# Description
Closes#7762. See issue for motivation.
# User-Facing Changes
Something like this is now possible without having to split this into 2
commands:
```
fetch "https://www.gutenberg.org/files/11/11-0.txt" | benchmark { str downcase | split words | uniq -c | sort-by count --reverse | first 10 }
```
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
Co-authored-by: Stefan Holderbach <sholderbach@users.noreply.github.com>
# Description
`src/locale_override.rs` is gated with `#![cfg(debug_assertions)]` but
`tests/get_system_locale.rs` is not; this makes `cargo test --release`
fails:
```
error[E0432]: unresolved import `nu_test_support::locale_override`
--> crates/nu-test-support/tests/get_system_locale.rs:1:22
|
1 | use nu_test_support::locale_override::with_locale_override;
| ^^^^^^^^^^^^^^^ could not find `locale_override` in `nu_test_support`
For more information about this error, try `rustc --explain E0432`.
error: could not compile `nu-test-support` due to previous error
warning: build failed, waiting for other jobs to finish...
```
With the change it now passes:
```
❯ cargo test --release
Compiling nu-test-support v0.74.1 (/home/michel/src/github/nushell/nushell/crates/nu-test-support)
Finished release [optimized] target(s) in 7.57s
Running unittests src/lib.rs (/home/michel/src/github/nushell/nushell/target/release/deps/nu_test_support-750505b13c102d2c)
running 3 tests
test tests::constructs_a_pipeline ... ok
test playground::tests::current_working_directory_back_to_root_from_anywhere ... ok
test playground::tests::current_working_directory_in_sandbox_directory_created ... ok
test result: ok. 3 passed; 0 failed; 0 ignored; 0 measured; 0 filtered out; finished in 0.00s
Running tests/get_system_locale.rs (/home/michel/src/github/nushell/nushell/target/release/deps/get_system_locale-e0ecabe312044fa8)
running 0 tests
test result: ok. 0 passed; 0 failed; 0 ignored; 0 measured; 0 filtered out; finished in 0.00s
```
Signed-off-by: Michel Alexandre Salim <salimma@fedoraproject.org>
# User-Facing Changes
N/A
Signed-off-by: Michel Alexandre Salim <salimma@fedoraproject.org>
# Updated description by @rgwood
This PR changes `fetch` to `http get` and `post` to `http post`. `fetch`
