2021-11-26 09:00:57 +01:00
|
|
|
use nu_protocol::engine::{EngineState, StateWorkingSet};
|
2021-09-03 00:58:15 +02:00
|
|
|
|
2023-06-14 23:12:55 +02:00
|
|
|
use crate::{
|
|
|
|
help::{HelpAliases, HelpCommands, HelpExterns, HelpModules, HelpOperators},
|
|
|
|
*,
|
|
|
|
};
|
|
|
|
pub fn add_shell_command_context(mut engine_state: EngineState) -> EngineState {
|
2021-09-03 00:58:15 +02:00
|
|
|
let delta = {
|
2021-10-25 08:31:39 +02:00
|
|
|
let mut working_set = StateWorkingSet::new(&engine_state);
|
2021-09-03 00:58:15 +02:00
|
|
|
|
2021-10-10 06:13:15 +02:00
|
|
|
macro_rules! bind_command {
|
2021-12-11 00:14:28 +01:00
|
|
|
( $( $command:expr ),* $(,)? ) => {
|
2021-10-10 06:13:15 +02:00
|
|
|
$( working_set.add_decl(Box::new($command)); )*
|
|
|
|
};
|
|
|
|
}
|
2021-09-23 21:03:08 +02:00
|
|
|
|
2021-11-28 20:35:02 +01:00
|
|
|
// If there are commands that have the same name as default declarations,
|
|
|
|
// they have to be registered before the main declarations. This helps to make
|
|
|
|
// them only accessible if the correct input value category is used with the
|
|
|
|
// declaration
|
2023-06-01 19:46:16 +02:00
|
|
|
|
2022-04-24 11:29:21 +02:00
|
|
|
// Database-related
|
|
|
|
// Adds all related commands to query databases
|
2022-11-23 01:58:11 +01:00
|
|
|
#[cfg(feature = "sqlite")]
|
2022-04-24 11:29:21 +02:00
|
|
|
add_database_decls(&mut working_set);
|
|
|
|
|
2022-05-13 13:48:47 +02:00
|
|
|
// Charts
|
|
|
|
bind_command! {
|
|
|
|
Histogram
|
|
|
|
}
|
|
|
|
|
2021-12-11 04:07:39 +01:00
|
|
|
// Filters
|
|
|
|
bind_command! {
|
|
|
|
All,
|
|
|
|
Any,
|
|
|
|
Append,
|
2021-12-18 19:14:28 +01:00
|
|
|
Columns,
|
2021-12-23 04:08:39 +01:00
|
|
|
Compact,
|
2022-01-25 16:02:15 +01:00
|
|
|
Default,
|
2021-12-11 04:07:39 +01:00
|
|
|
Drop,
|
2021-12-14 20:54:27 +01:00
|
|
|
DropColumn,
|
2021-12-15 13:26:15 +01:00
|
|
|
DropNth,
|
2021-12-11 04:07:39 +01:00
|
|
|
Each,
|
2021-12-19 20:11:57 +01:00
|
|
|
Empty,
|
2023-01-27 18:45:57 +01:00
|
|
|
Enumerate,
|
2021-12-31 00:41:18 +01:00
|
|
|
Every,
|
2022-12-10 18:23:24 +01:00
|
|
|
Filter,
|
2022-01-23 23:32:02 +01:00
|
|
|
Find,
|
2021-12-11 04:07:39 +01:00
|
|
|
First,
|
2021-12-17 21:44:51 +01:00
|
|
|
Flatten,
|
2021-12-11 04:07:39 +01:00
|
|
|
Get,
|
2022-03-07 02:01:29 +01:00
|
|
|
Group,
|
2022-01-21 21:28:21 +01:00
|
|
|
GroupBy,
|
2022-02-12 03:06:49 +01:00
|
|
|
Headers,
|
2022-03-17 18:55:02 +01:00
|
|
|
Insert,
|
2023-04-14 21:42:33 +02:00
|
|
|
Items,
|
2023-03-17 00:57:20 +01:00
