|
|
[(call-me) (say-hi)] | str join
|
2022-02-21 14:38:15 +01:00
|
|
|
|
2022-02-11 00:15:15 +01:00
|
|
|
"#,
|
|
|
|
"HelloHello",
|
|
|
|
)
|
|
|
|
}
|
2022-02-11 13:37:10 +01:00
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn capture_row_condition() -> TestResult {
|
|
|
|
run_test(
|
2022-09-11 10:48:27 +02:00
|
|
|
r#"let name = "foo"; [foo] | where $'($name)' =~ $it | str join"#,
|
2022-02-11 13:37:10 +01:00
|
|
|
"foo",
|
|
|
|
)
|
|
|
|
}
|
2022-02-14 18:33:47 +01:00
|
|
|
|
2022-04-01 20:35:46 +02:00
|
|
|
#[test]
|
|
|
|
fn starts_with_operator_succeeds() -> TestResult {
|
|
|
|
run_test(
|
2022-09-11 10:48:27 +02:00
|
|
|
r#"[Moe Larry Curly] | where $it starts-with L | str join"#,
|
2022-04-01 20:35:46 +02:00
|
|
|
"Larry",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
2022-05-02 10:02:38 +02:00
|
|
|
#[test]
|
|
|
|
fn ends_with_operator_succeeds() -> TestResult {
|
|
|
|
run_test(
|
2022-09-11 10:48:27 +02:00
|
|
|
r#"[Moe Larry Curly] | where $it ends-with ly | str join"#,
|
2022-05-02 10:02:38 +02:00
|
|
|
"Curly",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
2022-02-14 18:33:47 +01:00
|
|
|
#[test]
|
|
|
|
fn proper_missing_param() -> TestResult {
|
|
|
|
fail_test(r#"def foo [x y z w] { }; foo a b c"#, "missing w")
|
|
|
|
}
|
2022-02-17 12:40:24 +01:00
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn block_arity_check1() -> TestResult {
|
2024-04-25 22:47:30 +02:00
|
|
|
fail_test(r#"ls | each { |x, y| 1}"#, "expected 1 closure parameter")
|
2022-02-17 12:40:24 +01:00
|
|
|
}
|
2022-03-03 19:14:03 +01:00
|
|
|
|
2023-01-28 21:25:53 +01:00
|
|
|
// deprecating former support for escapes like `/uNNNN`, dropping test.
|
2022-03-03 19:14:03 +01:00
|
|
|
#[test]
|
2023-01-28 21:25:53 +01:00
|
|
|
fn string_escape_unicode_extended() -> TestResult {
|
|
|
|
run_test(r#""\u{015B}\u{1f10b}""#, "ś🄋")
|
2022-03-03 19:14:03 +01:00
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn string_escape_interpolation() -> TestResult {
|
2023-01-28 21:25:53 +01:00
|
|
|
run_test(r#"$"\u{015B}(char hamburger)abc""#, "ś≡abc")
|
2022-03-03 19:14:03 +01:00
|
|
|
}
|
2022-03-07 17:44:27 +01:00
|
|
|
|
2022-03-27 00:52:09 +01:00
|
|
|
#[test]
|
|
|
|
fn string_escape_interpolation2() -> TestResult {
|
|
|
|
run_test(r#"$"2 + 2 is \(2 + 2)""#, "2 + 2 is (2 + 2)")
|
|
|
|
}
|
|
|
|
|
2022-03-07 17:44:27 +01:00
|
|
|
#[test]
|
|
|
|
fn proper_rest_types() -> TestResult {
|
|
|
|
run_test(
|
2023-09-23 10:20:48 +02:00
|
|
|
r#"def foo [--verbose(-v), # my test flag
|
2022-03-07 17:44:27 +01:00
|
|
|
...rest: int # my rest comment
|
|
|
|
] { if $verbose { print "verbose!" } else { print "not verbose!" } }; foo"#,
|
|
|
|
"not verbose!",
|
|
|
|
)
|
|
|
|
}
|
2022-04-03 00:41:36 +02:00
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn single_value_row_condition() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"[[a, b]; [true, false], [true, true]] | where a | length"#,
|
|
|
|
"2",
|
|
|
|
)
|
|
|
|
}
|
2022-04-06 21:10:25 +02:00
|
|
|
|
Fix exponential parser time on sequence of [[[[ (#10439)
<!--
if this PR closes one or more issues, you can automatically link the PR
with
them by using one of the [*linking
keywords*](https://docs.github.com/en/issues/tracking-your-work-with-issues/linking-a-pull-request-to-an-issue#linking-a-pull-request-to-an-issue-using-a-keyword),
e.g.