and `post` are now deprecated. [I surveyed people on
Discord](https://discord.com/channels/601130461678272522/601130461678272524/1065706282566307910)
and users strongly approved of this change.
# Original Description
This PR is related to #2741 and my first pull request in rust :)
Implemented a new http mod to better http support and alias `fetch` and
`post` commands to `http get` and `http post` respectively.
# User-Facing Changes
Users will be able to use HTTP method via http command, for example
``` shell
> http get "https://www.example.com"
<!doctype html>
<html>
...
```
# Description
This PR fixes the signature display when running `help commands`. Before
this PR, there were leading spaces in the signatures column. Now,
they're left aligned for a cleaner look.
Before:
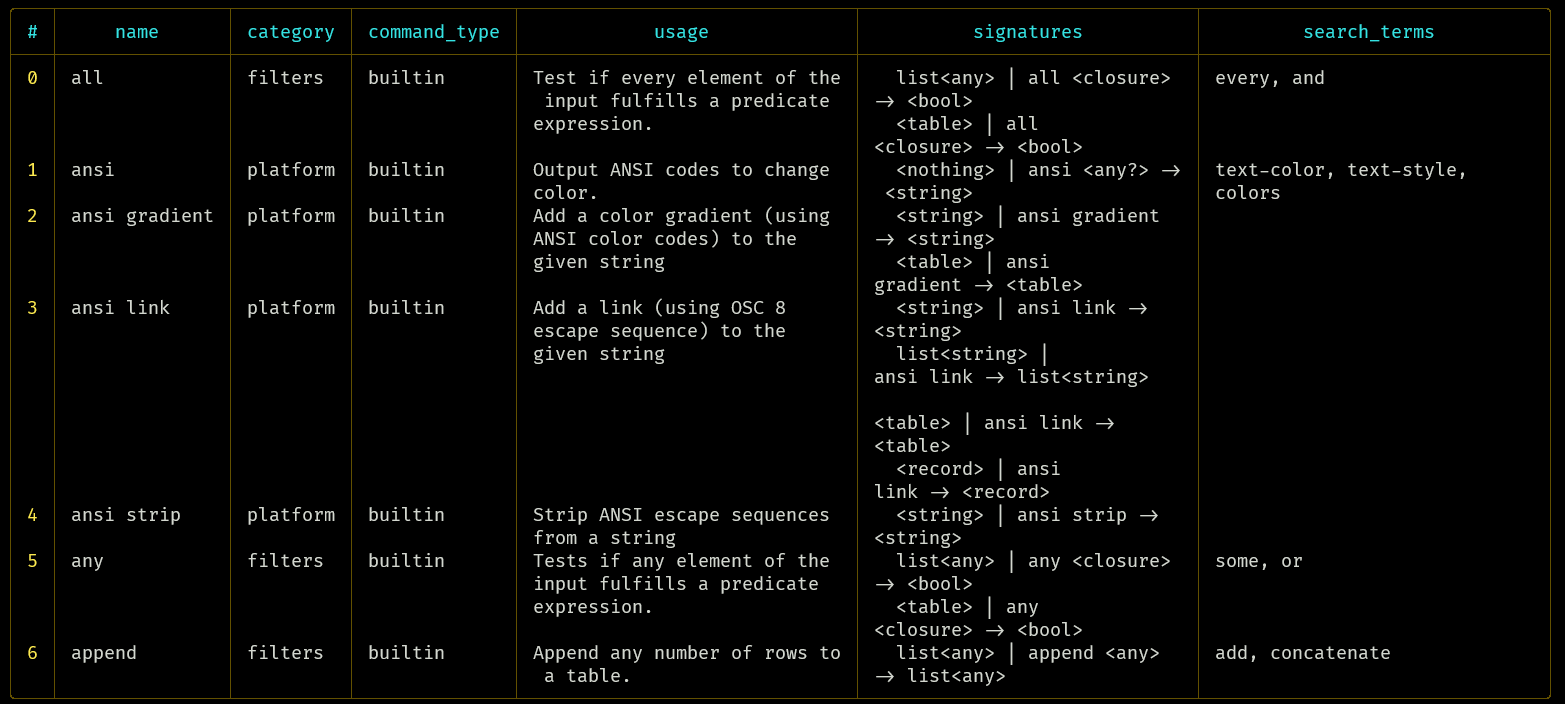
After:
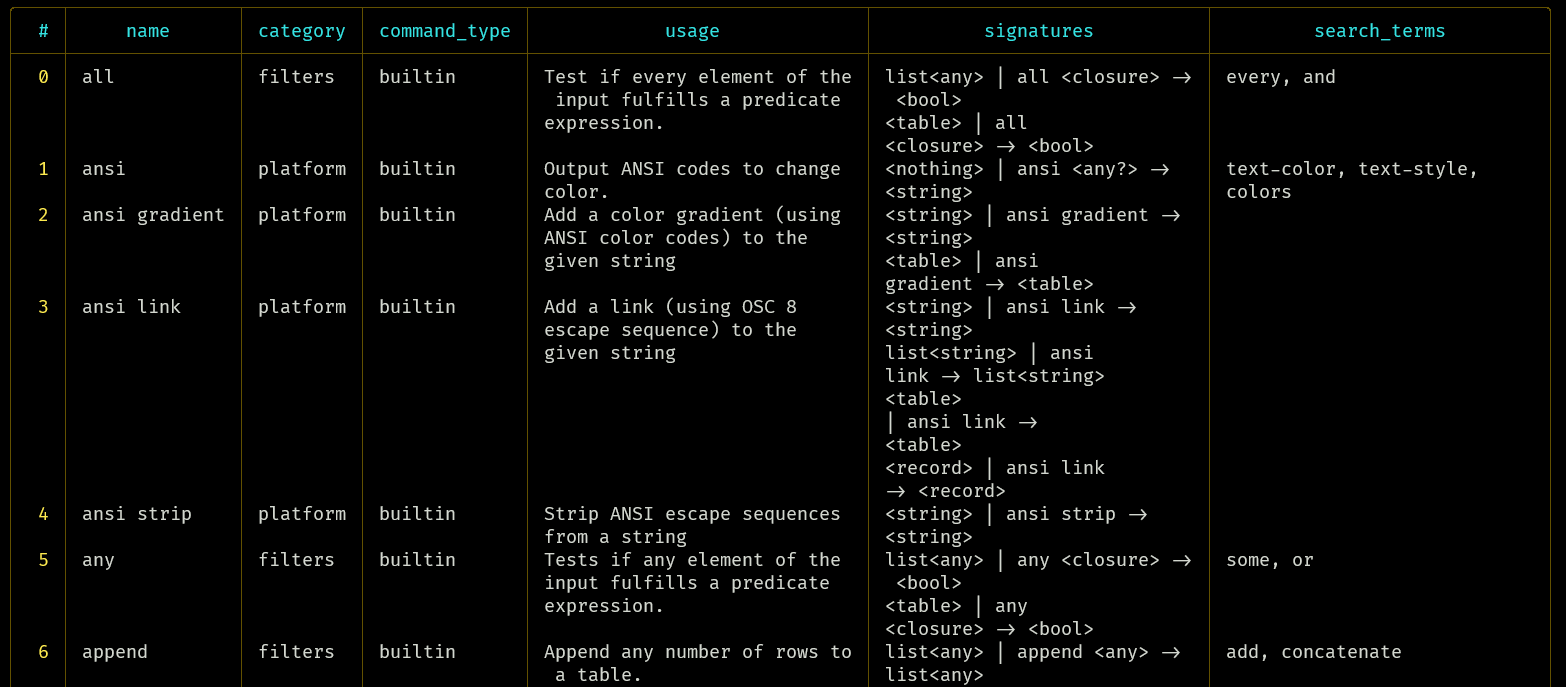
# User-Facing Changes
_(List of all changes that impact the user experience here. This helps
us keep track of breaking changes.)_
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
BEFORE:
```
Subcommands:
str camel-case - Convert a string to camelCase
str capitalize - Capitalize first letter of text
str collect - 'str collect' is deprecated. Please use 'str join' instead.
str contains - Checks if string input contains a substring
str distance - Compare two strings and return the edit distance/Levenshtein distance
str downcase - Make text lowercase
str ends-with - Check if an input ends with a string
str find-replace - Deprecated command
str index-of - Returns start index of first occurrence of string in input, or -1 if no match
str join - Concatenate multiple strings into a single string, with an optional separator between each
str kebab-case - Convert a string to kebab-case
str length - Output the length of any strings in the pipeline
str lpad - Left-pad a string to a specific length
str pascal-case - Convert a string to PascalCase
str replace - Find and replace text
str reverse - Reverse every string in the pipeline
str rpad - Right-pad a string to a specific length
str screaming-snake-case - Convert a string to SCREAMING_SNAKE_CASE
str snake-case - Convert a string to snake_case
str starts-with - Check if an input starts with a string