|
|
|
Join,
|
2022-01-31 00:29:21 +01:00
|
|
|
SplitBy,
|
2022-04-07 22:49:28 +02:00
|
|
|
Take,
|
2022-01-22 00:50:26 +01:00
|
|
|
Merge,
|
2022-01-24 20:43:38 +01:00
|
|
|
Move,
|
2022-04-07 22:49:28 +02:00
|
|
|
TakeWhile,
|
|
|
|
TakeUntil,
|
2021-10-26 20:29:00 +02:00
|
|
|
Last,
|
2021-10-10 22:24:54 +02:00
|
|
|
Length,
|
|
|
|
Lines,
|
2021-10-26 03:30:53 +02:00
|
|
|
ParEach,
|
2021-12-07 09:46:21 +01:00
|
|
|
Prepend,
|
2021-10-30 19:51:20 +02:00
|
|
|
Range,
|
2022-01-08 01:40:40 +01:00
|
|
|
Reduce,
|
2021-12-05 04:09:45 +01:00
|
|
|
Reject,
|
2022-01-29 11:26:47 +01:00
|
|
|
Rename,
|
2021-11-07 19:18:27 +01:00
|
|
|
Reverse,
|
2021-10-10 22:24:54 +02:00
|
|
|
Select,
|
2021-11-07 02:19:57 +01:00
|
|
|
Shuffle,
|
2021-11-29 07:52:23 +01:00
|
|
|
Skip,
|
|
|
|
SkipUntil,
|
|
|
|
SkipWhile,
|
2022-04-01 00:43:16 +02:00
|
|
|
Sort,
|
2022-01-22 21:49:50 +01:00
|
|
|
SortBy,
|
2022-07-16 13:24:37 +02:00
|
|
|
SplitList,
|
2022-01-21 21:28:21 +01:00
|
|
|
Transpose,
|
2021-12-11 04:07:39 +01:00
|
|
|
Uniq,
|
2022-12-02 11:36:01 +01:00
|
|
|
UniqBy,
|
2022-03-17 01:13:34 +01:00
|
|
|
Upsert,
|
2022-03-17 18:55:02 +01:00
|
|
|
Update,
|
2022-12-23 19:49:19 +01:00
|
|
|
Values,
|
2021-12-11 04:07:39 +01:00
|
|
|
Where,
|
2022-03-07 02:01:29 +01:00
|
|
|
Window,
|
2021-12-11 04:07:39 +01:00
|
|
|
Wrap,
|
|
|
|
Zip,
|
|
|
|
};
|
|
|
|
|
2022-06-16 18:58:38 +02:00
|
|
|
// Misc
|
|
|
|
bind_command! {
|
2023-05-04 00:02:03 +02:00
|
|
|
Source,
|
2022-06-16 18:58:38 +02:00
|
|
|
Tutor,
|
|
|
|
};
|
|
|
|
|
2021-12-13 02:47:14 +01:00
|
|
|
// Path
|
|
|
|
bind_command! {
|
|
|
|
Path,
|
|
|
|
PathBasename,
|
|
|
|
PathDirname,
|
|
|
|
PathExists,
|
|
|
|
PathExpand,
|
|
|
|
PathJoin,
|
|
|
|
PathParse,
|
|
|
|
PathRelativeTo,
|
|
|
|
PathSplit,
|
|
|
|
PathType,
|
|
|
|
};
|
|
|
|
|
2021-12-11 04:07:39 +01:00
|
|
|
// System
|
|
|
|
bind_command! {
|
2022-02-25 20:51:31 +01:00
|
|
|
Complete,
|
2021-12-11 04:07:39 +01:00
|
|
|
External,
|
2022-06-26 13:53:06 +02:00
|
|
|
NuCheck,
|
2021-12-11 04:07:39 +01:00
|
|
|
Sys,
|
2023-02-13 17:39:07 +01:00
|
|
|
};
|
|
|
|
|
2023-06-14 23:12:55 +02:00
|
|
|
// Help
|
|
|
|
bind_command! {
|
|
|
|
Help,
|
|
|
|
HelpAliases,
|
|
|
|
HelpExterns,
|
|
|
|
HelpCommands,
|
|
|
|
HelpModules,
|
|
|
|
HelpOperators,
|
|
|
|
};
|
|
|
|
|
2023-02-13 17:39:07 +01:00
|
|
|
// Debug
|
|
|
|
bind_command! {
|
2023-02-14 19:47:34 +01:00
|
|
|
Ast,
|
2023-02-13 17:39:07 +01:00
|
|
|
Debug,
|
|
|
|
Explain,
|
|
|
|
Inspect,
|
|
|
|