- this PR should close #xxxx
- fixes #xxxx
you can also mention related issues, PRs or discussions!
-->
# Description
<!--
Thank you for improving Nushell. Please, check our [contributing
guide](../CONTRIBUTING.md) and talk to the core team before making major
changes.
Description of your pull request goes here. **Provide examples and/or
screenshots** if your changes affect the user experience.
-->
Before this change, parsing `[[[[[[[[[[[[[[[[[[[[[[` would cause nushell
to consume several gigabytes of memory, now it should be linear in time.
The old code first tried parsing the head of the table as a list and
then after that it checked if it got more arguments. If it didn't, it
throws away the previous result and tries to parse the whole thing as a
list, which means we call `parse_list_expression` twice for each call to
`parse_table_expression`, resulting in the exponential growth
The fix is to simply check that we have all the arguments we need before
parsing the head of the table, so we know that we will either call
parse_list_expression only on sub-expressions or on the whole thing,
never both.
Fixes #10438
# User-Facing Changes
Should give a noticable speedup when typing a sequence of `[[[[[[` open
brackets
<!-- List of all changes that impact the user experience here. This
helps us keep track of breaking changes. -->
# Tests + Formatting
I would like to add tests, but I'm not sure how to do that without
crashing CI with OOM on regression
- [x] Don't forget to add tests that cover your changes.
- [x] `cargo fmt --all -- --check` to check standard code formatting
(`cargo fmt --all` applies these changes)
- [x] `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used`
to check that you're using the standard code style
- [x] `cargo test --workspace` to check that all tests pass (on Windows
make sure to [enable developer
mode](https://learn.microsoft.com/en-us/windows/apps/get-started/developer-mode-features-and-debugging))
- [x] `cargo run -- -c "use std testing; testing run-tests --path
crates/nu-std"` to run the tests for the standard library
<!--
> **Note**
> from `nushell` you can also use the `toolkit` as follows
> ```bash
> use toolkit.nu # or use an `env_change` hook to activate it
automatically
> toolkit check pr
> ```
-->
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
2023-09-20 17:53:48 +02:00
|
|
|
#[test]
|
|
|
|
fn performance_nested_lists() -> TestResult {
|
|
|
|
// Parser used to be exponential on deeply nested lists
|
|
|
|
// TODO: Add a timeout
|
|
|
|
fail_test(r#"[[[[[[[[[[[[[[[[[[[[[[[[[[[["#, "Unexpected end of code")
|
|
|
|
}
|
|
|
|
|
2022-04-06 21:10:25 +02:00
|
|
|
#[test]
|
|
|
|
fn unary_not_1() -> TestResult {
|
|
|
|
run_test(r#"not false"#, "true")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn unary_not_2() -> TestResult {
|
|
|
|
run_test(r#"not (false)"#, "true")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn unary_not_3() -> TestResult {
|
|
|
|
run_test(r#"(not false)"#, "true")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn unary_not_4() -> TestResult {
|
|
|
|
run_test(r#"if not false { "hello" } else { "world" }"#, "hello")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn unary_not_5() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"if not not not not false { "hello" } else { "world" }"#,
|
|
|
|
"world",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn unary_not_6() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"[[name, present]; [abc, true], [def, false]] | where not present | get name.0"#,
|
|
|
|
"def",
|
|
|
|
)
|
|
|
|
}
|
2022-04-07 08:02:28 +02:00
|
|
|
|
allow comment in multiple line pipeline (#9436)
<!--
if this PR closes one or more issues, you can automatically link the PR
with
them by using one of the [*linking
keywords*](https://docs.github.com/en/issues/tracking-your-work-with-issues/linking-a-pull-request-to-an-issue#linking-a-pull-request-to-an-issue-using-a-keyword),
e.g.
- this PR should close #xxxx
- fixes #xxxx
you can also mention related issues, PRs or discussions!
-->
# Description
- fixes: #5517
- fixes: #9250
For the following commands:
```
ls
# | le
| length
```
I found that it generates a bad lite parsing result:
```
LiteBlock {
block: [
LitePipeline {
commands: [
Command(None, LiteCommand { comments: [], parts: [Span { start: 138600, end: 138602 }] })
]
},
LitePipeline {
commands: [
Command(Some(Span { start: 138610, end: 138611 }),
LiteCommand { comments: [Span { start: 138603, end: 138609 }], parts: [Span { start: 138612, end: 138618 }] })
]
}
]
}
```
Which should contains only one `LitePipeline`, and the second
`LitePipeline` is generated because of `Eol` lex token:
```
[
Token { contents: Item, span: Span { start: 138600, end: 138602 } },
Token { contents: Eol, span: Span { start: 138602, end: 138603 } }, // it generates the second LitePipeline
Token { contents: Comment, span: Span { start: 138603, end: 138609 } },
Token { contents: Pipe, span: Span { start: 138610, end: 138611 } },
Token { contents: Item, span: Span { start: 138612, end: 138618 } }
]
```
To fix the issue, I remove the `Eol` token when we meet `Comment` right
after `Eol`, then it will generate a good LiteBlock, and everything will
work fine.