str substring - Get part of a string. Note that the start is included but the end is excluded, and that the first character of a string is index 0.
str title-case - Convert a string to Title Case
str to-datetime - Deprecated command
str to-decimal - Deprecated command
str to-int - Deprecated command
str trim - Trim whitespace or specific character
str upcase - Make text uppercase
```
AFTER:
```
Subcommands:
str camel-case - Convert a string to camelCase
str capitalize - Capitalize first letter of text
str contains - Checks if string input contains a substring
str distance - Compare two strings and return the edit distance/Levenshtein distance
str downcase - Make text lowercase
str ends-with - Check if an input ends with a string
str index-of - Returns start index of first occurrence of string in input, or -1 if no match
str join - Concatenate multiple strings into a single string, with an optional separator between each
str kebab-case - Convert a string to kebab-case
str length - Output the length of any strings in the pipeline
str lpad - Left-pad a string to a specific length
str pascal-case - Convert a string to PascalCase
str replace - Find and replace text
str reverse - Reverse every string in the pipeline
str rpad - Right-pad a string to a specific length
str screaming-snake-case - Convert a string to SCREAMING_SNAKE_CASE
str snake-case - Convert a string to snake_case
str starts-with - Check if an input starts with a string
str substring - Get part of a string. Note that the start is included but the end is excluded, and that the first character of a string is index 0.
str title-case - Convert a string to Title Case
str trim - Trim whitespace or specific character
str upcase - Make text uppercase
```
The deprecated subcommands still exist, but are no longer listed in
`help` for the containing command.
# User-Facing Changes
See above.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
Fixes#7774. The functionality should be the same as feeding all
`PipelineDate::Value(Value::String(_,_),_)` into `lines` before putting
it into `find`.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
This is an attempt to implement a new `Value::LazyRecord` variant for
performance reasons.