Metadata,
|
|
|
|
Profile,
|
2023-02-11 19:57:48 +01:00
|
|
|
TimeIt,
|
2023-02-13 17:39:07 +01:00
|
|
|
View,
|
|
|
|
ViewFiles,
|
|
|
|
ViewSource,
|
|
|
|
ViewSpan,
|
2021-12-11 04:07:39 +01:00
|
|
|
};
|
|
|
|
|
2022-10-07 20:54:36 +02:00
|
|
|
#[cfg(unix)]
|
|
|
|
bind_command! { Exec }
|
|
|
|
|
|
|
|
#[cfg(windows)]
|
|
|
|
bind_command! { RegistryQuery }
|
|
|
|
|
2022-09-01 02:34:26 +02:00
|
|
|
#[cfg(any(
|
|
|
|
target_os = "android",
|
|
|
|
target_os = "linux",
|
|
|
|
target_os = "macos",
|
|
|
|
target_os = "windows"
|
|
|
|
))]
|
|
|
|
bind_command! { Ps };
|
|
|
|
|
2022-03-29 13:10:43 +02:00
|
|
|
#[cfg(feature = "which-support")]
|
2022-01-20 19:02:53 +01:00
|
|
|
bind_command! { Which };
|
|
|
|
|
2021-12-11 04:07:39 +01:00
|
|
|
// Strings
|
|
|
|
bind_command! {
|
2021-12-16 00:08:12 +01:00
|
|
|
Char,
|
2021-12-24 08:22:11 +01:00
|
|
|
Decode,
|
2022-06-25 23:35:23 +02:00
|
|
|
Encode,
|
|
|
|
DecodeBase64,
|
|
|
|
EncodeBase64,
|
2022-01-30 13:52:24 +01:00
|
|
|
DetectColumns,
|
2021-12-11 04:07:39 +01:00
|
|
|
Parse,
|
|
|
|
Size,
|
2021-10-10 22:24:54 +02:00
|
|
|
Split,
|
|
|
|
SplitChars,
|
|
|
|
SplitColumn,
|
|
|
|
SplitRow,
|
2022-08-19 19:55:54 +02:00
|
|
|
SplitWords,
|
2021-11-09 02:59:44 +01:00
|
|
|
Str,
|
2021-11-09 08:40:56 +01:00
|
|
|
StrCapitalize,
|
2021-11-09 21:16:53 +01:00
|
|
|
StrContains,
|
2022-08-23 15:53:14 +02:00
|
|
|
StrDistance,
|
2021-11-09 21:16:53 +01:00
|
|
|
StrDowncase,
|
2021-11-10 01:51:55 +01:00
|
|
|
StrEndswith,
|
2023-06-28 19:57:44 +02:00
|
|
|
StrExpand,
|
2022-09-11 10:48:27 +02:00
|
|
|
StrJoin,
|
2022-04-07 15:41:09 +02:00
|
|
|
StrReplace,
|
2021-12-11 04:07:39 +01:00
|
|
|
StrIndexOf,
|
|
|
|
StrLength,
|
|
|
|
StrReverse,
|
2021-11-16 00:16:56 +01:00
|
|
|
StrStartsWith,
|
2021-12-01 07:42:57 +01:00
|
|
|
StrSubstring,
|
2021-12-02 05:38:44 +01:00
|
|
|
StrTrim,
|
2023-08-03 20:06:00 +02:00
|
|
|
StrUpcase,
|
2023-08-10 20:01:47 +02:00
|
|
|
FormatDate,
|
|
|
|
FormatDuration,
|
|
|
|
FormatFilesize,
|
2021-12-11 04:07:39 +01:00
|
|
|
};
|
|
|
|
|
|
|
|
// FileSystem
|
|
|
|
bind_command! {
|
|
|
|
Cd,
|
|
|
|
Ls,
|
|
|
|
Mkdir,
|
|
|
|
Mv,
|
use uutils/coreutils cp command in place of nushell's cp command (#10097)
<!--
if this PR closes one or more issues, you can automatically link the PR
with
them by using one of the [*linking
keywords*](https://docs.github.com/en/issues/tracking-your-work-with-issues/linking-a-pull-request-to-an-issue#linking-a-pull-request-to-an-issue-using-a-keyword),
e.g.