### After the fix:
Token:
```
[
Token { contents: Item, span: Span { start: 138618, end: 138620 } },
Token { contents: Comment, span: Span { start: 138622, end: 138628 } },
Token { contents: Pipe, span: Span { start: 138629, end: 138630 } },
Token { contents: Item, span: Span { start: 138631, end: 138637 } }
]
```
LiteBlock:
```
LiteBlock {
block: [
LitePipeline {
commands: [
Command(
None,
LiteCommand {
comments: [Span { start: 138622, end: 138628 }],
parts: [Span { start: 138618, end: 138620 }]
}
),
Command(
Some(Span { start: 138629, end: 138630 }),
LiteCommand { comments: [], parts: [Span { start: 138631, end: 138637 }] })] }] }
```
<!--
Thank you for improving Nushell. Please, check our [contributing
guide](../CONTRIBUTING.md) and talk to the core team before making major
changes.
Description of your pull request goes here. **Provide examples and/or
screenshots** if your changes affect the user experience.
-->
# User-Facing Changes
<!-- List of all changes that impact the user experience here. This
helps us keep track of breaking changes. -->
# Tests + Formatting
<!--
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect -A clippy::result_large_err` to check that
you're using the standard code style
- `cargo test --workspace` to check that all tests pass
- `cargo run -- crates/nu-std/tests/run.nu` to run the tests for the
standard library
> **Note**
> from `nushell` you can also use the `toolkit` as follows
> ```bash
> use toolkit.nu # or use an `env_change` hook to activate it
automatically
> toolkit check pr
> ```
-->
# After Submitting
<!-- If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
-->
2023-06-15 13:11:42 +02:00
|
|
|
#[test]
|
|
|
|
fn comment_in_multiple_pipelines() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"[[name, present]; [abc, true], [def, false]]
|
|
|
|
# | where not present
|
|
|
|
| get name.0"#,
|
|
|
|
"abc",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
2022-04-07 08:02:28 +02:00
|
|
|
#[test]
|
|
|
|
fn date_literal() -> TestResult {
|
|
|
|
run_test(r#"2022-09-10 | date to-record | get day"#, "10")
|
|
|
|
}
|
2022-04-22 21:14:31 +02:00
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn and_and_or() -> TestResult {
|
|
|
|
run_test(r#"true and false or true"#, "true")
|
|
|
|
}
|
2022-11-26 17:02:37 +01:00
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn and_and_xor() -> TestResult {
|
|
|
|
// Assumes the precedence NOT > AND > XOR > OR
|
|
|
|
run_test(r#"true and true xor true and false"#, "true")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn or_and_xor() -> TestResult {
|
|
|
|
// Assumes the precedence NOT > AND > XOR > OR
|
|
|
|
run_test(r#"true or false xor true or false"#, "true")
|
|
|
|
}
|
2023-01-24 09:05:46 +01:00
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn unbalanced_delimiter() -> TestResult {
|
|
|
|
fail_test(r#"{a:{b:5}}}"#, "unbalanced { and }")
|
|
|
|
}
|
2023-03-10 21:26:14 +01:00
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn unbalanced_delimiter2() -> TestResult {
|
|
|
|
fail_test(r#"{}#.}"#, "unbalanced { and }")
|
|
|
|
}
|
2023-03-17 03:19:23 +01:00
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn unbalanced_delimiter3() -> TestResult {
|
|
|
|
fail_test(r#"{"#, "Unexpected end of code")
|
|
|
|
}
|
2023-04-08 22:04:57 +02:00
|
|
|
|
2023-04-17 11:51:10 +02:00
|
|
|
#[test]
|
|
|
|
fn unbalanced_delimiter4() -> TestResult {
|
|
|
|
fail_test(r#"}"#, "unbalanced { and }")
|
|
|
|
}
|
|
|
|
|
2024-03-07 13:05:04 +01:00
|
|
|
#[test]
|
|
|
|
fn unbalanced_parens1() -> TestResult {
|
|
|
|
fail_test(r#")"#, "unbalanced ( and )")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn unbalanced_parens2() -> TestResult {
|
|
|
|
fail_test(r#"("("))"#, "unbalanced ( and )")
|
|
|
|
}
|
|
|
|
|
2023-04-08 22:04:57 +02:00
|
|
|
#[test]
|
|
|
|
fn register_with_string_literal() -> TestResult {
|
|
|
|
fail_test(r#"register 'nu-plugin-math'"#, "File not found")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn register_with_string_constant() -> TestResult {