`LazyRecord` is like a regular `Record`, but it's possible to access
individual columns without evaluating other columns. I've implemented
`LazyRecord` for the special `$nu` variable; accessing `$nu` is
relatively slow because of all the information in `scope`, and [`$nu`
accounts for about 2/3 of Nu's startup time on
Linux](https://github.com/nushell/nushell/issues/6677#issuecomment-1364618122).
### Benchmarks
I ran some benchmarks on my desktop (Linux, 12900K) and the results are
very pleasing.
Nu's time to start up and run a command (`cargo build --release;
hyperfine 'target/release/nu -c "echo \"Hello, world!\""' --shell=none
--warmup 10`) goes from **8.8ms to 3.2ms, about 2.8x faster**.
Tests are also much faster! Running `cargo nextest` (with our very slow
`proptest` tests disabled) goes from **7.2s to 4.4s (1.6x faster)**,
because most tests involve launching a new instance of Nu.
### Design (updated)
I've added a new `LazyRecord` trait and added a `Value` variant wrapping
those trait objects, much like `CustomValue`. `LazyRecord`
implementations must implement these 2 functions:
```rust
// All column names
fn column_names(&self) -> Vec<&'static str>;
// Get 1 specific column value
fn get_column_value(&self, column: &str) -> Result<Value, ShellError>;
```
### Serializability
`Value` variants must implement `Serializable` and `Deserializable`, which poses some problems because I want to use unserializable things like `EngineState` in `LazyRecord`s. To work around this, I basically lie to the type system:
1. Add `#[typetag::serde(tag = "type")]` to `LazyRecord` to make it serializable
2. Any unserializable fields in `LazyRecord` implementations get marked with `#[serde(skip)]`
3. At the point where a `LazyRecord` normally would get serialized and sent to a plugin, I instead collect it into a regular `Value::Record` (which can be serialized)
Previously the group check was only for the current users gid, now we
check against all the users groups.
# Description
Currently when using the `cd` command to enter a directory there was
only a check against the current user id, and exact current group id,
meaning if a directory had a group permission and that group wasn't the
users group it was effectively ignored.
The fix simply implements a check through all the users current groups.
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
# Description
Nothing changed, just fix some typos
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
Co-authored-by: Stefan Holderbach <sholderbach@users.noreply.github.com>
# Description
This PR updates the `sqlparser` dependency and updates code to the
latest api changes.
# User-Facing Changes
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
This PR updates the semver dependency and updates the `inc` plugin to
use the latest api.
# User-Facing Changes
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
Closes: #7590
# User-Facing Changes
So the following command
```
1..100 | each { |i| sleep 400ms; $i} | save --raw -f output.txt
```
Will stream data to `output.txt`
But I'm note sure how to make a proper test for it, so I leave with no
new test cases..
Also rename from `string_binary_list_value_to_bytes ` to
`value_to_bytes` to accepts more Value type.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
Refactor command: "to url" in: "to url query". Changed usage sentence.
Closes: #7495
# User-Facing Changes
Now we get a query string from a record or table by using command: "to
url query".
```
> help to url query
Convert record or table into query string applying percent-encoding.
Usage:
> to url query
Flags:
-h, --help - Display the help message for this command
Signatures:
<record> | to url query -> <string>
<table> | to url query -> <string>
Examples:
Outputs a query string representing the contents of this record
> { mode:normal userid:31415 } | to url query
Outputs a query string representing the contents of this 1-row table
> [[foo bar]; ["1" "2"]] | to url query
Outputs a query string representing the contents of this record
> {a:"AT&T", b: "AT T"} | to url query
```
# Tests + Formatting
Added this test:
```
Example {
description: "Outputs a query string representing the contents of this record",
example: r#"{a:"AT&T", b: "AT T"} | to url query"#,
result: Some(Value::test_string("a=AT%26T&b=AT+T")),
},
```
to ensure percent-encoding.
# After Submitting
If PR is accepted I'll open another PR on documentation to notify
changes on
[this.](https://github.com/nushell/nushell.github.io/blob/main/book/commands/to_url.md)
# Description
This PR makes changes that allow underscores in numbers.