- this PR should close #xxxx
- fixes #xxxx
you can also mention related issues, PRs or discussions!
-->
# Description
Hi. Basically, this is a continuation of the work that @fdncred started.
Given some nice discussions on #9463 , and [merged uutils
PR](https://github.com/uutils/coreutils/pull/5152) from @tertsdiepraam
we have decided to give the `cp` command the `crawl` stage as it was
named.
> [!NOTE]
Given that the `uutils` crate has not made the release for the merged
PR, just make sure you checkout latest and put it in the required place
to make this PR work.
The aim of this PR is for is to see how to move forward using `uutils`
crate. In order to getting this started, I have made the current
`nushell cp tests` pass along with some extra ones I copied over from
the `uutils` repo.
With all of that being said, things that would be nice to decide, and
keep working on:
Crawl:
- Handling of certain `named` flags, with their long and short
forms(e.g. --update, --reflink, --preserve, etc), and using default
values. Maybe `-u` can already have a `default_missing_value`.
- Should we maybe just support one single option `switch` flags (see
`--backup` in code) as a contrast to the other named args.
- Complete test coverage from `uutils`. They had > 100 tests, and I
could only port like 12 as they are a bit time consuming given they
cannot be straight up copy pasted. Maybe we do not need all >100, but
maybe the more relevant to what we want.
- Refactor this code
Walk:
- Non fatal errors on `copy` from `utils`. Currently it just sends it to
stdout but errors have no span
- Better integration
An added possibility is the addition of `SyntaxShape::OneOf()` for
`Named` arguments which was briefly mentioned in the discord server, but
that is still to be decided. This could greatly improve some of the
integration. This would enable something like `cp --preserve [all
timestamp]` or `cp --preserve all` to both work.
I did not want to keep holding on this, and wait till I was happy with
the code because I think its nice if everyone can start up and suggest
refactors, but the main important part now was getting it out the door,
as if I take my sweet time this will take way longer :stuck_out_tongue:
<!--
Thank you for improving Nushell. Please, check our [contributing
guide](../CONTRIBUTING.md) and talk to the core team before making major
changes.
Description of your pull request goes here. **Provide examples and/or
screenshots** if your changes affect the user experience.