|
|
|
|
let input = "\
|
|
|
|
const file = 'nu-plugin-math'
|
|
|
|
register $file
|
|
|
|
";
|
|
|
|
// should not fail with `not a constant`
|
|
|
|
fail_test(input, "File not found")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn register_with_string_variable() -> TestResult {
|
|
|
|
let input = "\
|
|
|
|
let file = 'nu-plugin-math'
|
|
|
|
register $file
|
|
|
|
";
|
|
|
|
fail_test(input, "Value is not a parse-time constant")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn register_with_non_string_constant() -> TestResult {
|
|
|
|
let input = "\
|
|
|
|
const file = 6
|
|
|
|
register $file
|
|
|
|
";
|
|
|
|
fail_test(input, "expected string, found int")
|
|
|
|
}
|
2023-05-12 16:10:40 +02:00
|
|
|
|
2024-04-23 13:37:50 +02:00
|
|
|
#[test]
|
|
|
|
fn plugin_use_with_string_literal() -> TestResult {
|
|
|
|
fail_test(
|
|
|
|
r#"plugin use 'nu-plugin-math'"#,
|
2024-04-25 00:40:39 +02:00
|
|
|
"Plugin registry file not set",
|
2024-04-23 13:37:50 +02:00
|
|
|
)
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn plugin_use_with_string_constant() -> TestResult {
|
|
|
|
let input = "\
|
|
|
|
const file = 'nu-plugin-math'
|
|
|
|
plugin use $file
|
|
|
|
";
|
|
|
|
// should not fail with `not a constant`
|
2024-04-25 00:40:39 +02:00
|
|
|
fail_test(input, "Plugin registry file not set")
|
2024-04-23 13:37:50 +02:00
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn plugin_use_with_string_variable() -> TestResult {
|
|
|
|
let input = "\
|
|
|
|
let file = 'nu-plugin-math'
|
|
|
|
plugin use $file
|
|
|
|
";
|
|
|
|
fail_test(input, "Value is not a parse-time constant")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn plugin_use_with_non_string_constant() -> TestResult {
|
|
|
|
let input = "\
|
|
|
|
const file = 6
|
|
|
|
plugin use $file
|
|
|
|
";
|
|
|
|
fail_test(input, "expected string, found int")
|
|
|
|
}
|
|
|
|
|
2023-05-12 16:10:40 +02:00
|
|
|
#[test]
|
|
|
|
fn extern_errors_with_no_space_between_params_and_name_1() -> TestResult {
|
|
|
|
fail_test("extern cmd[]", "expected space")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn extern_errors_with_no_space_between_params_and_name_2() -> TestResult {
|
|
|
|
fail_test("extern cmd(--flag)", "expected space")
|
|
|
|
}
|
2023-05-18 01:54:35 +02:00
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn duration_with_underscores_1() -> TestResult {
|
|
|
|
run_test("420_min", "7hr")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn duration_with_underscores_2() -> TestResult {
|
|
|
|
run_test("1_000_000sec", "1wk 4day 13hr 46min 40sec")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn duration_with_underscores_3() -> TestResult {
|
Rewrite run_external.rs (#12921)
This PR is a complete rewrite of `run_external.rs`. The main goal of the
rewrite is improving readability, but it also fixes some bugs related to
argument handling and the PATH variable (fixes
https://github.com/nushell/nushell/issues/6011).
I'll discuss some technical details to make reviewing easier.
## Argument handling
Quoting arguments for external commands is hard. Like, *really* hard.
We've had more than a dozen issues and PRs dedicated to quoting
arguments (see Appendix) but the current implementation is still buggy.
Here's a demonstration of the buggy behavior:
```nu
let foo = "'bar'"
^touch $foo # This creates a file named `bar`, but it should be `'bar'`
^touch ...[ "'bar'" ] # Same
```
I'll describe how this PR deals with argument handling.
First, we'll introduce the concept of **bare strings**. Bare strings are
**string literals** that are either **unquoted** or **quoted by
backticks** [^1]. Strings within a list literal are NOT considered bare
strings, even if they are unquoted or quoted by backticks.
When a bare string is used as an argument to external process, we need
to perform tilde-expansion, glob-expansion, and inner-quotes-removal, in
that order. "Inner-quotes-removal" means transforming from
`--option="value"` into `--option=value`.