Example:
```nu
# allows underscores to be placed arbitrarily to enhance readability.
let pi = 3.1415_9265_3589_793
# works with integers
let num = 1_000_000_000_000
let fav_color = 0x68_9d_6a
```
I have changed `assert!(a == b)` calls to `assert_eq!(a, b)`, which give
better error messages. Similarly for `assert!(a != b)` and
`assert_ne!(a, b)`. Basically all instances were comparing primitives
(string slices or integers), so there is no loss of generality from
special-case macros,
I have also fixed a number of typos in comments, variable names, and a
few user-facing messages.
# Description
This PR allows the configuration of cursor shapes in nushell for each
edit mode. This is the change that is in the default_config.nu file.
```
cursor_shape: {
emacs: line # block, underscore, line (line is the default)
vi_insert: block # block, underscore, line (block is the default)
vi_normal: underscore # block, underscore, line (underscore is the default)
}
```
# User-Facing Changes
See above. If you'd prefer a different default, please speak up and let
us know.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
Closes#6337 and #5366. Prior to this PR, when "shelling out" to cmd.exe
on Windows we were not trimming quotes correctly:
```bash
〉^echo "foo"
\"foo\"
```
After this change, we do:
```bash
〉^echo "foo"
foo
```
### Breaking Change
I ended up removing `dir` from the list of supported cmd.exe internal
commands as part of this PR.
For this PR, I extracted the argument-cleaning-and-expanding code from
`spawn_simple_command()` for reuse in `spawn_cmd_command()`. This means
that we now expand globs, which broke some tests for the `dir` cmd.exe
internal command.
I probably could have kept the tests working, but... tbh, I don't think
it's worth it. I don't want to make the `cmd.exe` functionality any more
complicated than it already is, and calling `dir` from Nu is always
going to be weird+hacky compared to `ls`.
# Description
As title
# User-Facing Changes
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
This PR updates the base64 crate, which has changed significantly, so
all the base64 implementations had to be changed too. Tests pass. I hope
that's enough.
# User-Facing Changes
None, except added a new character encoding imap-mutf7 as mutf7.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
In bash when a program crashes, it prints the reason for what happened:
```
$ ./division_by_zero
Floating point exception (core dumped)
$ ./segfault
Segmentation fault (core dumped)
```
Nushell always prints the same thing in this case:
```
> ./division_by_zero
nushell: oops, process './division_by_zero' core dumped
Error: nu:🐚:external_command (link)
# etc..
```
This PR adds more detailed error printing, like in bash:
```
> ./division_by_zero
Floating point exception: oops, process './division_by_zero' core dumped
Error: nu:🐚:external_command (link)
# etc..
```
I made this message format as an example:
```
Floating point exception: oops, process './division_by_zero' core dumped
```
Instead of `nushell:` it writes a meaningful message, but I can change
this format as per the suggestions.
I tested the change only on linux, but it should work on other unix
systems.
# User-Facing Changes
The error message only.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
Co-authored-by: Stefan Holderbach <sholderbach@users.noreply.github.com>
# Description
Closes#7514.
* For both `encode` and `decode`: add a special case allowing `utf16` as
a valid alias for `utf-16` (just as `utf-8` has `utf8`).
* For `encode` , make it an error when encodings_rs replaces characters
outside the given encoding with HTML entities
* For `encode` , add `-i`/`--ignore-errors` flag to bring back this
behaviour.
Note: `--ignore-errors` does NOT ignore the error for using a wrong
encoding label like `uft8`
# User-Facing Changes
See above.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
# Description
Refers to: [5093](https://github.com/nushell/nushell/issues/5093)
# Tests
- [x] `cargo fmt --all -- --check` to check standard code formatting
(`cargo fmt --all` applies these changes)
- [x] `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- [x] `cargo test --workspace` to check that all tests pass
# Description
Closes: #7696
# User-Facing Changes
Before:
```
❯ 'temp/aa' | path exists
Error: nu:🐚:io_error (link)
× I/O error
help: Os { code: 13, kind: PermissionDenied, message: "Permission denied" }
```
After:
```
❯ 'temp/aa' | path exists
Error: nu:🐚:io_error (link)
× I/O error
╭─[entry #42:1:1]
1 │ 'temp/aa' | path exists
· ────┬────
· ╰── Permission denied (os error 13)
╰────
```
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
# Description
As title
Fixes: #7673Fixes: #4205
Also possiblely fixes: https://github.com/nushell/nushell/issues/6993
# User-Facing Changes
Before:
```
> ^echo "~"
/Users/ttt
```
After:
```
> ^echo "~"
~
```
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.