-->
# User-Facing Changes
<!-- List of all changes that impact the user experience here. This
helps us keep track of breaking changes. -->
# Tests + Formatting
Make sure you've run and fixed any issues with these commands:
- [X] cargo fmt --all -- --check` to check standard code formatting
(`cargo fmt --all` applies these changes)
- [X] cargo clippy --workspace -- -D warnings -D clippy::unwrap_used` to
check that you're using the standard code style
- [X] cargo test --workspace` to check that all tests pass
- [X] cargo run -- -c "use std testing; testing run-tests --path
crates/nu-std"` to run the tests for the standard library
> **Note**
> from `nushell` you can also use the `toolkit` as follows
> ```bash
> use toolkit.nu # or use an `env_change` hook to activate it
automatically
> toolkit check pr
> ```
-->
# After Submitting
<!-- If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
-->
---------
Co-authored-by: Darren Schroeder <343840+fdncred@users.noreply.github.com>
2023-09-08 20:57:38 +02:00
|
|
|
Cp,
|
|
|
|
UCp,
|
2021-12-24 20:24:55 +01:00
|
|
|
Open,
|
2023-01-03 19:47:37 +01:00
|
|
|
Start,
|
2021-12-11 04:07:39 +01:00
|
|
|
Rm,
|
2022-01-15 21:44:20 +01:00
|
|
|
Save,
|
2021-12-11 04:07:39 +01:00
|
|
|
Touch,
|
2022-04-04 22:45:01 +02:00
|
|
|
Glob,
|
2022-04-28 16:26:34 +02:00
|
|
|
Watch,
|
2021-12-11 04:07:39 +01:00
|
|
|
};
|
|
|
|
|
|
|
|
// Platform
|
|
|
|
bind_command! {
|
2021-12-17 18:40:47 +01:00
|
|
|
Ansi,
|
2021-12-17 21:32:03 +01:00
|
|
|
AnsiStrip,
|
2021-12-11 04:07:39 +01:00
|
|
|
Clear,
|
2022-06-15 20:11:26 +02:00
|
|
|
Du,
|
2022-01-16 05:28:28 +01:00
|
|
|
Input,
|
2023-05-04 19:14:41 +02:00
|
|
|
InputList,
|
2023-07-03 17:23:44 +02:00
|
|
|
InputListen,
|
2021-12-11 04:07:39 +01:00
|
|
|
Kill,
|
|
|
|
Sleep,
|
2022-01-21 04:31:33 +01:00
|
|
|
TermSize,
|
2021-12-11 04:07:39 +01:00
|
|
|
};
|
|
|
|
|
|
|
|
// Date
|
|
|
|
bind_command! {
|
|
|
|
Date,
|
|
|
|
DateHumanize,
|
|
|
|
DateListTimezones,
|
|
|
|
DateNow,
|
2022-04-01 10:09:30 +02:00
|
|
|
DateToRecord,
|
2021-12-11 04:07:39 +01:00
|
|
|
DateToTable,
|
|
|
|
DateToTimezone,
|
|
|
|
};
|
|
|
|
|
|
|
|
// Shells
|
|
|
|
bind_command! {
|
|
|
|
Exit,
|
|
|
|
};
|
|
|
|
|
|
|
|
// Formats
|
|
|
|
bind_command! {
|
|
|
|
From,
|
|
|
|
FromCsv,
|
|
|
|
FromJson,
|
2022-02-20 22:26:41 +01:00
|
|
|
FromNuon,
|
2021-12-11 04:07:39 +01:00
|
|
|
FromOds,
|
|
|
|
FromSsv,
|
|
|
|
FromToml,
|
|
|
|
FromTsv,
|
|
|
|
FromXlsx,
|
|
|
|
FromXml,
|
|
|
|
FromYaml,
|
|
|
|
FromYml,
|
2021-10-29 08:26:29 +02:00
|
|
|
To,
|
2021-12-03 03:02:22 +01:00
|
|
|
ToCsv,
|
2021-12-11 04:07:39 +01:00
|
|
|
ToJson,
|
2021-12-10 02:16:35 +01:00
|
|
|
ToMd,
|
2022-02-20 22:26:41 +01:00
|
|
|
ToNuon,
|
2022-05-04 21:12:23 +02:00
|
|
|
ToText,
|
2021-12-11 04:07:39 +01:00
|
|
|
ToToml,
|
|
|
|
ToTsv,
|
2021-12-11 21:08:17 +01:00
|
|
|
Touch,
|
2022-03-17 01:13:34 +01:00
|
|
|
Upsert,
|
2021-12-11 21:08:17 +01:00
|
|
|
Where,
|
2021-12-10 21:46:43 +01:00
|
|
|
ToXml,
|
2021-12-11 04:07:39 +01:00
|
|
|
ToYaml,
|
|
|
|
};
|
|
|
|
|
|
|
|
// Viewers
|
|
|
|
bind_command! {
|
|
|
|
Griddle,
|
|
|
|
Table,
|
|
|
|
};
|
|
|
|
|
|
|
|
// Conversions
|
|
|
|
bind_command! {
|
A `fill` command to replace `str lpad` and `str rpad` (#7846)
# Description
The point of this command is to allow you to be able to format ints,
floats, filesizes, and strings with an alignment, padding, and a fill
character, as strings. It's meant to take the place of `str lpad` and
`str rpad`.