## `.bat` files and CMD built-ins
On Windows, `.bat` files and `.cmd` files are considered executable, but
they need `CMD.exe` as the interpreter. The Rust standard library
supports running `.bat` files directly and will spawn `CMD.exe` under
the hood (see
[documentation](https://doc.rust-lang.org/std/process/index.html#windows-argument-splitting)).
However, other extensions are not supported [^2].
Nushell also supports a selected number of CMD built-ins. The problem
with CMD is that it uses a different set of quoting rules. Correctly
quoting for CMD requires using
[Command::raw_arg()](https://doc.rust-lang.org/std/os/windows/process/trait.CommandExt.html#tymethod.raw_arg)
and manually quoting CMD special characters, on top of quoting from the
Nushell side. ~~I decided that this is too complex and chose to reject
special characters in CMD built-ins instead [^3]. Hopefully this will
not affact real-world use cases.~~ I've implemented escaping that works
reasonably well.
## `which-support` feature
The `which` crate is now a hard dependency of `nu-command`, making the
`which-support` feature essentially useless. The `which` crate is
already a hard dependency of `nu-cli`, and we should consider removing
the `which-support` feature entirely.
## Appendix
Here's a list of quoting-related issues and PRs in rough chronological
order.
* https://github.com/nushell/nushell/issues/4609
* https://github.com/nushell/nushell/issues/4631
* https://github.com/nushell/nushell/issues/4601
* https://github.com/nushell/nushell/pull/5846
* https://github.com/nushell/nushell/issues/5978
* https://github.com/nushell/nushell/pull/6014
* https://github.com/nushell/nushell/issues/6154
* https://github.com/nushell/nushell/pull/6161
* https://github.com/nushell/nushell/issues/6399
* https://github.com/nushell/nushell/pull/6420
* https://github.com/nushell/nushell/pull/6426
* https://github.com/nushell/nushell/issues/6465
* https://github.com/nushell/nushell/issues/6559
* https://github.com/nushell/nushell/pull/6560
[^1]: The idea that backtick-quoted strings act like bare strings was
introduced by Kubouch and briefly mentioned in [the language
reference](https://www.nushell.sh/lang-guide/chapters/strings_and_text.html#backtick-quotes).
[^2]: The documentation also said "running .bat scripts in this way may
be removed in the future and so should not be relied upon", which is
another reason to move away from this. But again, quoting for CMD is
hard.
[^3]: If anyone wants to try, the best resource I found on the topic is
[this](https://daviddeley.com/autohotkey/parameters/parameters.htm).
2024-05-23 04:05:27 +02:00
|
|
|
fail_test("1_000_d_ay", "Command `1_000_d_ay` not found")
|
2023-05-18 01:54:35 +02:00
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn duration_with_faulty_number() -> TestResult {
|
|
|
|
fail_test("sleep 4-ms", "duration value must be a number")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn filesize_with_underscores_1() -> TestResult {
|
|
|
|
run_test("420_mb", "400.5 MiB")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn filesize_with_underscores_2() -> TestResult {
|
|
|
|
run_test("1_000_000B", "976.6 KiB")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn filesize_with_underscores_3() -> TestResult {
|
Rewrite run_external.rs (#12921)
This PR is a complete rewrite of `run_external.rs`. The main goal of the
rewrite is improving readability, but it also fixes some bugs related to
argument handling and the PATH variable (fixes
https://github.com/nushell/nushell/issues/6011).
I'll discuss some technical details to make reviewing easier.
## Argument handling
Quoting arguments for external commands is hard. Like, *really* hard.
We've had more than a dozen issues and PRs dedicated to quoting
arguments (see Appendix) but the current implementation is still buggy.
Here's a demonstration of the buggy behavior:
```nu
let foo = "'bar'"
^touch $foo # This creates a file named `bar`, but it should be `'bar'`
^touch ...[ "'bar'" ] # Same
```
I'll describe how this PR deals with argument handling.
First, we'll introduce the concept of **bare strings**. Bare strings are
**string literals** that are either **unquoted** or **quoted by
backticks** [^1]. Strings within a list literal are NOT considered bare
strings, even if they are unquoted or quoted by backticks.
When a bare string is used as an argument to external process, we need
to perform tilde-expansion, glob-expansion, and inner-quotes-removal, in
that order. "Inner-quotes-removal" means transforming from
`--option="value"` into `--option=value`.
## `.bat` files and CMD built-ins
On Windows, `.bat` files and `.cmd` files are considered executable, but
they need `CMD.exe` as the interpreter. The Rust standard library
supports running `.bat` files directly and will spawn `CMD.exe` under
the hood (see
[documentation](https://doc.rust-lang.org/std/process/index.html#windows-argument-splitting)).
However, other extensions are not supported [^2].
Nushell also supports a selected number of CMD built-ins. The problem
with CMD is that it uses a different set of quoting rules. Correctly
quoting for CMD requires using
[Command::raw_arg()](https://doc.rust-lang.org/std/os/windows/process/trait.CommandExt.html#tymethod.raw_arg)
and manually quoting CMD special characters, on top of quoting from the
Nushell side. ~~I decided that this is too complex and chose to reject
special characters in CMD built-ins instead [^3]. Hopefully this will
not affact real-world use cases.~~ I've implemented escaping that works
reasonably well.
## `which-support` feature
The `which` crate is now a hard dependency of `nu-command`, making the
`which-support` feature essentially useless. The `which` crate is
already a hard dependency of `nu-cli`, and we should consider removing
the `which-support` feature entirely.
## Appendix
Here's a list of quoting-related issues and PRs in rough chronological
order.
* https://github.com/nushell/nushell/issues/4609
* https://github.com/nushell/nushell/issues/4631
* https://github.com/nushell/nushell/issues/4601
* https://github.com/nushell/nushell/pull/5846
* https://github.com/nushell/nushell/issues/5978
* https://github.com/nushell/nushell/pull/6014
* https://github.com/nushell/nushell/issues/6154
* https://github.com/nushell/nushell/pull/6161
* https://github.com/nushell/nushell/issues/6399
* https://github.com/nushell/nushell/pull/6420
* https://github.com/nushell/nushell/pull/6426
* https://github.com/nushell/nushell/issues/6465
* https://github.com/nushell/nushell/issues/6559
* https://github.com/nushell/nushell/pull/6560
[^1]: The idea that backtick-quoted strings act like bare strings was
introduced by Kubouch and briefly mentioned in [the language
reference](https://www.nushell.sh/lang-guide/chapters/strings_and_text.html#backtick-quotes).
[^2]: The documentation also said "running .bat scripts in this way may
be removed in the future and so should not be relied upon", which is
another reason to move away from this. But again, quoting for CMD is
hard.
[^3]: If anyone wants to try, the best resource I found on the topic is
[this](https://daviddeley.com/autohotkey/parameters/parameters.htm).
2024-05-23 04:05:27 +02:00
|
|
|
fail_test("42m_b", "Command `42m_b` not found")
|
2023-05-18 01:54:35 +02:00
|
|
|
}
|
2023-05-28 12:56:58 +02:00
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn filesize_is_not_hex() -> TestResult {
|
|
|
|
run_test("0x42b", "1067")
|
|
|
|
}
|
Improve type hovers (#9515)
# Description
This PR does a few things to help improve type hovers and, in the
process, fixes a few outstanding issues in the type system. Here's a
list of the changes:
* `for` now will try to infer the type of the iteration variable based
on the expression it's given. This fixes things like `for x in [1, 2, 3]
{ }` where `x` now properly gets the int type.
* Removed old input/output type fields from the signature, focuses on
the vec of signatures. Updated a bunch of dataframe commands that hadn't
moved over. This helps tie things together a bit better
* Fixed inference of types from subexpressions to use the last
expression in the block
* Fixed handling of explicit types in `let` and `mut` calls, so we now
respect that as the authoritative type
I also tried to add `def` input/output type inference, but unfortunately
we only know the predecl types universally, which means we won't have
enough information to properly know what the types of the custom
commands are.
# User-Facing Changes
Script typechecking will get tighter in some cases
Hovers should be more accurate in some cases that previously resorted to
any.