```
> help fill
Fill and Align
Search terms: display, render, format, pad, align
Usage:
> fill {flags}
Flags:
-h, --help - Display the help message for this command
-w, --width <Int> - The width of the output. Defaults to 1
-a, --alignment <String> - The alignment of the output. Defaults to Left (Left(l), Right(r), Center(c/m), MiddleRight(cr/mr))
-c, --character <String> - The character to fill with. Defaults to ' ' (space)
Signatures:
<number> | fill -> <string>
<string> | fill -> <string>
Examples:
Fill a string on the left side to a width of 15 with the character '─'
> 'nushell' | fill -a l -c '─' -w 15
Fill a string on the right side to a width of 15 with the character '─'
> 'nushell' | fill -a r -c '─' -w 15
Fill a string on both sides to a width of 15 with the character '─'
> 'nushell' | fill -a m -c '─' -w 15
Fill a number on the left side to a width of 5 with the character '0'
> 1 | fill --alignment right --character 0 --width 5
Fill a filesize on the left side to a width of 5 with the character '0'
> 1kib | fill --alignment middle --character 0 --width 10
```
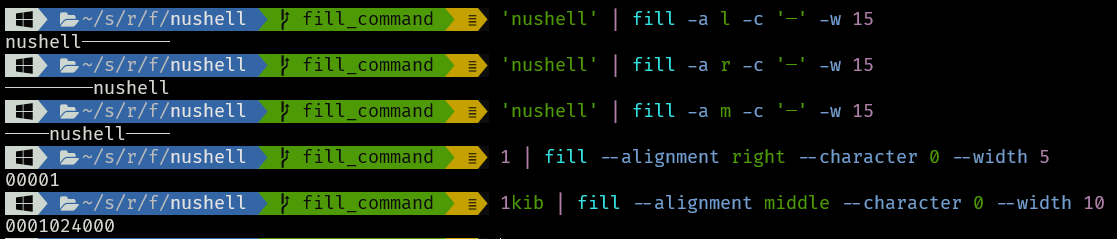
# User-Facing Changes
Deprecated `str lpad` and `str rpad`.
# Tests + Formatting
Don't forget to add tests that cover your changes.
Make sure you've run and fixed any issues with these commands:
- `cargo fmt --all -- --check` to check standard code formatting (`cargo
fmt --all` applies these changes)
- `cargo clippy --workspace -- -D warnings -D clippy::unwrap_used -A
clippy::needless_collect` to check that you're using the standard code
style
- `cargo test --workspace` to check that all tests pass
# After Submitting
If your PR had any user-facing changes, update [the
documentation](https://github.com/nushell/nushell.github.io) after the
PR is merged, if necessary. This will help us keep the docs up to date.