# Tests + Formatting
<!--
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect -A clippy::result_large_err` to check that
you're using the standard code style
- `cargo test --workspace` to check that all tests pass
- `cargo run -- crates/nu-std/tests/run.nu` to run the tests for the
standard library
> **Note**
> from `nushell` you can also use the `toolkit` as follows
> ```bash
> use toolkit.nu # or use an `env_change` hook to activate it
automatically
> toolkit check pr
> ```
-->
# After Submitting
<!-- If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
-->
---------
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2023-06-28 19:19:48 +02:00
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn let_variable_type_mismatch() -> TestResult {
|
|
|
|
fail_test(r#"let x: int = "foo""#, "expected int, found string")
|
|
|
|
}
|
2023-07-14 23:51:28 +02:00
|
|
|
|
2023-10-05 22:39:37 +02:00
|
|
|
#[test]
|
|
|
|
fn let_variable_disallows_completer() -> TestResult {
|
|
|
|
fail_test(
|
|
|
|
r#"let x: int@completer = 42"#,
|
|
|
|
"Unexpected custom completer",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
2023-07-14 23:51:28 +02:00
|
|
|
#[test]
|
2024-04-10 16:28:54 +02:00
|
|
|
fn def_with_input_output() -> TestResult {
|
2023-07-14 23:51:28 +02:00
|
|
|
run_test(r#"def foo []: nothing -> int { 3 }; foo"#, "3")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
2024-04-10 16:28:54 +02:00
|
|
|
fn def_with_input_output_with_line_breaks() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"def foo []: [
|
|
|
|
nothing -> int
|
|
|
|
] { 3 }; foo"#,
|
|
|
|
"3",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn def_with_multi_input_output_with_line_breaks() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"def foo []: [
|
|
|
|
nothing -> int
|
|
|
|
string -> int
|
|
|
|
] { 3 }; foo"#,
|
|
|
|
"3",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn def_with_multi_input_output_without_commas() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"def foo []: [nothing -> int string -> int] { 3 }; foo"#,
|
|
|
|
"3",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn def_with_multi_input_output_called_with_first_sig() -> TestResult {
|
2023-07-14 23:51:28 +02:00
|
|
|
run_test(
|
|
|
|
r#"def foo []: [int -> int, string -> int] { 3 }; 10 | foo"#,
|
|
|
|
"3",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
2024-04-10 16:28:54 +02:00
|
|
|
fn def_with_multi_input_output_called_with_second_sig() -> TestResult {
|
2023-07-14 23:51:28 +02:00
|
|
|
run_test(
|
|
|
|
r#"def foo []: [int -> int, string -> int] { 3 }; "bob" | foo"#,
|
|
|
|
"3",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn def_with_input_output_mismatch_1() -> TestResult {
|
|
|
|
fail_test(
|
|
|
|
r#"def foo []: [int -> int, string -> int] { 3 }; foo"#,
|
|
|
|
"command doesn't support",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn def_with_input_output_mismatch_2() -> TestResult {
|
|
|
|
fail_test(
|
|
|
|
r#"def foo []: [int -> int, string -> int] { 3 }; {x: 2} | foo"#,
|
|
|
|
"command doesn't support",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn def_with_input_output_broken_1() -> TestResult {
|
|
|
|
fail_test(r#"def foo []: int { 3 }"#, "expected arrow")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn def_with_input_output_broken_2() -> TestResult {
|
|
|
|
fail_test(r#"def foo []: int -> { 3 }"#, "expected type")
|
|
|
|
}
|
2023-07-15 09:41:48 +02:00
|
|
|
|
2023-10-05 22:39:37 +02:00
|
|
|
#[test]
|
|
|
|
fn def_with_input_output_broken_3() -> TestResult {
|
|
|
|
fail_test(
|
|
|
|
r#"def foo []: int -> int@completer {}"#,
|
|
|
|
"Unexpected custom completer",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn def_with_input_output_broken_4() -> TestResult {
|
|
|
|
fail_test(
|
|
|
|
r#"def foo []: int -> list<int@completer> {}"#,
|
|
|
|
"Unexpected custom completer",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
2023-07-15 09:41:48 +02:00
|
|
|
#[test]
|
|
|
|
fn def_with_in_var_let_1() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"def foo []: [int -> int, string -> int] { let x = $in; if ($x | describe) == "int" { 3 } else { 4 } }; "100" | foo"#,
|
|
|
|
"4",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn def_with_in_var_let_2() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"def foo []: [int -> int, string -> int] { let x = $in; if ($x | describe) == "int" { 3 } else { 4 } }; 100 | foo"#,
|
|
|
|
"3",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn def_with_in_var_mut_1() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"def foo []: [int -> int, string -> int] { mut x = $in; if ($x | describe) == "int" { 3 } else { 4 } }; "100" | foo"#,
|
|
|
|
"4",
|
|
|
|
)
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn def_with_in_var_mut_2() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"def foo []: [int -> int, string -> int] { mut x = $in; if ($x | describe) == "int" { 3 } else { 4 } }; 100 | foo"#,
|
|
|
|
"3",
|
|
|
|
)
|
|
|
|
}
|
2023-07-20 21:10:54 +02:00
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn properly_nest_captures() -> TestResult {
|
|
|
|
run_test(r#"do { let b = 3; def c [] { $b }; c }"#, "3")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn properly_nest_captures_call_first() -> TestResult {
|
|
|
|
run_test(r#"do { let b = 3; c; def c [] { $b }; c }"#, "3")
|
|
|
|
}
|
2023-07-26 20:22:08 +02:00
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn properly_typecheck_rest_param() -> TestResult {
|
|
|
|
run_test(
|
|
|
|
r#"def foo [...rest: string] { $rest | length }; foo "a" "b" "c""#,
|
|
|
|
"3",
|
|
|
|
)
|
|
|
|
}
|
2023-07-27 20:26:28 +02:00
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn implied_collect_has_compatible_type() -> TestResult {
|
|
|
|
run_test(r#"let idx = 3 | $in; $idx < 1"#, "false")
|
|
|
|
}
|
More specific errors for missing values in records (#11423)
# Description
Currently, when writing a record, if you don't give the value for a
field, the syntax error highlights the entire record instead of
pinpointing the issue. Here's some examples:
```nushell
> { a: 2, 3 } # Missing colon (and value)
Error: nu::parser::parse_mismatch
× Parse mismatch during operation.
╭─[entry #2:1:1]
1 │ { a: 2, 3 }
· ─────┬─────
· ╰── expected record
╰────
> { a: 2, 3: } # Missing value
Error: nu::parser::parse_mismatch
× Parse mismatch during operation.
╭─[entry #3:1:1]
1 │ { a: 2, 3: }
· ──────┬─────
· ╰── expected record
╰────
> { a: 2, 3 4 } # Missing colon
Error: nu::parser::parse_mismatch
× Parse mismatch during operation.
╭─[entry #4:1:1]
1 │ { a: 2, 3 4 }
· ──────┬──────
· ╰── expected record
╰────
```
In all of them, the entire record is highlighted red because an
`Expr::Garbage` is returned covering that whole span:

This PR is for highlighting only the part inside the record that could
not be parsed. If the record literal is big, an error message pointing
to the start of where the parser thinks things went wrong should help
people fix their code.
# User-Facing Changes
Below are screenshots of the new errors:
If there's a stray record key right before the record ends, it
highlights only that key and tells the user it expected a colon after
it:

If the record ends before the value for the last field was given, it
highlights the key and colon of that field and tells the user it
expected a value after the colon:

If there are two consecutive expressions without a colon between them,
it highlights everything from the second expression to the end of the
record and tells the user it expected a colon. I was tempted to add a
help message suggesting adding a colon in between, but that may not
always be the right thing to do.

# Tests + Formatting
# After Submitting
2023-12-27 10:15:12 +01:00
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn record_expected_colon() -> TestResult {
|
|
|
|
fail_test(r#"{ a: 2 b }"#, "expected ':'")?;
|
|
|
|
fail_test(r#"{ a: 2 b 3 }"#, "expected ':'")
|
|
|
|
}
|
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn record_missing_value() -> TestResult {
|
|
|
|
fail_test(r#"{ a: 2 b: }"#, "expected value for record field")
|
|
|
|
}
|
2024-02-03 12:20:40 +01:00
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn def_requires_body_closure() -> TestResult {
|
|
|
|
fail_test("def a [] (echo 4)", "expected definition body closure")
|
|
|
|
}
|
2024-04-23 00:10:35 +02:00
|
|
|
|
|
|
|
#[test]
|
|
|
|
fn not_panic_with_recursive_call() {
|
|
|
|
let result = nu!(nu_repl_code(&[
|
|
|
|
"def px [] { if true { 3 } else { px } }",
|
|
|
|
"let x = 1",
|
|
|
|
"$x | px",
|
|
|
|
]));
|
|
|
|
assert_eq!(result.out, "3");
|
|
|
|
|
|
|
|
let result = nu!(nu_repl_code(&[
|
|
|
|
"def px [n=0] { let l = $in; if $n == 0 { return false } else { $l | px ($n - 1) } }",
|
|
|
|
"let x = 1",
|
|
|
|
"$x | px"
|
|
|
|
]));
|
|
|
|
assert_eq!(result.out, "false");
|
|
|
|
|
|
|
|
let result = nu!(nu_repl_code(&[
|
|
|
|
"def px [n=0] { let l = $in; if $n == 0 { return false } else { $l | px ($n - 1) } }",
|
|
|
|
"let x = 1",
|
|
|
|
"def foo [] { $x }",
|
|
|
|
"foo | px"
|
|
|
|
]));
|
|
|
|
assert_eq!(result.out, "false");
|
|
|
|
|
|
|
|
let result = nu!(nu_repl_code(&[
|
|
|
|
"def px [n=0] { let l = $in; if $n == 0 { return false } else { $l | px ($n - 1) } }",
|
|
|
|
"let x = 1",
|
|
|
|
"do {|| $x } | px"
|
|
|
|
]));
|
|
|
|
assert_eq!(result.out, "false");
|
|
|
|
|
|
|
|
let result = nu!(
|
|
|
|
cwd: "tests/parsing/samples",
|
|
|
|
"nu recursive_func_with_alias.nu"
|
|
|
|
);
|
|
|
|
assert!(result.status.success());
|
|
|
|
}
|