2023-02-09 21:56:52 +01:00
|
|
|
Fill,
|
2021-12-11 04:07:39 +01:00
|
|
|
Into,
|
2021-12-19 21:11:28 +01:00
|
|
|
IntoBool,
|
2021-12-11 04:07:39 +01:00
|
|
|
IntoBinary,
|
|
|
|
IntoDatetime,
|
|
|
|
IntoDecimal,
|
2022-03-03 14:16:04 +01:00
|
|
|
IntoDuration,
|
2023-09-12 13:02:47 +02:00
|
|
|
IntoFloat,
|
2021-12-11 04:07:39 +01:00
|
|
|
IntoFilesize,
|
|
|
|
IntoInt,
|
2022-11-26 16:00:47 +01:00
|
|
|
IntoRecord,
|
2021-12-11 04:07:39 +01:00
|
|
|
IntoString,
|
|
|
|
};
|
|
|
|
|
|
|
|
// Env
|
|
|
|
bind_command! {
|
2022-08-18 22:24:39 +02:00
|
|
|
ExportEnv,
|
2022-01-16 00:50:11 +01:00
|
|
|
LoadEnv,
|
2022-08-31 22:32:56 +02:00
|
|
|
SourceEnv,
|
2021-11-04 03:32:35 +01:00
|
|
|
WithEnv,
|
2022-05-22 05:13:58 +02:00
|
|
|
ConfigNu,
|
|
|
|
ConfigEnv,
|
2022-05-22 19:31:57 +02:00
|
|
|
ConfigMeta,
|
2022-08-01 03:44:33 +02:00
|
|
|
ConfigReset,
|
2021-12-11 04:07:39 +01:00
|
|
|
};
|
|
|
|
|
|
|
|
// Math
|
|
|
|
bind_command! {
|
|
|
|
Math,
|
|
|
|
MathAbs,
|
|
|
|
MathAvg,
|
|
|
|
MathCeil,
|
|
|
|
MathFloor,
|
|
|
|
MathMax,
|
|
|
|
MathMedian,
|
|
|
|
MathMin,
|
|
|
|
MathMode,
|
|
|
|
MathProduct,
|
|
|
|
MathRound,
|
|
|
|
MathSqrt,
|
|
|
|
MathStddev,
|
|
|
|
MathSum,
|
|
|
|
MathVariance,
|
2022-12-09 11:20:42 +01:00
|
|
|
MathLog,
|
2021-12-11 04:07:39 +01:00
|
|
|
};
|
|
|
|
|
2023-08-19 22:43:53 +02:00
|
|
|
// Bytes
|
|
|
|
bind_command! {
|
|
|
|
Bytes,
|
|
|
|
BytesLen,
|
|
|
|
BytesStartsWith,
|
|
|
|
BytesEndsWith,
|
|
|
|
BytesReverse,
|
|
|
|
BytesReplace,
|
|
|
|
BytesAdd,
|
|
|
|
BytesAt,
|
|
|
|
BytesIndexOf,
|
|
|
|
BytesCollect,
|
|
|
|
BytesRemove,
|
|
|
|
BytesBuild
|
|
|
|
}
|
|
|
|
|
2021-12-12 20:42:04 +01:00
|
|
|
// Network
|
|
|
|
bind_command! {
|
2023-01-20 19:38:30 +01:00
|
|
|
Http,
|
2023-02-24 00:52:12 +01:00
|
|
|
HttpDelete,
|
2023-01-20 19:38:30 +01:00
|
|
|
HttpGet,
|
2023-02-24 00:52:12 +01:00
|
|
|
HttpHead,
|
|
|
|
HttpPatch,
|
2023-01-20 19:38:30 +01:00
|
|
|
HttpPost,
|
2023-02-24 00:52:12 +01:00
|
|
|
HttpPut,
|
2023-06-09 15:00:40 +02:00
|
|
|
HttpOptions,
|
2021-12-12 20:42:04 +01:00
|
|
|
Url,
|
2023-01-15 19:16:29 +01:00
|
|
|
UrlBuildQuery,
|
2023-01-05 19:24:38 +01:00
|
|
|
UrlEncode,
|
Feat/7725 url join (#7823)
# Description
Added command: `url join`.
Closes: #7725
# User-Facing Changes
```
Converts a record to url
Search terms: scheme, username, password, hostname, port, path, query, fragment
Usage:
> url join
Flags:
-h, --help - Display the help message for this command
Signatures:
<record> | url join -> <string>
Examples:
Outputs a url representing the contents of this record
> {
"scheme": "http",
"username": "",
"password": "",
"host": "www.pixiv.net",
"port": "",
"path": "/member_illust.php",
"query": "mode=medium&illust_id=99260204",
"fragment": "",
"params":
{
"mode": "medium",
"illust_id": "99260204"
}
} | url join
Outputs a url representing the contents of this record
> {
"scheme": "http",
"username": "user",
"password": "pwd",
"host": "www.pixiv.net",
"port": "1234",
"query": "test=a",
"fragment": ""
} | url join
Outputs a url representing the contents of this record
> {
"scheme": "http",
"host": "www.pixiv.net",
"port": "1234",
"path": "user",
"fragment": "frag"
} | url join
```
# Questions
- Which parameters should be required? Currenlty are: `scheme` and
`host`.
2023-01-22 19:49:40 +01:00
|
|
|
UrlJoin,
|
2022-11-19 19:14:29 +01:00
|
|
|
UrlParse,
|
2022-06-22 05:27:58 +02:00
|
|
|
Port,
|
2021-12-12 20:42:04 +01:00
|
|
|
}
|
|
|
|
|
2021-12-11 04:07:39 +01:00
|
|
|
// Random
|
|
|
|
bind_command! {
|
|
|
|
Random,
|
2021-12-12 20:42:04 +01:00
|
|
|
RandomBool,
|
|
|
|
RandomChars,
|
|
|
|
RandomDecimal,
|
|
|
|
RandomDice,
|
|
|
|
RandomInteger,
|
|
|
|
RandomUuid,
|
2021-12-11 04:07:39 +01:00
|
|
|
};
|
|
|
|
|
|
|
|
// Generators
|
|
|
|
bind_command! {
|
|
|
|
Cal,
|
2022-01-16 14:52:41 +01:00
|
|
|
Seq,
|
2022-01-14 23:07:28 +01:00
|
|
|
SeqDate,
|
2022-05-06 17:40:02 +02:00
|
|
|
SeqChar,
|
2021-12-11 04:07:39 +01:00
|
|
|
};
|
|
|
|
|
|
|
|
// Hash
|
|
|
|
bind_command! {
|
2021-12-11 00:14:28 +01:00
|
|
|
Hash,
|
|
|
|
HashMd5::default(),
|
|
|
|
HashSha256::default(),
|
2021-12-11 04:07:39 +01:00
|
|
|
};
|
2021-09-03 04:15:01 +02:00
|
|
|
|
2022-01-30 23:05:25 +01:00
|
|
|
// Experimental
|
|
|
|
bind_command! {
|
2022-06-06 13:55:23 +02:00
|
|
|
IsAdmin,
|
2022-01-30 23:05:25 +01:00
|
|
|
};
|
|
|
|
|
2023-08-14 21:17:31 +02:00
|
|
|
// Removed
|
2022-02-10 13:55:19 +01:00
|
|
|
bind_command! {
|
2023-08-06 13:42:16 +02:00
|
|
|
LetEnv,
|
|
|
|
DateFormat,
|
2022-02-10 13:55:19 +01:00
|
|
|
};
|
|
|
|
|
2021-09-03 00:58:15 +02:00
|
|
|
working_set.render()
|
|
|
|
};
|
|
|
|
|
2022-07-14 16:09:27 +02:00
|
|
|
if let Err(err) = engine_state.merge_delta(delta) {
|
2023-01-30 02:37:54 +01:00
|
|
|
eprintln!("Error creating default context: {err:?}");
|
2022-07-14 16:09:27 +02:00
|
|
|
}
|
2021-09-03 00:58:15 +02:00
|
|
|
|
2023-03-25 18:12:57 +01:00
|
|
|
// Cache the table decl id so we don't have to look it up later
|
|
|
|
let table_decl_id = engine_state.find_decl("table".as_bytes(), &[]);
|
|
|
|
engine_state.table_decl_id = table_decl_id;
|
|
|
|
|
2021-09-03 00:58:15 +02:00
|
|
|
engine_state
|
2021-01-01 03:12:16 +01:00
|
|
|
}
|